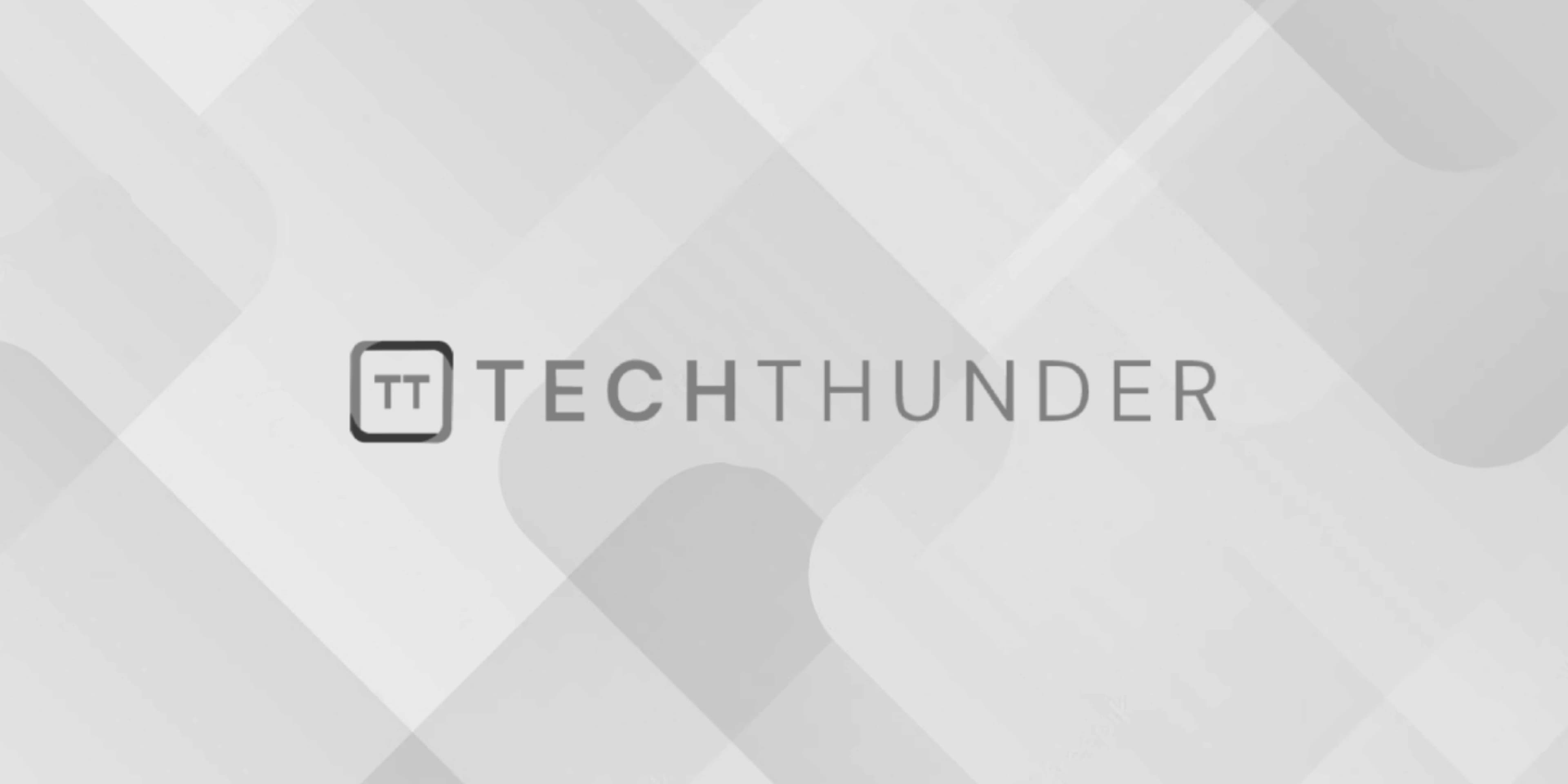
How to draw a line using javascript
To draw a line using JavaScript, you can utilize the HTML5 canvas element. The canvas element provides a drawing surface on which you can render graphics using JavaScript. Here’s an example of how to draw a line:
// Get the canvas element
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
// Set the starting and ending points of the line
const startX = 50;
const startY = 50;
const endX = 200;
const endY = 200;
// Set the line color and width
context.strokeStyle = 'blue';
context.lineWidth = 2;
// Begin drawing the line
context.beginPath();
// Set the starting point of the line
context.moveTo(startX, startY);
// Set the ending point of the line
context.lineTo(endX, endY);
// End drawing the line
context.stroke();
In this example, we first retrieve the canvas element using document.getElementById()
. Then, we obtain the 2D rendering context of the canvas using canvas.getContext('2d')
. Next, we define the starting and ending points of the line using startX
, startY
, endX
, and endY
variables.
We set the line color using context.strokeStyle
and the line width using context.lineWidth
. You can adjust these properties to customize the appearance of the line.
After that, we begin the path for drawing using context.beginPath()
. We set the starting point of the line using context.moveTo(startX, startY)
and the ending point using context.lineTo(endX, endY)
.
Finally, we call context.stroke()
to actually draw the line on the canvas.
You will need to create an HTML element with the id “myCanvas” to contain the canvas element in your HTML markup. For example:
<canvas id="myCanvas" width="300" height="300"></canvas>
This code will create a canvas element with a width and height of 300 pixels. The drawn line will appear within this canvas element.
By modifying the coordinates and styling properties, you can draw lines in different positions and with different colors and widths.