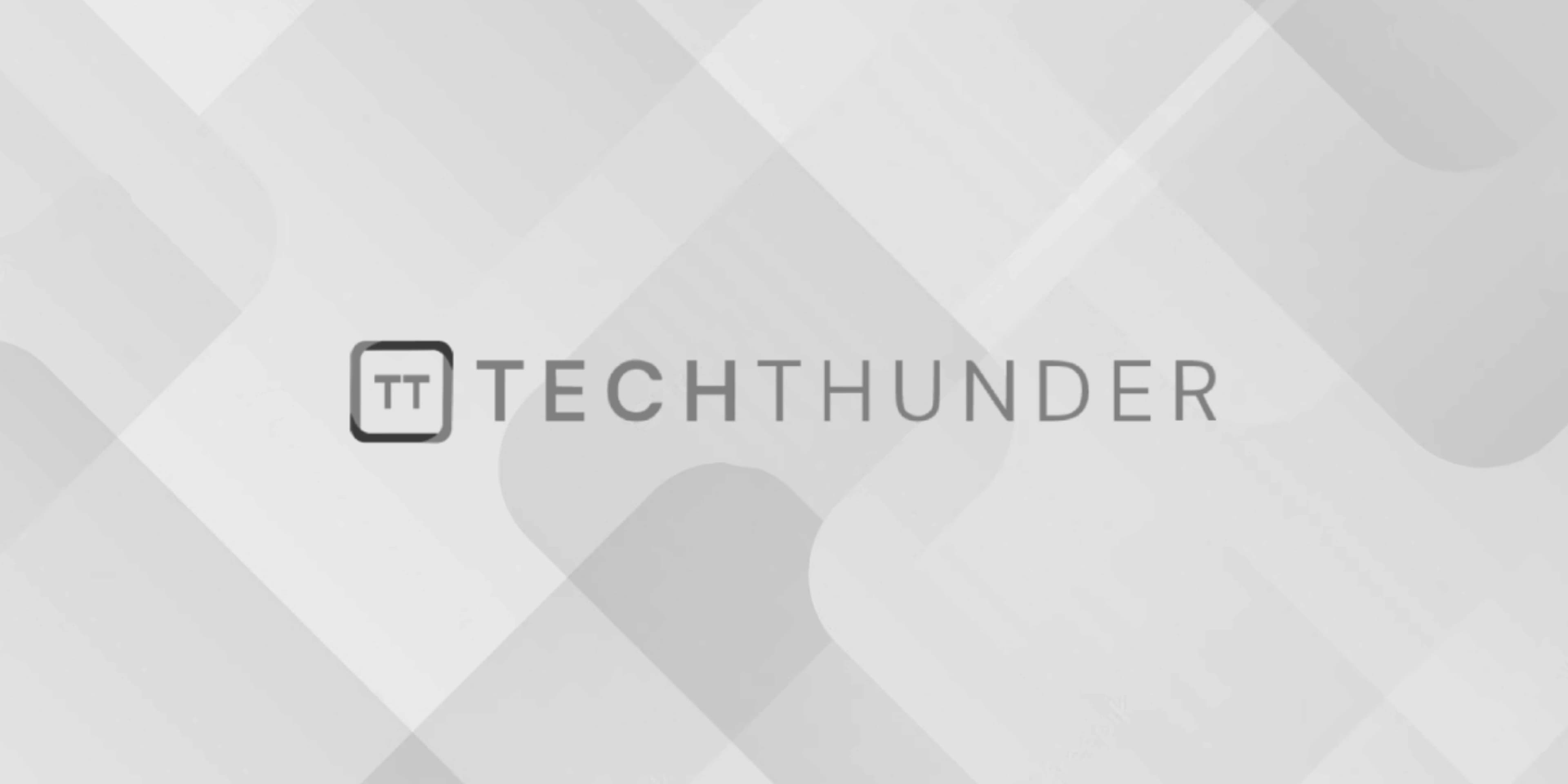
JavaScript Functions
The javascript function is a block of reusable code that performs a specific task. Functions are used to break down large programs into smaller, manageable and modular components.
To create a function in JavaScript, the function
keyword is used, followed by the name of the function, a set of parentheses that may contain parameters, and a set of curly braces that contain the code to be executed by the function.
Here’s an example of a simple JavaScript function that takes two parameters and returns their sum:
function addNumbers(num1, num2) {
return num1 + num2;
}
Functions can also be defined using function expressions, which involve assigning a function to a variable. Here’s an example:
const multiplyNumbers = function(num1, num2) {
return num1 * num2;
};
Functions can be called (or executed) by using the function name followed by a set of parentheses containing any arguments to be passed to the function. Here’s an example of calling the addNumbers
function defined above:
let sum = addNumbers(3, 5);
console.log(sum); // Output: 8
Functions can also be nested within other functions, and can be passed as arguments to other functions.