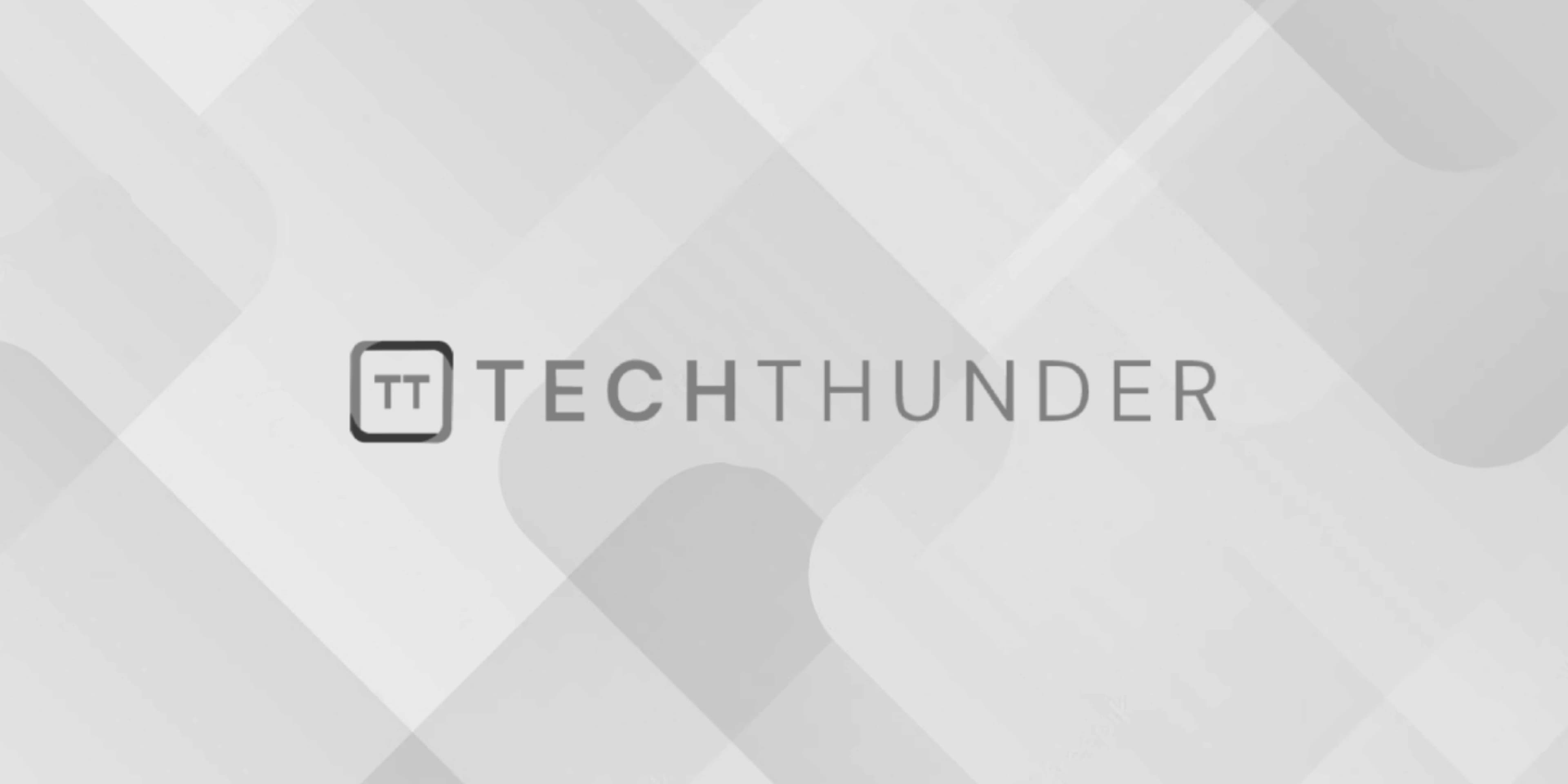
414 views
JavaScript Loops
The javascript loops are used to execute a block of code repeatedly until a certain condition is met. There are three types of loops in JavaScript: for
, while
, and do-while
.
1. for
loop: The for
loop is used when we know how many times we want to execute the loop. It consists of three parts: the initialization, the condition, and the increment/decrement.
JavaScript
for (let i = 0; i < 10; i++) {
console.log(i);
}
2. while
loop: The while
loop is used when we don’t know how many times we want to execute the loop. It executes the code block as long as the condition is true.
JavaScript
let i = 0;
while (i < 10) {
console.log(i);
i++;
}
3. do-while
loop: The do-while
loop is similar to the while
loop, but it always executes the code block at least once before checking the condition.
JavaScript
let i = 0;
do {
console.log(i);
i++;
} while (i < 10);