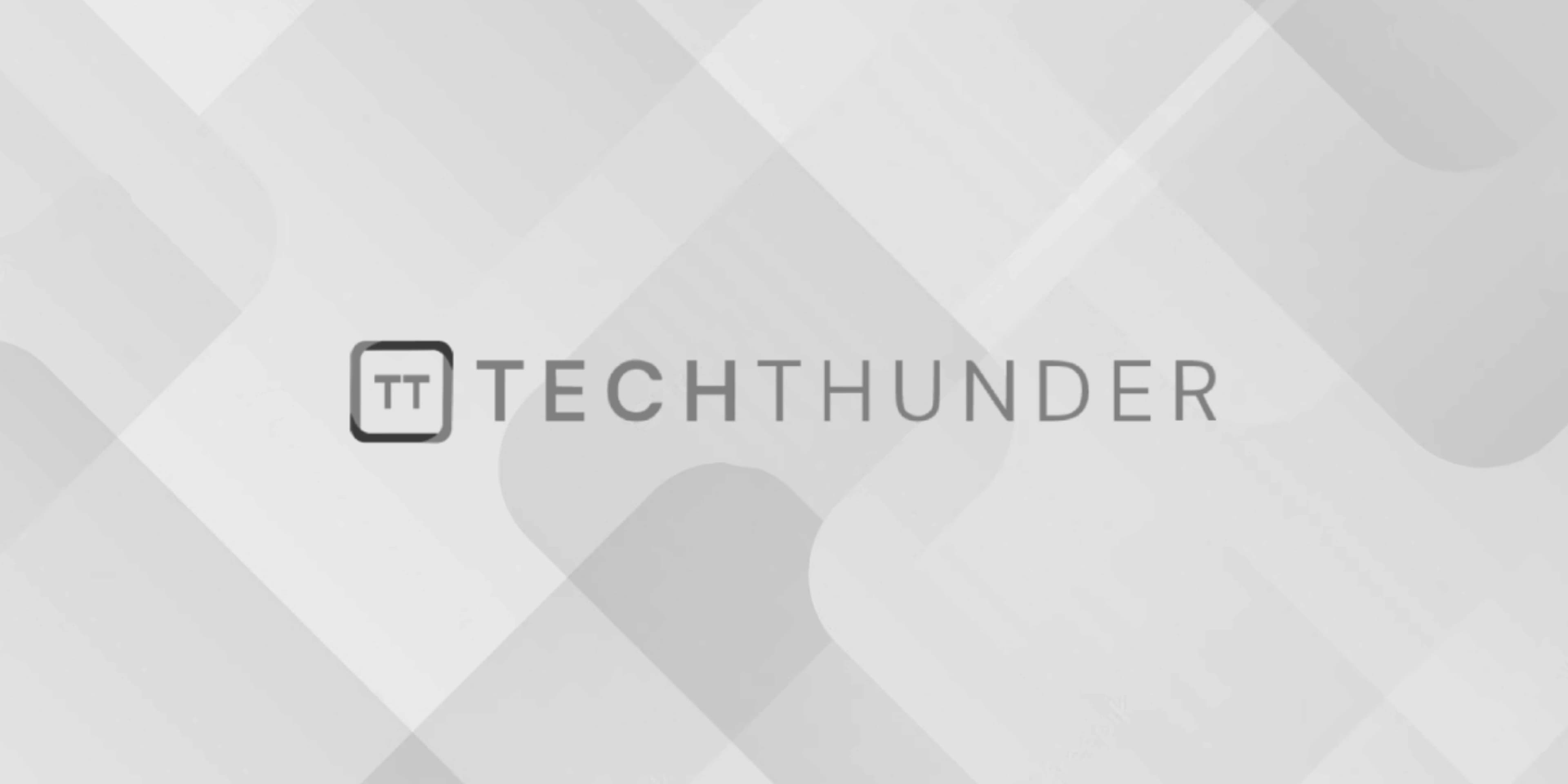
JavaScript callback
The JavaScript callback is a function that is passed as an argument to another function and is executed at a later point in time. Callback functions are commonly used in asynchronous programming to handle the completion of an operation or to respond to events.
Here’s an example of how to use a callback function in JavaScript:
function fetchData(callback) {
// Simulating an asynchronous operation (e.g., fetching data from a server)
setTimeout(() => {
const data = { id: 1, name: 'John Doe' };
callback(data); // Invoke the callback function with the fetched data
}, 2000);
}
// Define a callback function
function processResult(data) {
console.log('Received data:', data);
// Perform further processing or manipulation of the received data
}
// Call the fetchData function and pass the callback function as an argument
fetchData(processResult);
In the above example, the fetchData
function simulates an asynchronous operation by using setTimeout
. It takes a callback function as an argument and executes it after a delay of 2 seconds. Once the operation is complete and the data is fetched, the callback function processResult
is invoked with the fetched data.
Callbacks are commonly used in scenarios such as making AJAX requests, handling events, working with timers, and performing other asynchronous operations. They allow you to define custom behavior that should occur when a certain operation completes or an event occurs. Callbacks are an essential concept in JavaScript for building asynchronous and event-driven applications.