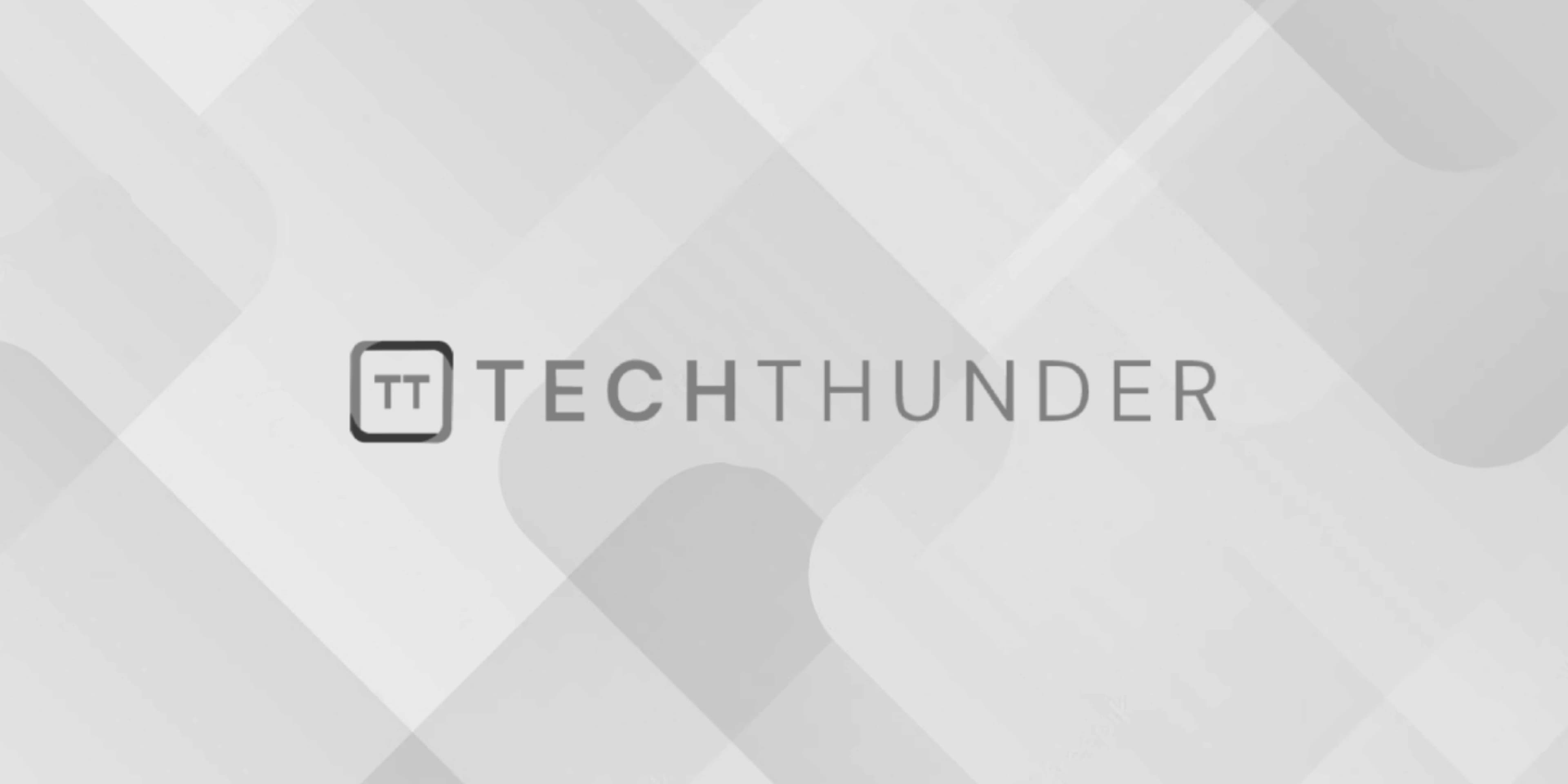
JavaScript email validation
To validate an email address using JavaScript, you can use regular expressions to match the email format. Here’s an example of how to perform email validation in JavaScript:
<input type="text" id="emailInput">
<button onclick="validateEmail()">Submit</button>
<script>
function validateEmail() {
// Get the email input value
var email = document.getElementById("emailInput").value;
// Regular expression pattern for email validation
var emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
// Check if the email matches the pattern
if (emailPattern.test(email)) {
alert("Valid email address");
} else {
alert("Invalid email address");
}
}
</script>
In the example above, an input field with the id “emailInput” is used to collect the email address. The validateEmail()
function is called when the submit button is clicked. Inside the function, the value of the email input is retrieved.
A regular expression pattern emailPattern
is defined to match the email format. The pattern checks for alphanumeric characters, dots, dashes, and the presence of a domain extension with at least two characters.
The test()
method is used to check if the email matches the pattern. If it does, an alert message saying “Valid email address” is displayed. Otherwise, an alert with “Invalid email address” is shown.
You can modify the regular expression pattern to suit your specific email validation requirements.