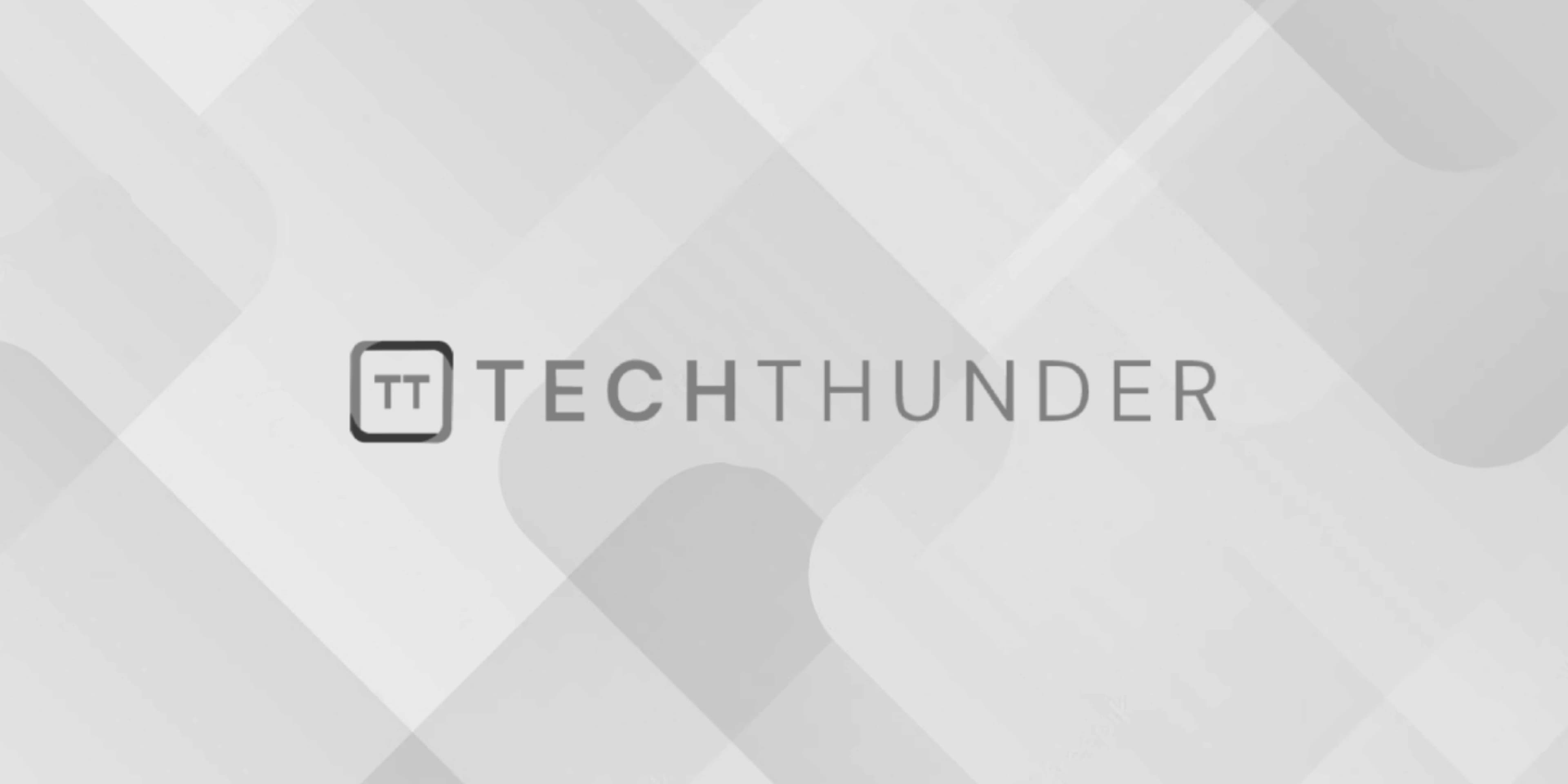
JavaScript Form
To work with forms in JavaScript, you can access and manipulate form elements and handle form submissions. Here’s an example of how you can interact with a form using JavaScript:
HTML form structure:
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Submit">
</form>
JavaScript code to handle form submission:
// Access the form element
const form = document.getElementById("myForm");
// Add a submit event listener to the form
form.addEventListener("submit", function(event) {
event.preventDefault(); // Prevent the default form submission behavior
// Access the form input values
const nameInput = document.getElementById("name");
const emailInput = document.getElementById("email");
// Retrieve the values entered by the user
const name = nameInput.value;
const email = emailInput.value;
// Perform validation or further processing
if (name && email) {
// Form is valid, perform desired actions
console.log("Name:", name);
console.log("Email:", email);
// Reset the form
form.reset();
} else {
// Display an error message or handle invalid form submission
console.log("Please enter valid values for name and email.");
}
});
In the above example, we first access the form element using its id
attribute. Then, we add a submit event listener to the form using the addEventListener()
method. Inside the event listener, we prevent the default form submission behavior using event.preventDefault()
.
Next, we access the individual form input elements using their id
attributes. We retrieve the entered values using the value
property of each input element.
After retrieving the form values, you can perform validation or further processing based on your requirements. In this example, we check if both the name and email values are provided. If they are valid, we perform desired actions (in this case, printing the values to the console and resetting the form). Otherwise, we display an error message.
Remember to wrap your JavaScript code in a script tag or include it in an external JavaScript file and place it after the form element in your HTML document.