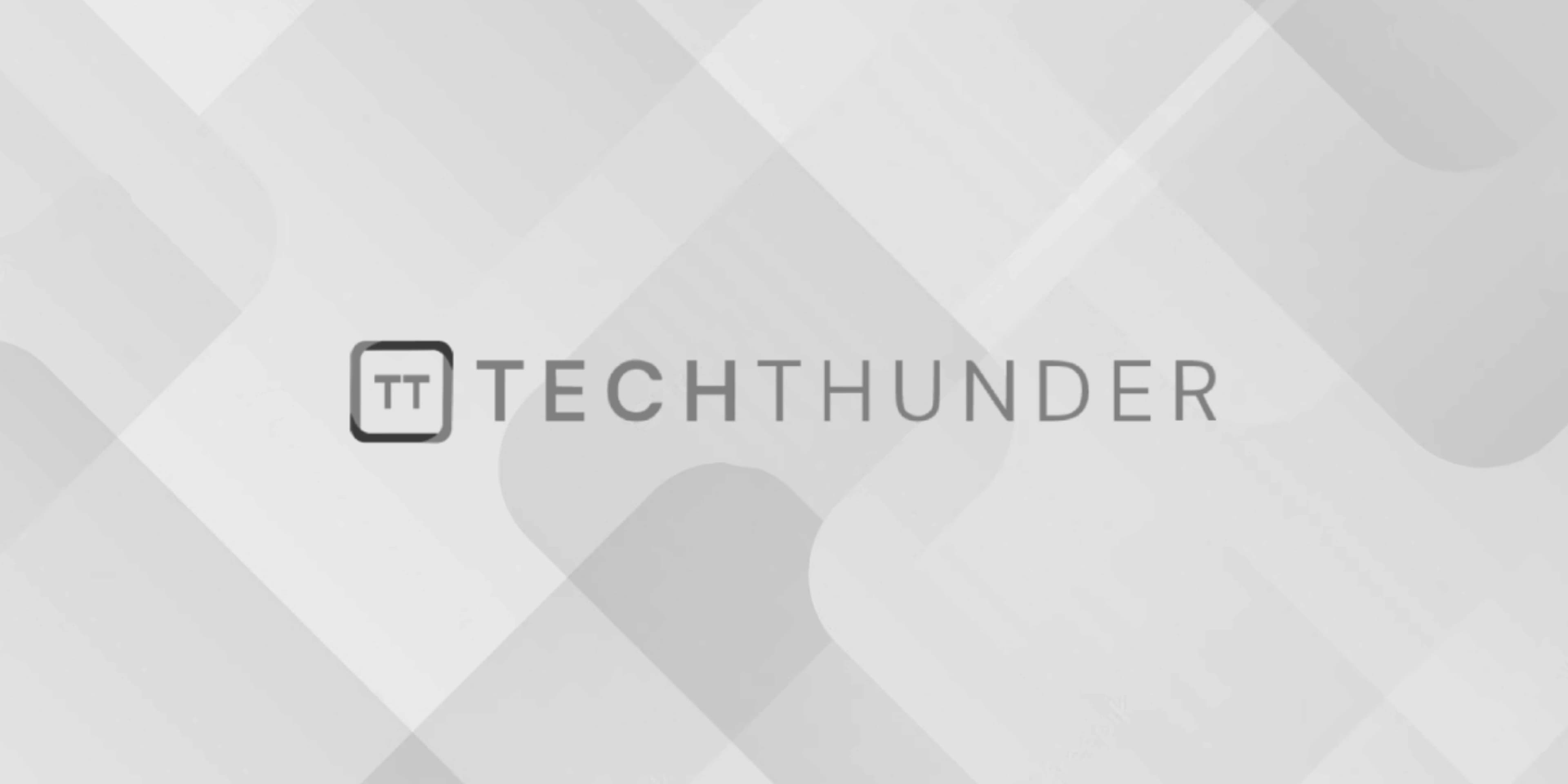
Convert object to array in Javascript
To convert an object to an array in JavaScript, you can use several approaches depending on the desired structure of the resulting array. Here are a few common methods:
- Object.values():
TheObject.values()
method retrieves the enumerable property values of an object and returns them as an array.
const obj = { a: 1, b: 2, c: 3 };
const arr = Object.values(obj);
console.log(arr); // Output: [1, 2, 3]
In this example, Object.values(obj)
returns an array [1, 2, 3]
containing the values of the properties in the object obj
.
- Object.entries():
TheObject.entries()
method returns an array of an object’s own enumerable string-keyed property [key, value] pairs.
const obj = { a: 1, b: 2, c: 3 };
const arr = Object.entries(obj);
console.log(arr); // Output: [['a', 1], ['b', 2], ['c', 3]]
In this example, Object.entries(obj)
returns an array [['a', 1], ['b', 2], ['c', 3]]
containing the key-value pairs of the properties in the object obj
.
- Object.keys() with Array.map():
If you only want to extract the keys or values from an object, you can useObject.keys()
along withArray.map()
. To get an array of keys:
const obj = { a: 1, b: 2, c: 3 };
const keys = Object.keys(obj);
console.log(keys); // Output: ['a', 'b', 'c']
To get an array of values:
const obj = { a: 1, b: 2, c: 3 };
const values = Object.keys(obj).map(key => obj[key]);
console.log(values); // Output: [1, 2, 3]
In the second example, Object.keys(obj)
returns an array ['a', 'b', 'c']
containing the keys of the properties in the object obj
. Then, Array.map()
is used to iterate over the keys and retrieve the corresponding values from the object obj
, resulting in the array [1, 2, 3]
containing the property values.
These methods provide different ways to convert an object to an array based on the specific requirements you have for the resulting array structure.