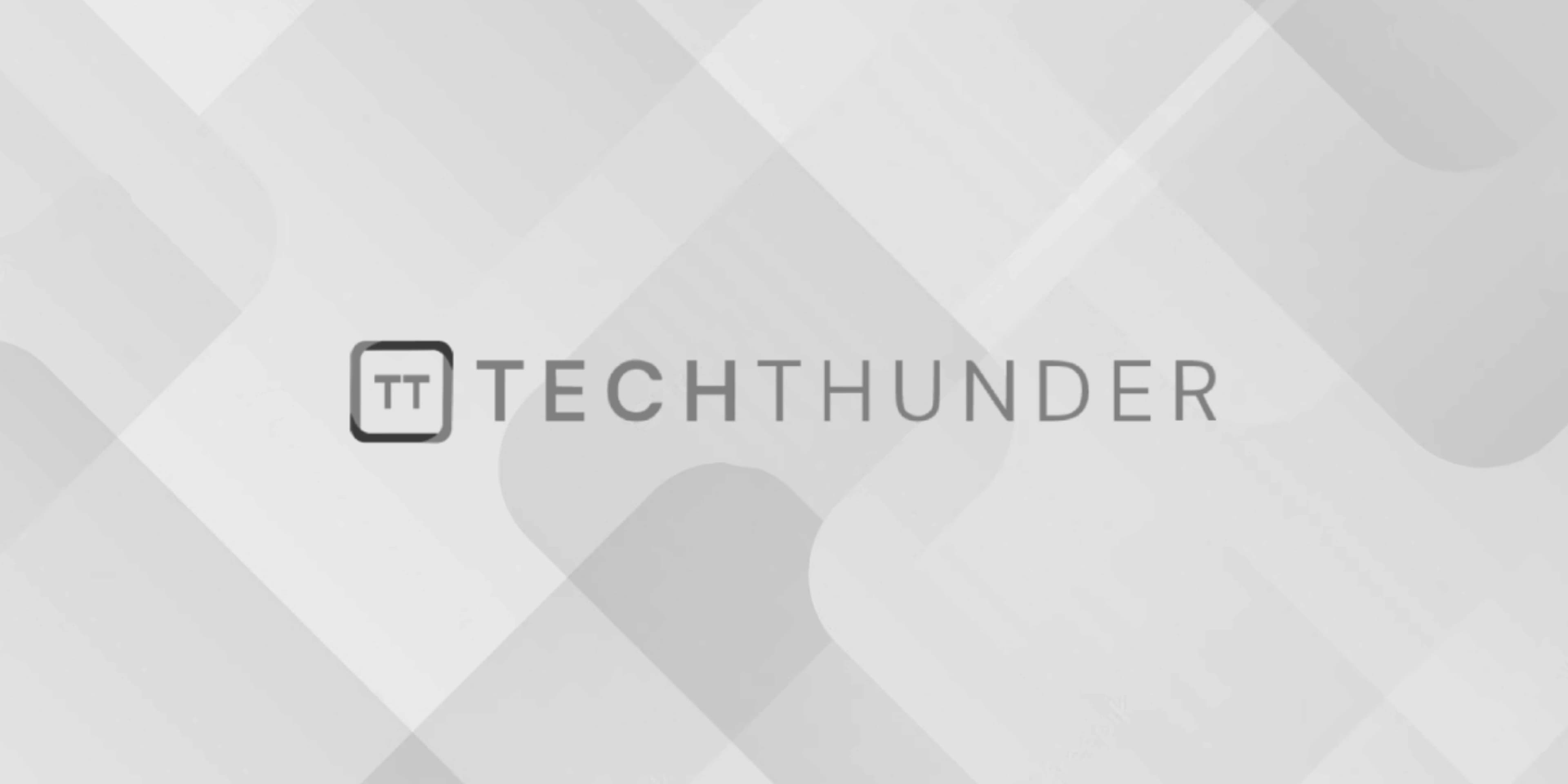
JavaScript Debouncing
JavaScript debouncing is a technique used to control the frequency of a function call, particularly in scenarios where the function is invoked multiple times within a short time period (such as user input events like scrolling, resizing, or typing). Debouncing helps optimize performance by delaying the execution of the function until a certain amount of time has passed since the last invocation.
Here’s an example implementation of debouncing in JavaScript:
function debounce(func, delay) {
let timerId;
return function(...args) {
clearTimeout(timerId);
timerId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
In this example, the debounce
function takes two arguments: func
, which is the function to be debounced, and delay
, which specifies the time in milliseconds to wait before invoking the function.
The debounce
function returns a new function that wraps the original function. When this wrapper function is called, it clears any previously set timeout using clearTimeout
and sets a new timeout using setTimeout
. The wrapped function is invoked after the specified delay, ensuring that the original function is executed only after a certain period of inactivity.
Here’s an example of how to use the debounce
function:
function handleScroll() {
console.log("Scrolling...");
}
// Debounce the handleScroll function with a delay of 200 milliseconds
const debouncedScroll = debounce(handleScroll, 200);
// Attach the debounced function to the scroll event
window.addEventListener("scroll", debouncedScroll);
In this example, the handleScroll
function is debounced with a delay of 200 milliseconds. The debounced version, debouncedScroll
, is then attached to the scroll event. The handleScroll
function will be called only after a 200 millisecond period of inactivity since the last scroll event. This helps optimize the performance by reducing the number of function invocations during rapid scrolling.