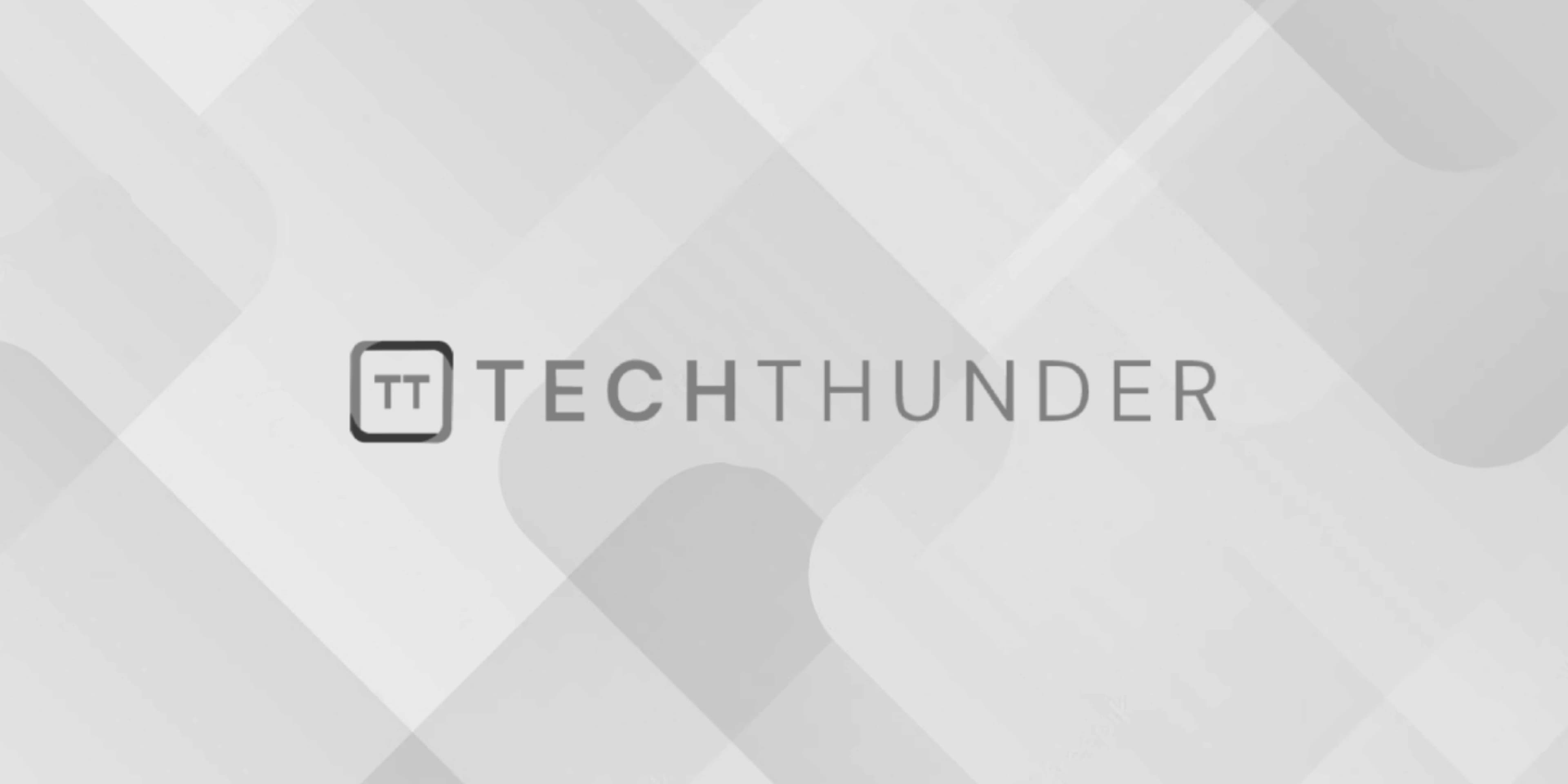
JavaScript Number
The JavaScript number is a primitive data type that represents numeric values. JavaScript supports both integer and floating-point numbers.
Here are some examples of numeric values in JavaScript:
let num1 = 42; // integer number
let num2 = 3.14; // floating-point number
let num3 = 0.000001; // small floating-point number
let num4 = -10; // negative integer number
JavaScript provides several built-in methods for working with numbers, such as toFixed()
, toPrecision()
, and toString()
. These methods can be used to format numbers and convert them to strings, among other things.
let num = 3.14159;
console.log(num.toFixed(2)); // Output: 3.14 (formats the number to 2 decimal places)
console.log(num.toPrecision(3)); // Output: 3.14 (formats the number to 3 significant digits)
console.log(num.toString()); // Output: "3.14159" (converts the number to a string)
JavaScript also provides a number of built-in mathematical functions, such as Math.sqrt()
, Math.pow()
, and Math.round()
. These functions can be used to perform mathematical operations on numbers.
console.log(Math.sqrt(16)); // Output: 4 (calculates the square root of 16)
console.log(Math.pow(2, 3)); // Output: 8 (calculates 2 to the power of 3)
console.log(Math.round(3.14)); // Output: 3 (rounds the number to the nearest integer)
It’s worth noting that JavaScript numbers have a limited precision and range. For example, the largest number that can be represented in JavaScript is Number.MAX_VALUE
, which is approximately 1.79 x 10^308. Numbers that exceed this limit will be represented as Infinity
or -Infinity
.