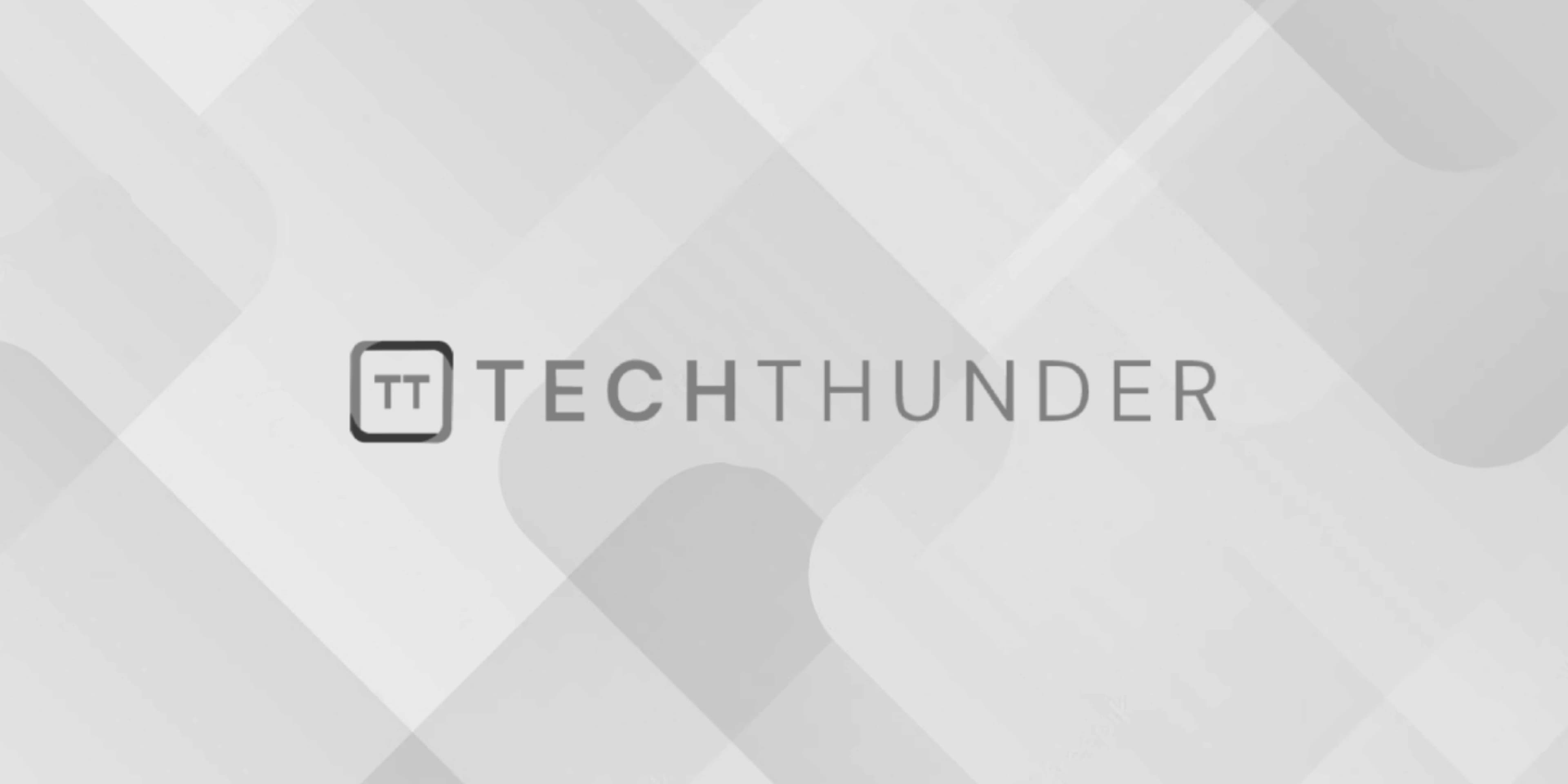
Javacript – document.getElementsByClassName()
The JavaScript document.getElementsByClassName()
method is used to get a collection of all the elements in the document with a specific class name. It returns a HTMLCollection
object, which is an ordered collection of elements with the same class name.
The getElementsByClassName()
method takes a class name as its parameter and returns a HTMLCollection
object that contains all the elements in the document with the specified class name.
The getElementsByClassName()
method is called with the argument “myClass” to get all the <p>
elements with the class name “myClass” in the document. The returned HTMLCollection
object is then logged to the console, which will output an array-like object containing all the matching <p>
elements in the document.
Note that the HTMLCollection
object is not a true array and therefore cannot be manipulated using array methods, but it can be looped over using a for
loop or converted to an array using Array.from()
.
Here is an example of how to use getElementsByClassName()
method to get all the <p>
elements with the class name “myClass” in an HTML document:
<html>
<head>
<title>Example</title>
<style>
.myClass {
color: red;
}
</style>
</head>
<body>
<p class="myClass">Paragraph 1</p>
<p class="myClass">Paragraph 2</p>
<script>
const paragraphs = document.getElementsByClassName("myClass");
console.log(paragraphs); // Output: HTMLCollection[<p>, <p>]
</script>
</body>
</html>