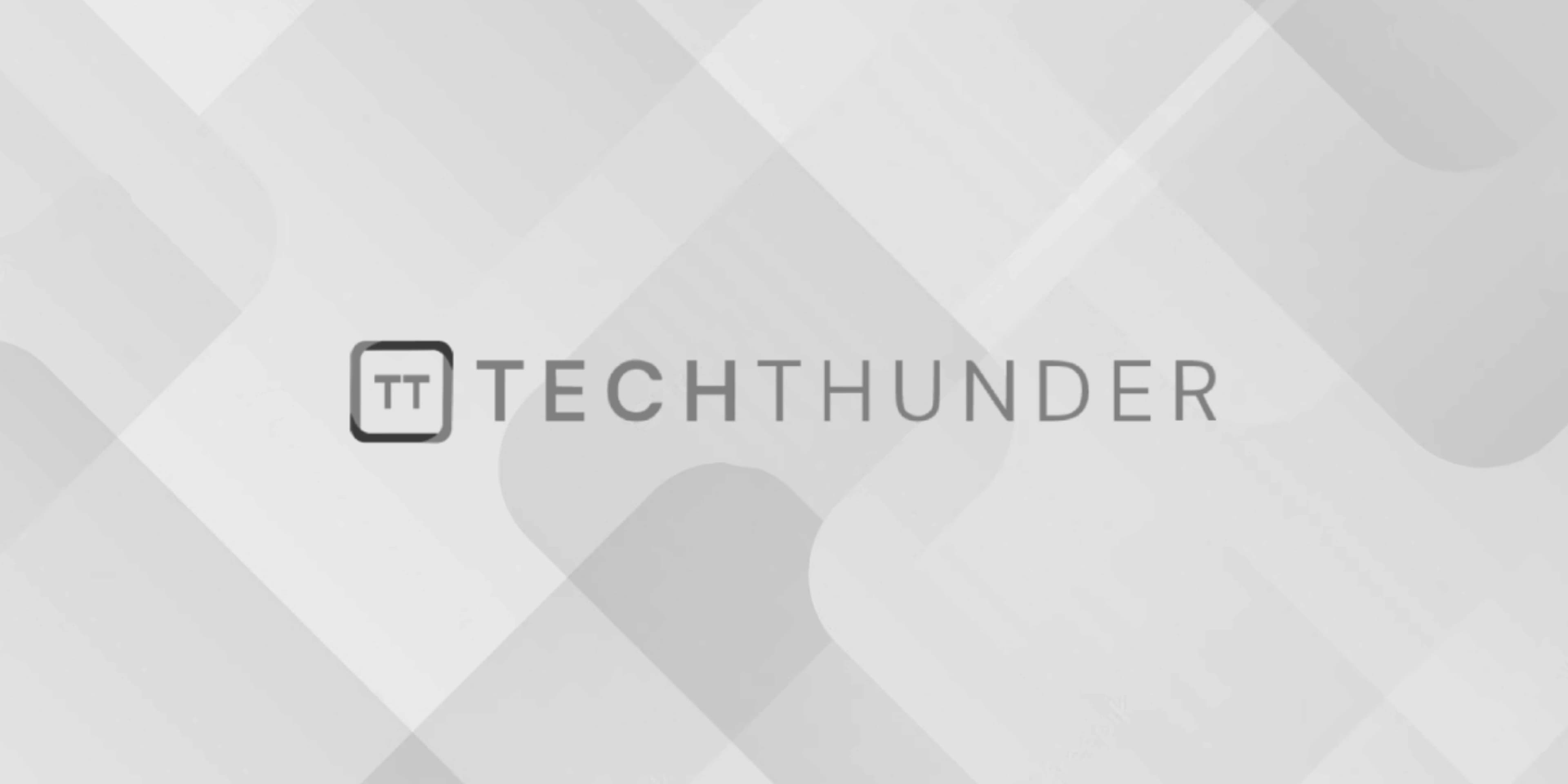
JavaScript Blob
A Blob (Binary Large Object) is a data type used to represent binary data or data of arbitrary file-like objects. It allows you to work with binary data such as images, audio files, or other types of data that can be represented as raw bytes.
To create a Blob object, you can use the Blob
constructor or the Blob()
function. Here’s an example that demonstrates how to create a Blob from a string:
const text = "Hello, world!";
const blob = new Blob([text], { type: 'text/plain' });
In this example, we create a string text
containing the text “Hello, world!”. Then we create a Blob object blob
by passing an array [text]
as the first argument to the Blob
constructor. The second argument is an optional options object that specifies the MIME type of the data. In this case, we set the MIME type to 'text/plain'
to indicate that it’s plain text.
Once you have a Blob object, you can use it in various ways. Here are a few common operations:
- Downloading a Blob: You can create a URL for the Blob using the
URL.createObjectURL()
function and then use it to download the Blob as a file. Here’s an example:
const downloadLink = document.createElement('a');
downloadLink.href = URL.createObjectURL(blob);
downloadLink.download = 'mytextfile.txt';
downloadLink.click();
In this example, we create a download link using createElement('a')
. We set the href
attribute of the link to the URL created by URL.createObjectURL(blob)
. We also set the download
attribute to specify the suggested filename for the downloaded file. Finally, we trigger the download by programmatically clicking the link with click()
.
- Reading the contents of a Blob: You can read the contents of a Blob using the
FileReader
object. Here’s an example that reads the text contents of the Blob:
const reader = new FileReader();
reader.onload = function(event) {
const contents = event.target.result;
console.log(contents);
};
reader.readAsText(blob);
In this example, we create a FileReader
object reader
and set its onload
event handler to retrieve the contents of the Blob when it’s loaded. We then use the readAsText()
method to read the Blob as text.
These are just a few examples of how you can work with Blobs in JavaScript. Blobs provide a flexible way to handle binary data and perform operations such as downloading, reading, or uploading files.