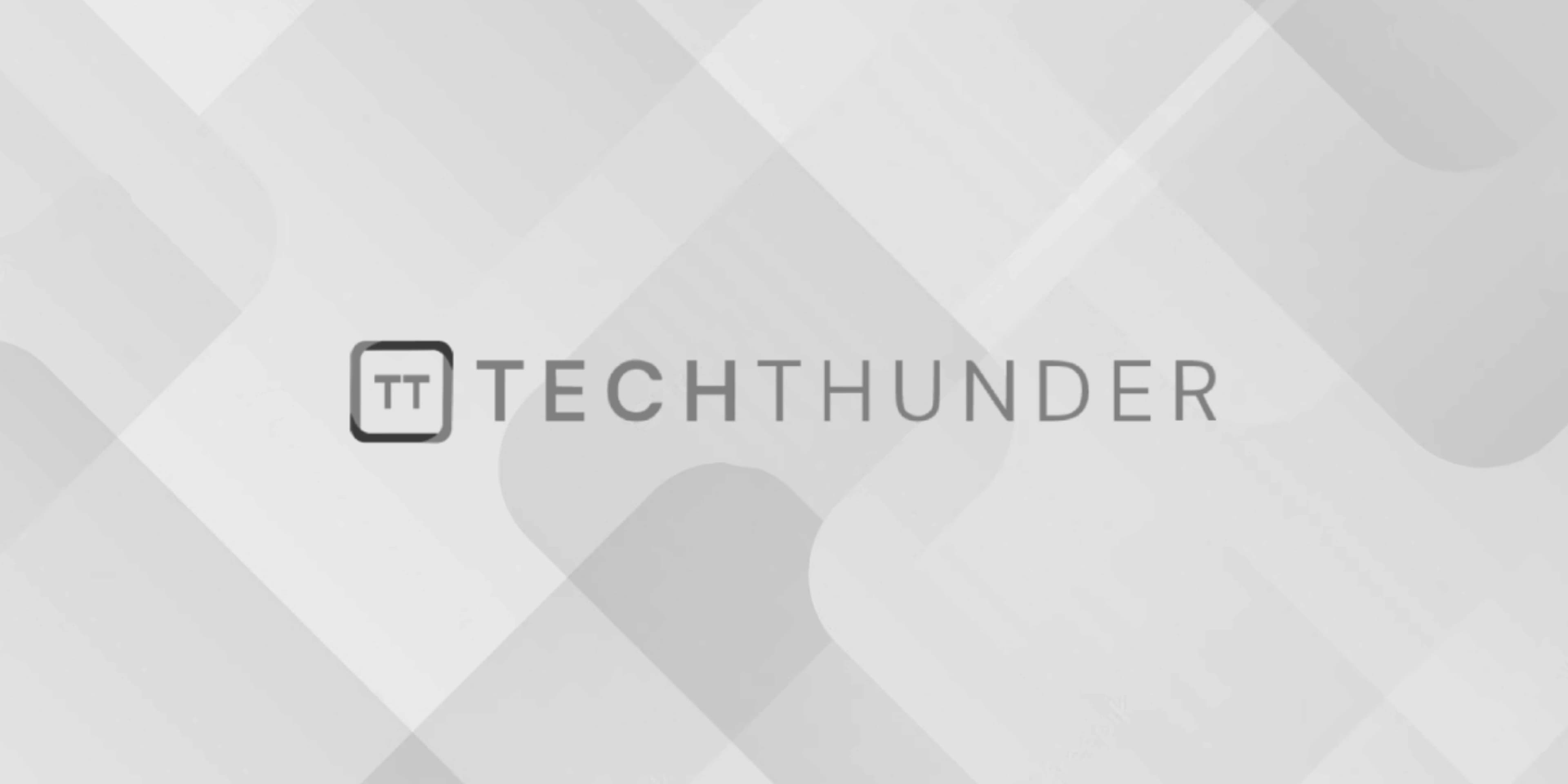
How to check two array values are equal in JavaScript
To check if two arrays have equal values in JavaScript, you can use the every()
method along with the includes()
or indexOf()
method. Here are two approaches:
- Using
every()
andincludes()
:
function arraysEqual(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
return arr1.every((value) => arr2.includes(value));
}
In this example, the arraysEqual()
function takes two arrays (arr1
and arr2
) as parameters. It first checks if the lengths of the two arrays are equal. If not, it returns false
since arrays with different lengths cannot have equal values.
Then, it uses the every()
method to iterate over each element of arr1
and checks if that element is present in arr2
using the includes()
method. If all elements of arr1
are found in arr2
, it returns true
; otherwise, it returns false
.
- Using
every()
andindexOf()
:
function arraysEqual(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
return arr1.every((value) => arr2.indexOf(value) !== -1);
}
This approach is similar to the previous one, but instead of using includes()
, it uses the indexOf()
method to check if an element exists in arr2
. If the indexOf()
method returns -1
, it means the element is not found in arr2
.
Here’s an example of using these functions:
const array1 = [1, 2, 3, 4];
const array2 = [1, 2, 3, 4];
const array3 = [1, 2, 3, 5];
console.log(arraysEqual(array1, array2)); // Output: true
console.log(arraysEqual(array1, array3)); // Output: false
In this example, array1
and array2
have the same values, so arraysEqual()
returns true
. However, array1
and array3
differ in value at the last index, so arraysEqual()
returns false
.
By using these approaches, you can compare the values of two arrays in JavaScript and determine if they are equal.