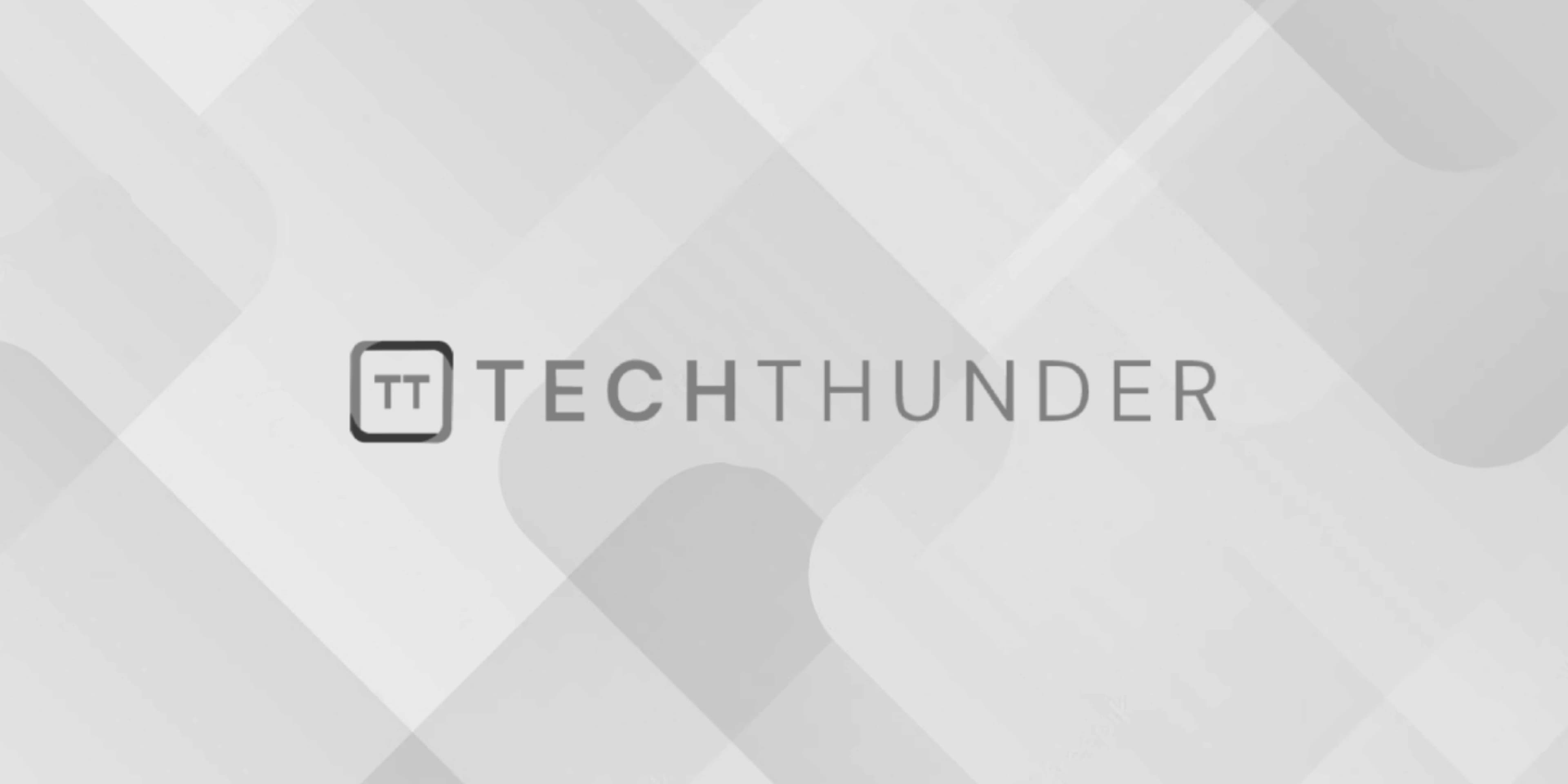
How to Iterate through a JavaScript Object
To iterate through a JavaScript object, you can use several methods depending on your requirements. Here are three common approaches:
- for…in loop:
Thefor...in
loop allows you to iterate over the enumerable properties of an object. It iterates through each property, including inherited properties from the object’s prototype chain. Here’s an example:
var obj = {
name: 'John',
age: 30,
city: 'New York'
};
for (var key in obj) {
if (obj.hasOwnProperty(key)) {
console.log(key + ': ' + obj[key]);
}
}
In the example above, we use a for...in
loop to iterate through the properties of the obj
object. The hasOwnProperty()
method is used to ensure that only the object’s own properties are processed, excluding inherited properties. Inside the loop, we can access the property key and its corresponding value using obj[key]
.
- Object.keys() method:
TheObject.keys()
method returns an array of an object’s own enumerable property keys. You can then iterate over this array using a loop or array methods likeforEach()
. Here’s an example:
var obj = {
name: 'John',
age: 30,
city: 'New York'
};
Object.keys(obj).forEach(function(key) {
console.log(key + ': ' + obj[key]);
});
In this example, we use Object.keys(obj)
to get an array of the object’s own property keys. Then, we use the forEach()
method to iterate over the array, accessing each property key and its corresponding value inside the callback function.
- Object.entries() method:
TheObject.entries()
method returns an array of an object’s own enumerable property key-value pairs as arrays. You can then iterate over this array using a loop or array methods. Here’s an example:
var obj = {
name: 'John',
age: 30,
city: 'New York'
};
Object.entries(obj).forEach(function([key, value]) {
console.log(key + ': ' + value);
});
In this example, we use Object.entries(obj)
to get an array of the object’s own property key-value pairs. Then, we use the forEach()
method to iterate over the array. Inside the callback function, we use destructuring to access the property key and its corresponding value.
These methods provide different ways to iterate through a JavaScript object. Choose the one that best suits your needs based on whether you want to iterate over all properties (including inherited ones), only the object’s own properties, or the key-value pairs of the object.