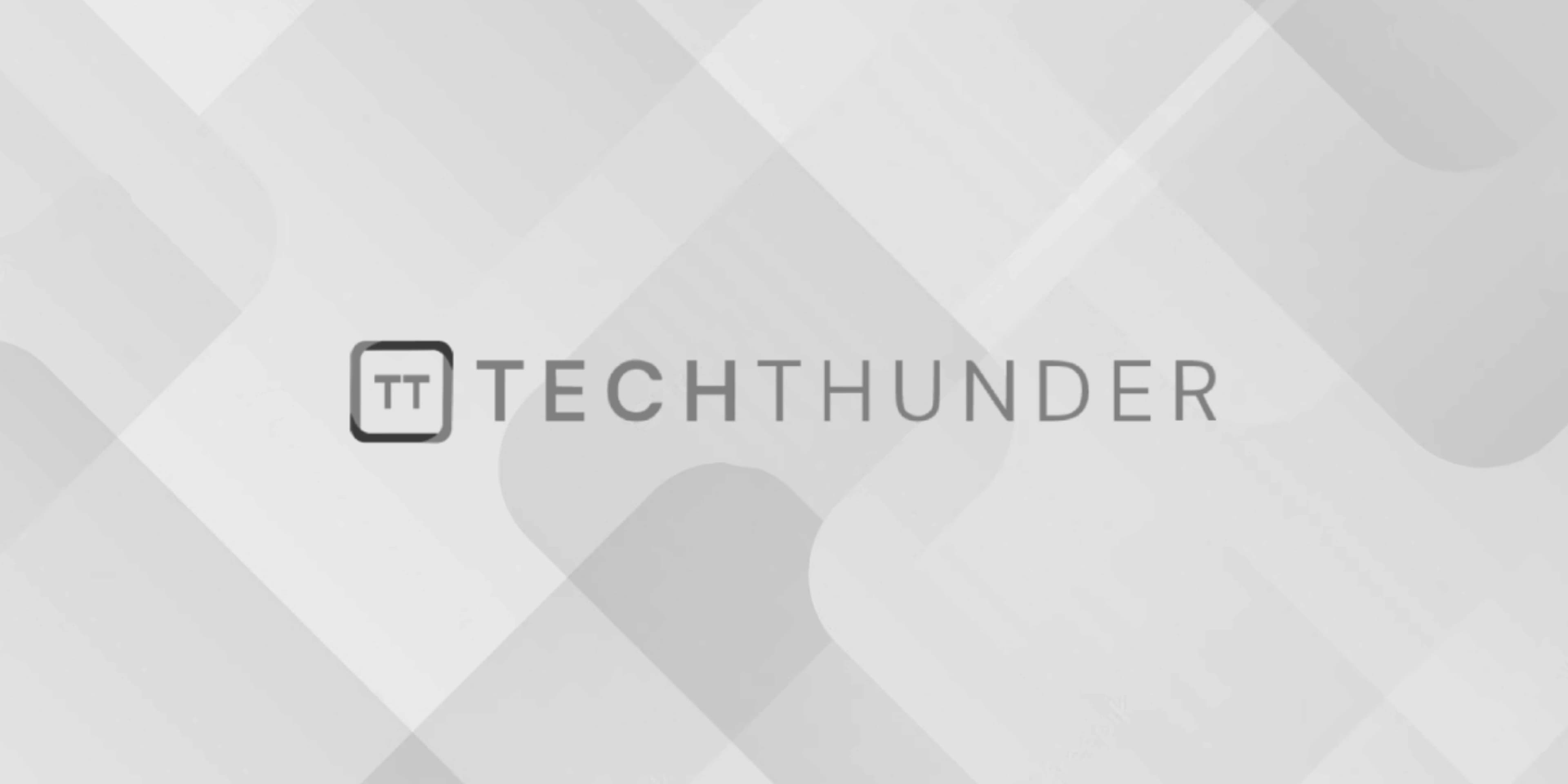
JavaScript querySelector
The JavaScript querySelector
method is used to select and retrieve the first element that matches a specified CSS selector. It is a method available on the document
object and can also be called on individual elements to search within their descendants. Here are some examples:
- Selecting an element by ID:
var element = document.querySelector("#myElement");
In this example, the querySelector
method selects the first element with the ID "myElement"
.
- Selecting an element by class:
var element = document.querySelector(".myClass");
Here, the querySelector
method selects the first element with the class "myClass"
.
- Selecting an element by tag name:
var element = document.querySelector("div");
This example selects the first <div>
element in the document.
- Selecting an element within another element:
var parentElement = document.querySelector("#parentElement");
var childElement = parentElement.querySelector(".childElement");
In this case, querySelector
is called on parentElement
to select the first element with the class "childElement"
within it.
- Selecting multiple elements:
var elements = document.querySelectorAll(".myClass");
The querySelectorAll
method is used to select all elements that match the specified CSS selector. It returns a NodeList
containing all the matched elements.
Note that querySelector
and querySelectorAll
support a wide range of CSS selectors, allowing you to select elements based on various criteria like class, ID, attribute, hierarchy, etc.
When using querySelector
, if no element matches the specified selector, it returns null
. When using querySelectorAll
, if no elements match the selector, it returns an empty NodeList
.
These examples demonstrate how to use querySelector
to select elements in different ways. Once you have selected an element, you can manipulate it or access its properties and content using JavaScript.