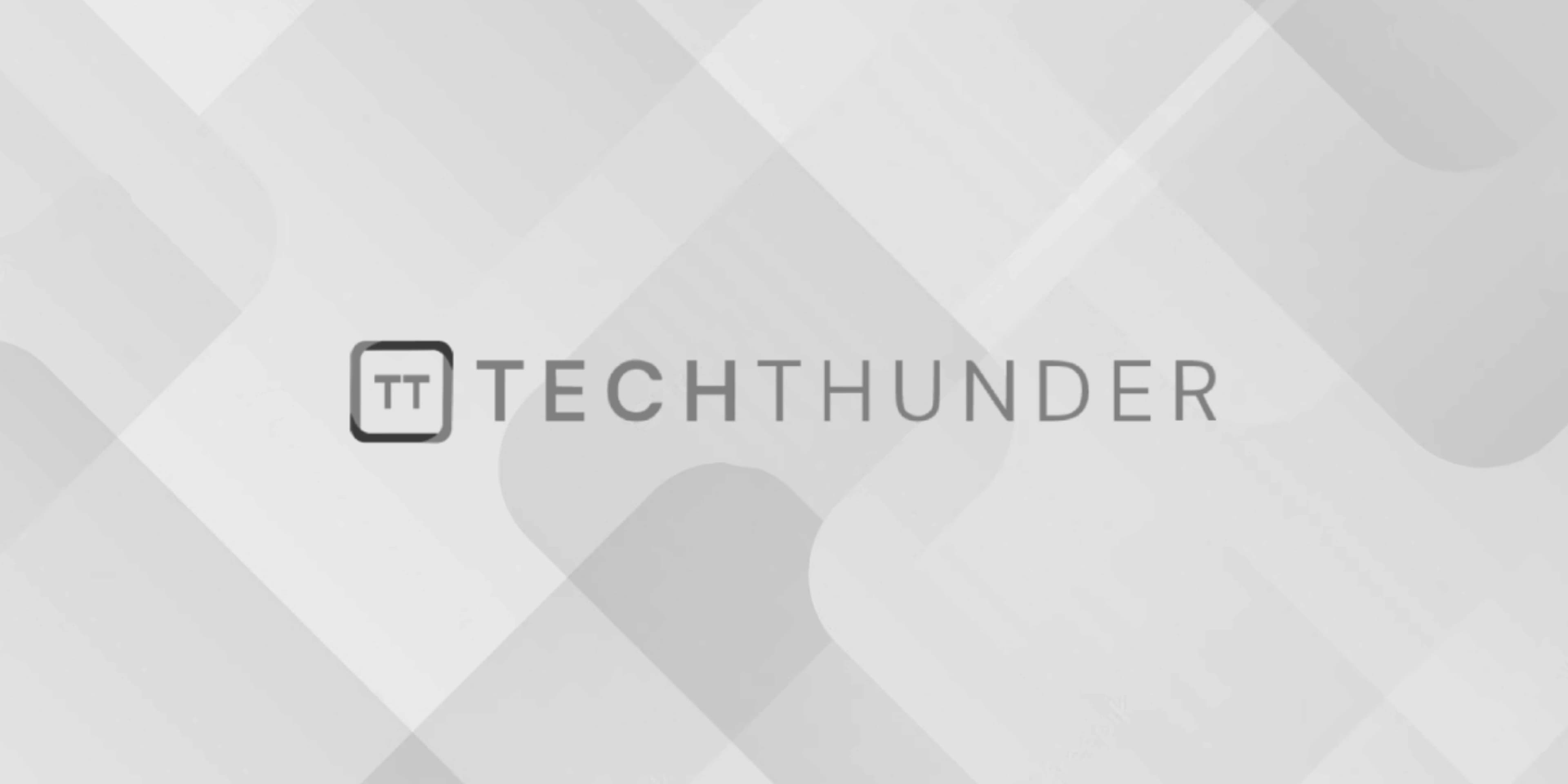
96 views
JavaScript String startsWith
The startsWith()
method is a built-in method in JavaScript that is used to determine whether a string starts with a specified substring. It returns true
if the string starts with the specified substring, and false
otherwise.
Here’s the syntax of the startsWith()
method:
JavaScript
str.startsWith(searchString[, position])
searchString
: The substring to search for at the start of the string.position
(optional): The position in the string at which to start the search. Defaults to0
.
Here’s an example usage of the startsWith()
method:
JavaScript
const str = 'Hello, world!';
console.log(str.startsWith('Hello')); // Output: true
console.log(str.startsWith('hello')); // Output: false
console.log(str.startsWith('world', 7)); // Output: true
In this example, we have a string str
that contains the text “Hello, world!”. We use the startsWith()
method to check if the string starts with different substrings.
- The first
startsWith('Hello')
call returnstrue
because the stringstr
starts with the substring “Hello”. - The second
startsWith('hello')
call returnsfalse
because thestartsWith()
method performs a case-sensitive comparison, and the stringstr
does not start with the substring “hello” (note the lowercase ‘h’). - The third
startsWith('world', 7)
call returnstrue
because the search starts at index 7, and the remaining part of the string starting from that index is “world”.
The startsWith()
method is particularly useful when you need to perform string prefix checks or pattern matching in JavaScript.