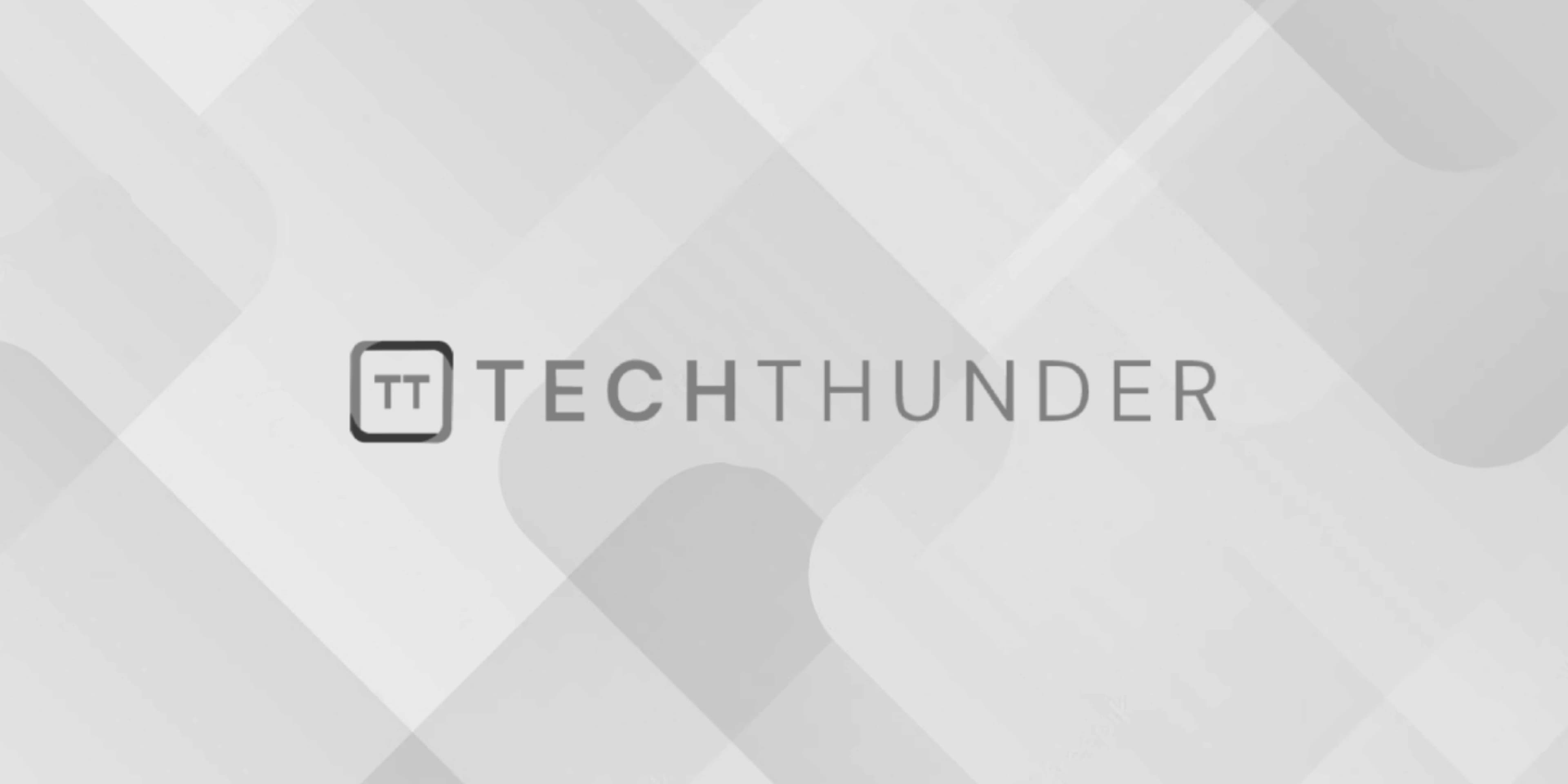
Removing Duplicate From Arrays
To remove duplicates from an array in JavaScript, you can use various approaches. Here are a few common methods:
- Using the Set data structure:
The Set object in JavaScript allows you to store unique values. By converting the array into a Set and then back into an array, you can automatically remove duplicates.
var array = [1, 2, 3, 4, 2, 1, 5, 3];
var uniqueArray = Array.from(new Set(array));
console.log(uniqueArray); // [1, 2, 3, 4, 5]
In this example, the array [1, 2, 3, 4, 2, 1, 5, 3]
is converted to a Set using new Set(array)
. The Set automatically removes duplicates. Finally, Array.from()
is used to convert the Set back into an array.
- Using the filter() method:
Thefilter()
method can be used to create a new array that contains only the unique elements from the original array.
var array = [1, 2, 3, 4, 2, 1, 5, 3];
var uniqueArray = array.filter((value, index, self) => {
return self.indexOf(value) === index;
});
console.log(uniqueArray); // [1, 2, 3, 4, 5]
In this example, the filter()
method is applied to the array, and the callback function checks if the index of the current element (self.indexOf(value)
) is equal to the current index. If they match, it means the element is the first occurrence in the array, and it is added to the new uniqueArray
.
- Using a temporary object or map:
You can iterate over the array and store the unique elements in a temporary object or map. Then, extract the values to get an array without duplicates.
var array = [1, 2, 3, 4, 2, 1, 5, 3];
var uniqueArray = [];
var tempObj = {};
for (var i = 0; i < array.length; i++) {
var element = array[i];
if (!tempObj[element]) {
uniqueArray.push(element);
tempObj[element] = true;
}
}
console.log(uniqueArray); // [1, 2, 3, 4, 5]
In this example, the tempObj
object is used to keep track of unique elements encountered so far. If an element is not present in the tempObj
, it is added to the uniqueArray
and marked as true
in the tempObj
.
These are just a few examples of how you can remove duplicates from an array in JavaScript. Choose the method that best suits your needs and the specific requirements of your application.