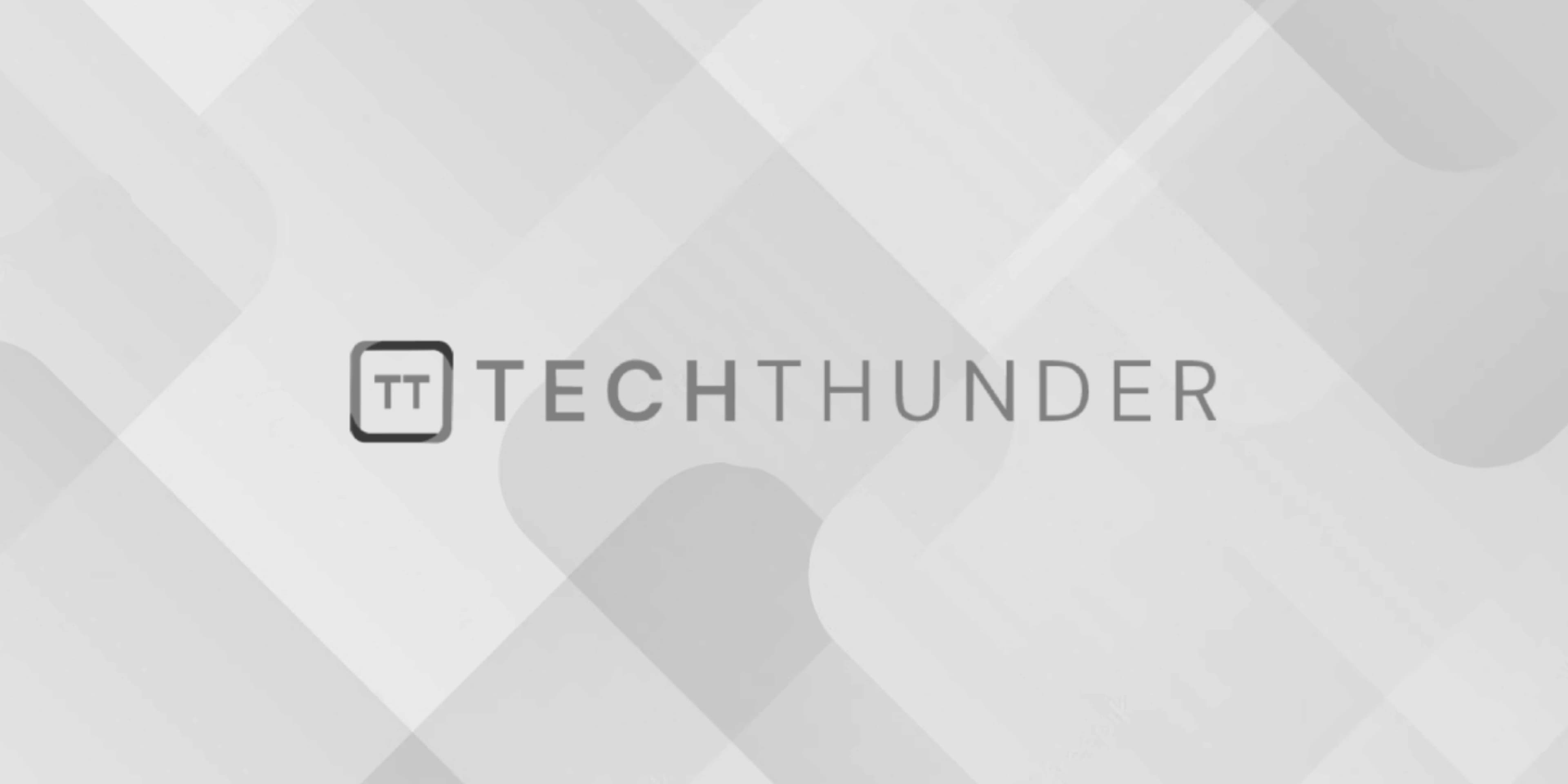
Javascript Setinterval
The setInterval()
function in JavaScript is used to repeatedly execute a given function or code snippet at a fixed interval. It takes two parameters: the function to be executed and the time interval (in milliseconds) between each execution.
Here’s an example of how to use setInterval()
:
// Example function to be executed
function doSomething() {
console.log("Doing something...");
}
// Execute the function every 1 second (1000 milliseconds)
var intervalID = setInterval(doSomething, 1000);
In this example, the doSomething()
function will be executed every 1 second. The setInterval()
function returns an interval ID, which can be used to stop the interval using the clearInterval()
function:
// Clear the interval after 5 seconds
setTimeout(function() {
clearInterval(intervalID);
}, 5000);
In this case, the clearInterval()
function is called after 5 seconds using setTimeout()
, which stops the execution of the setInterval()
function.
It’s important to note that setInterval()
will continue executing the provided function indefinitely until it is explicitly stopped using clearInterval()
.