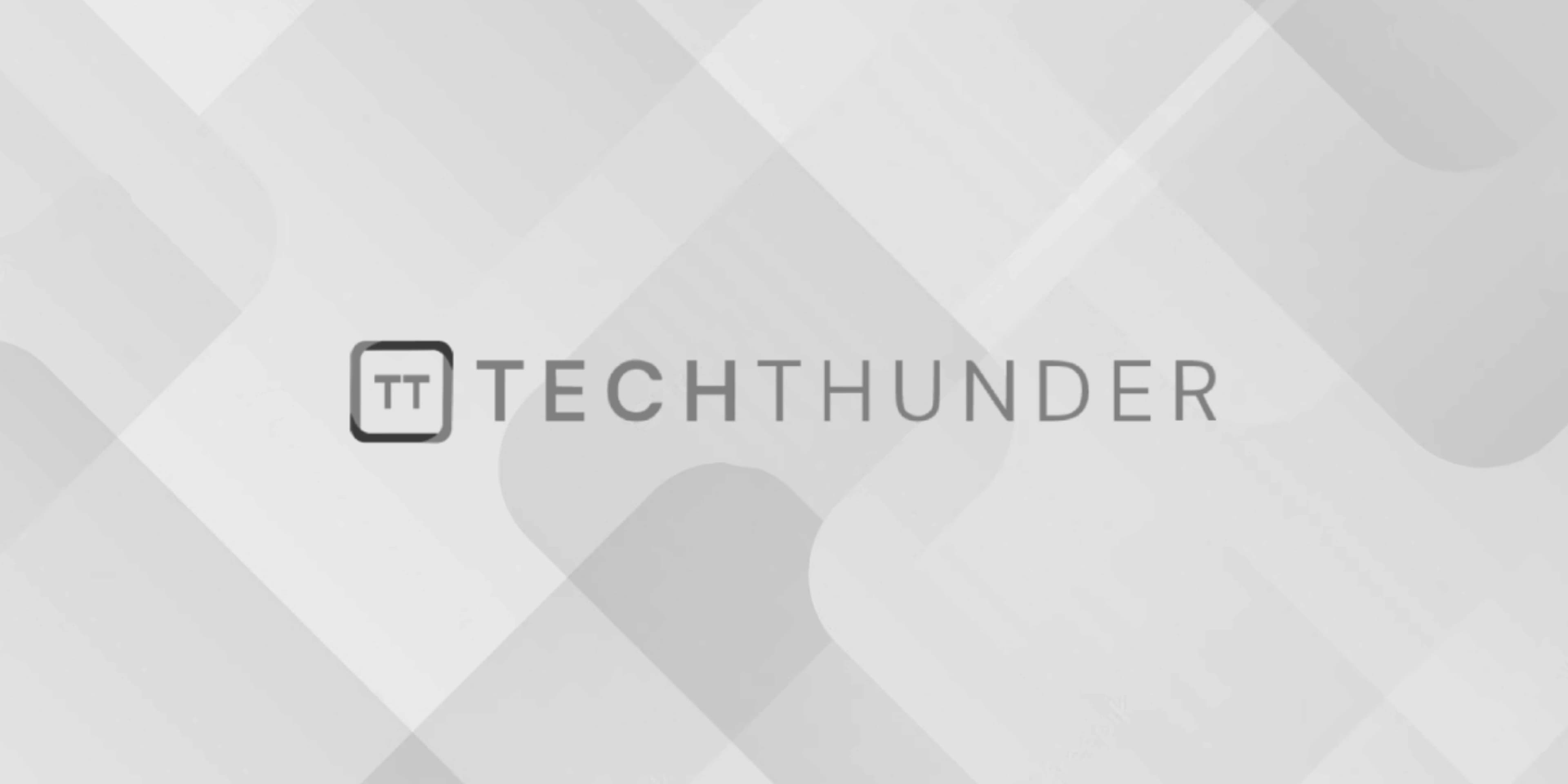
How to get all checked checkbox value in JavaScript
To retrieve the values of all checked checkboxes using JavaScript, you can iterate over the checkboxes and check their checked
property to identify which ones are selected. Here’s an example:
HTML:
<input type="checkbox" name="option" value="option1">
<input type="checkbox" name="option" value="option2">
<input type="checkbox" name="option" value="option3">
JavaScript:
// Get all checkboxes by their name attribute
var checkboxes = document.getElementsByName('option');
// Array to store the values of checked checkboxes
var checkedValues = [];
// Iterate over the checkboxes
for (var i = 0; i < checkboxes.length; i++) {
var checkbox = checkboxes[i];
// Check if the checkbox is checked
if (checkbox.checked) {
// Add the value to the checkedValues array
checkedValues.push(checkbox.value);
}
}
// Display the values of checked checkboxes
console.log(checkedValues);
In this example, the HTML code contains three checkboxes with the same name attribute (option
), but with different values.
The JavaScript code starts by retrieving all checkboxes with the name attribute option
using the getElementsByName()
method. The checkboxes are stored in the checkboxes
variable as a collection.
Next, an empty array called checkedValues
is created to store the values of the checked checkboxes.
The for
loop iterates over each checkbox in the checkboxes
collection. For each checkbox, it checks the checked
property to determine if it is selected.
If a checkbox is checked, its value is added to the checkedValues
array using the push()
method.
Finally, the values of the checked checkboxes are displayed in the console using console.log()
.
By executing this code, you will obtain an array (checkedValues
) containing the values of all the checked checkboxes.