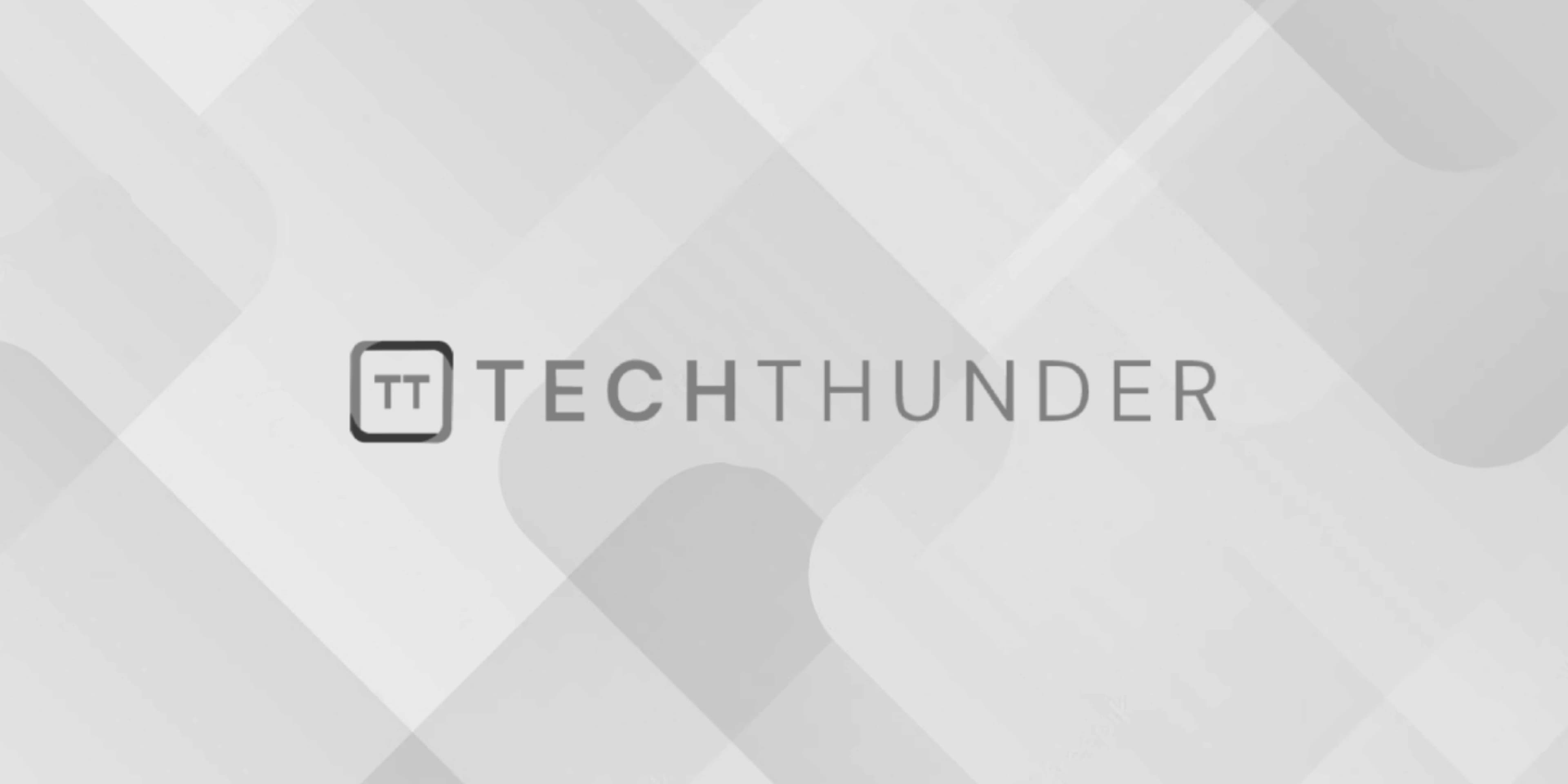
What is hoisting in JavaScript
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their respective scopes during the compilation phase, regardless of where they are actually written in the code. This means that you can use variables and functions before they are declared in the code.
However, it’s important to note that only the declarations are hoisted, not the initializations or assignments. In other words, hoisting moves the declaration to the top but leaves the assignment in place.
Here’s an example to illustrate hoisting with variable and function declarations:
console.log(myVariable); // undefined
var myVariable = 10;
hoistedFunction(); // "Hello, I am a hoisted function."
function hoistedFunction() {
console.log("Hello, I am a hoisted function.");
}
In this example, even though the myVariable
declaration appears after the console.log
statement, the variable is still accessible but has the value of undefined
until it is assigned a value later.
Similarly, the hoistedFunction
is called before its actual declaration in the code, but it still works correctly because function declarations are hoisted to the top.
It’s worth mentioning that hoisting affects only declarations, not variable assignments or function expressions. To avoid confusion and potential issues, it is generally recommended to declare variables and functions before using them in the code, even though hoisting allows them to be used beforehand.