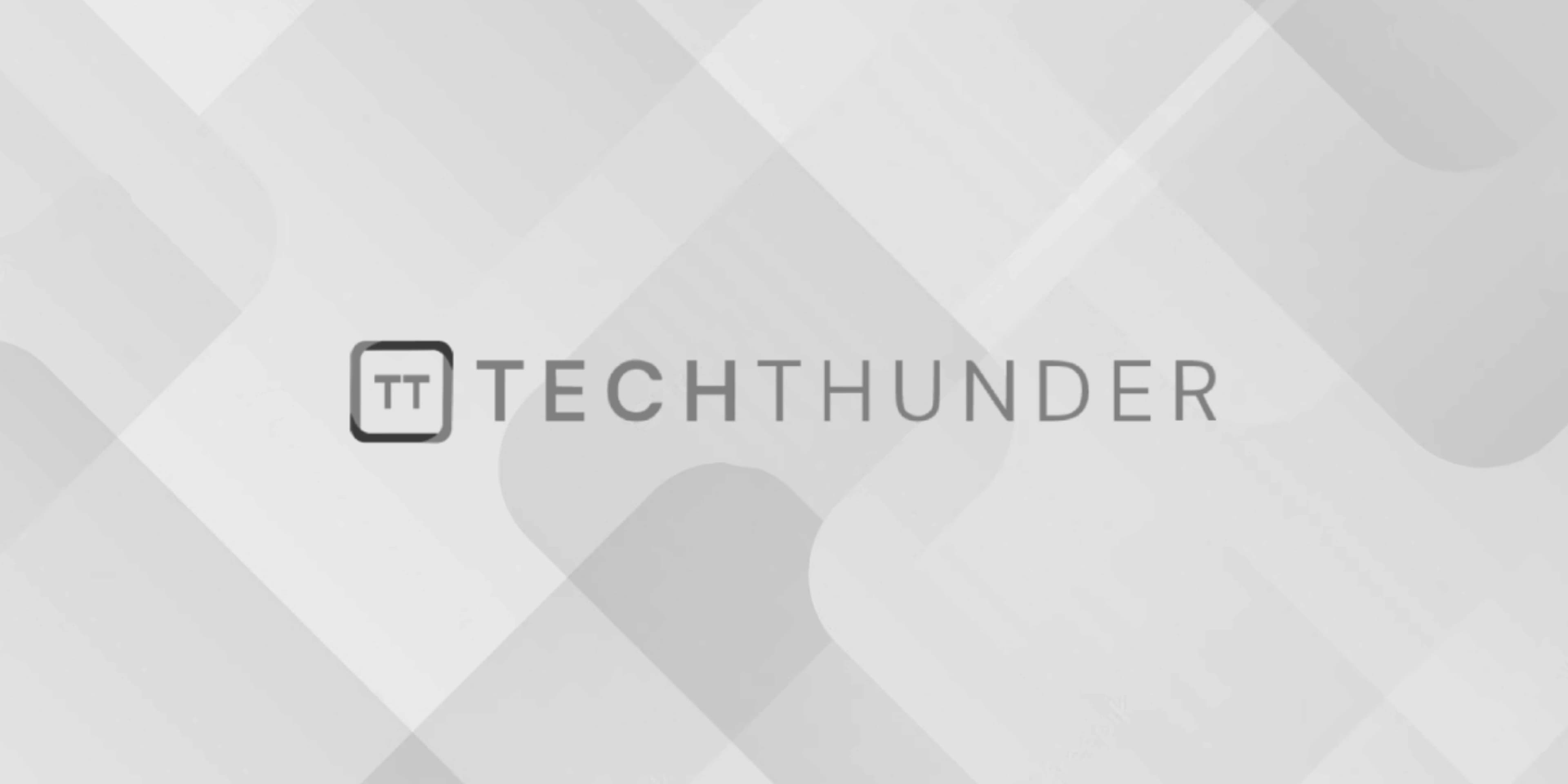
JavaScript typeof operator
The JavaScript typeof
operator is used to determine the data type of a value or variable. It returns a string indicating the type of the operand.
Here are some examples of using the typeof
operator:
console.log(typeof 42); // Output: "number"
console.log(typeof "Hello"); // Output: "string"
console.log(typeof true); // Output: "boolean"
console.log(typeof undefined); // Output: "undefined"
console.log(typeof null); // Output: "object"
console.log(typeof [1, 2, 3]); // Output: "object"
console.log(typeof { name: "John", age: 30 }); // Output: "object"
console.log(typeof function() {}); // Output: "function"
The typeof
operator can be used with any value or variable, including numbers, strings, booleans, objects, arrays, functions, and even null
and undefined
. It returns a string representing the type of the operand.
However, it’s important to note that the typeof
operator has some quirks and limitations. For example, it returns "object"
for arrays and null
, which can be misleading. Also, it treats typeof null
as "object"
instead of "null"
, which is a historical mistake in the JavaScript language.
To perform more precise type checking, it’s recommended to use other methods like instanceof
, Array.isArray()
, or custom type-checking functions.