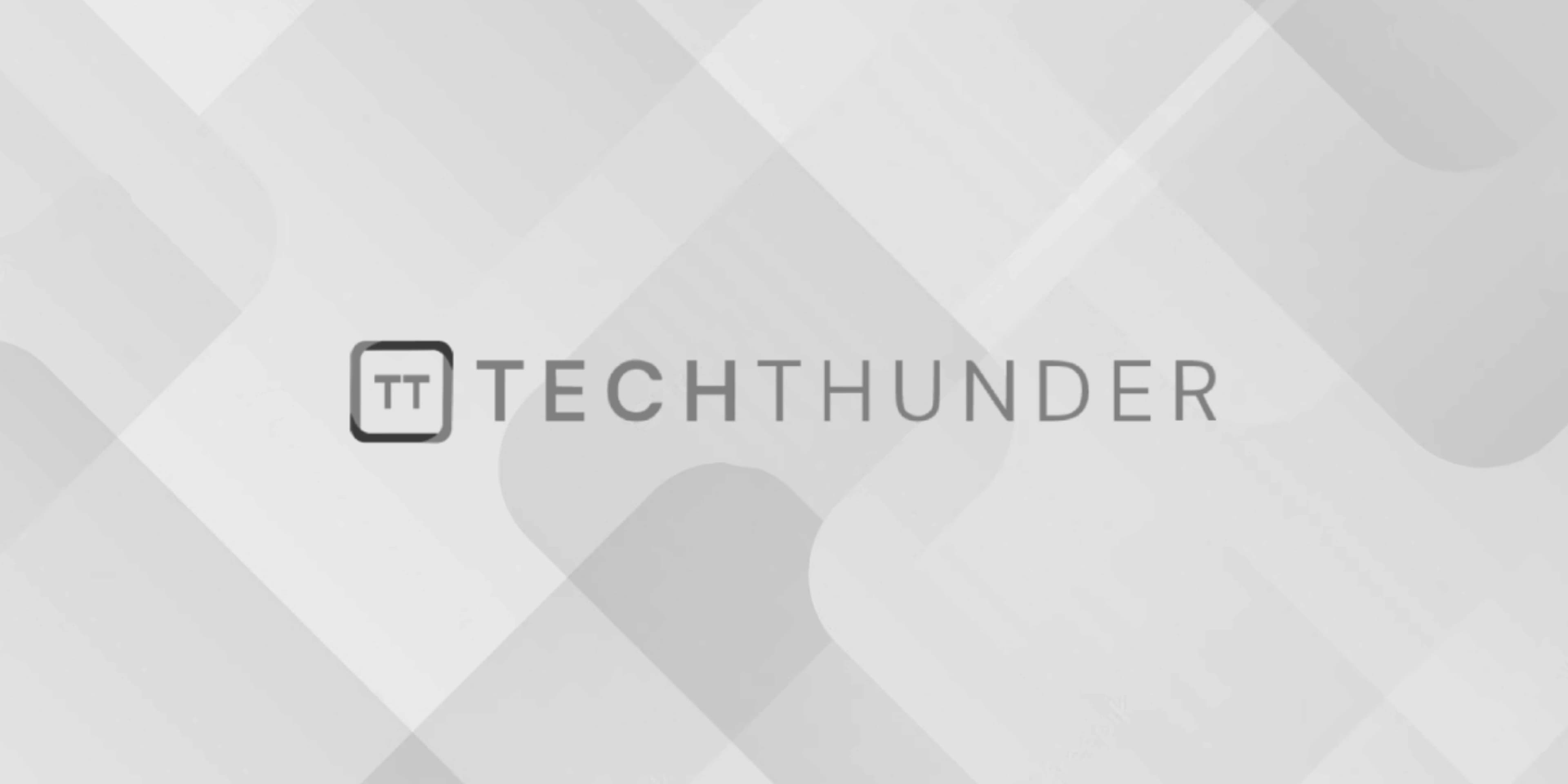
240 views
JavaScript Inheritance
JavaScript supports prototype-based inheritance, where objects can inherit properties and methods from other objects. Inheritance allows you to create a hierarchy of objects, where a child object inherits the characteristics of its parent object.
There are different ways to implement inheritance in JavaScript:
- Prototype Chain: JavaScript objects have a built-in property called
prototype
, which allows objects to inherit properties and methods from other objects. When a property or method is accessed on an object, JavaScript looks for it first on the object itself, and if not found, it searches up the prototype chain until it finds the property or reaches the top-level object, usuallyObject.prototype
. To create an inheritance relationship between objects, you can use theObject.create()
method to create a new object that inherits from another object’s prototype. By setting the prototype of a child object to be an instance of the parent object, the child object can inherit the parent’s properties and methods. - Constructor Functions: Another way to implement inheritance in JavaScript is through constructor functions and the
new
keyword. Constructor functions can be used to create objects with shared properties and methods. By using theprototype
property of constructor functions, you can define shared properties and methods that are inherited by objects created with the constructor. To establish inheritance between constructor functions, you can use theprototype
property of the child constructor function to link it to an instance of the parent constructor function. This allows objects created with the child constructor to inherit properties and methods defined on the parent’s prototype. - ES6 Classes: With the introduction of ES6 (ECMAScript 2015), JavaScript added syntactical support for classes. Classes in JavaScript are essentially syntactic sugar over constructor functions and prototype-based inheritance. You can define classes using the
class
keyword and use theextends
keyword to create inheritance relationships between classes. By extending a class, the child class inherits all the properties and methods from the parent class. You can also override methods in the child class to provide a different implementation.
Regardless of the method used, inheritance in JavaScript allows objects to inherit properties and methods from other objects, enabling code reuse and creating hierarchical relationships. It is important to understand how prototype chains work and choose the appropriate method for implementing inheritance based on the specific requirements of your project.