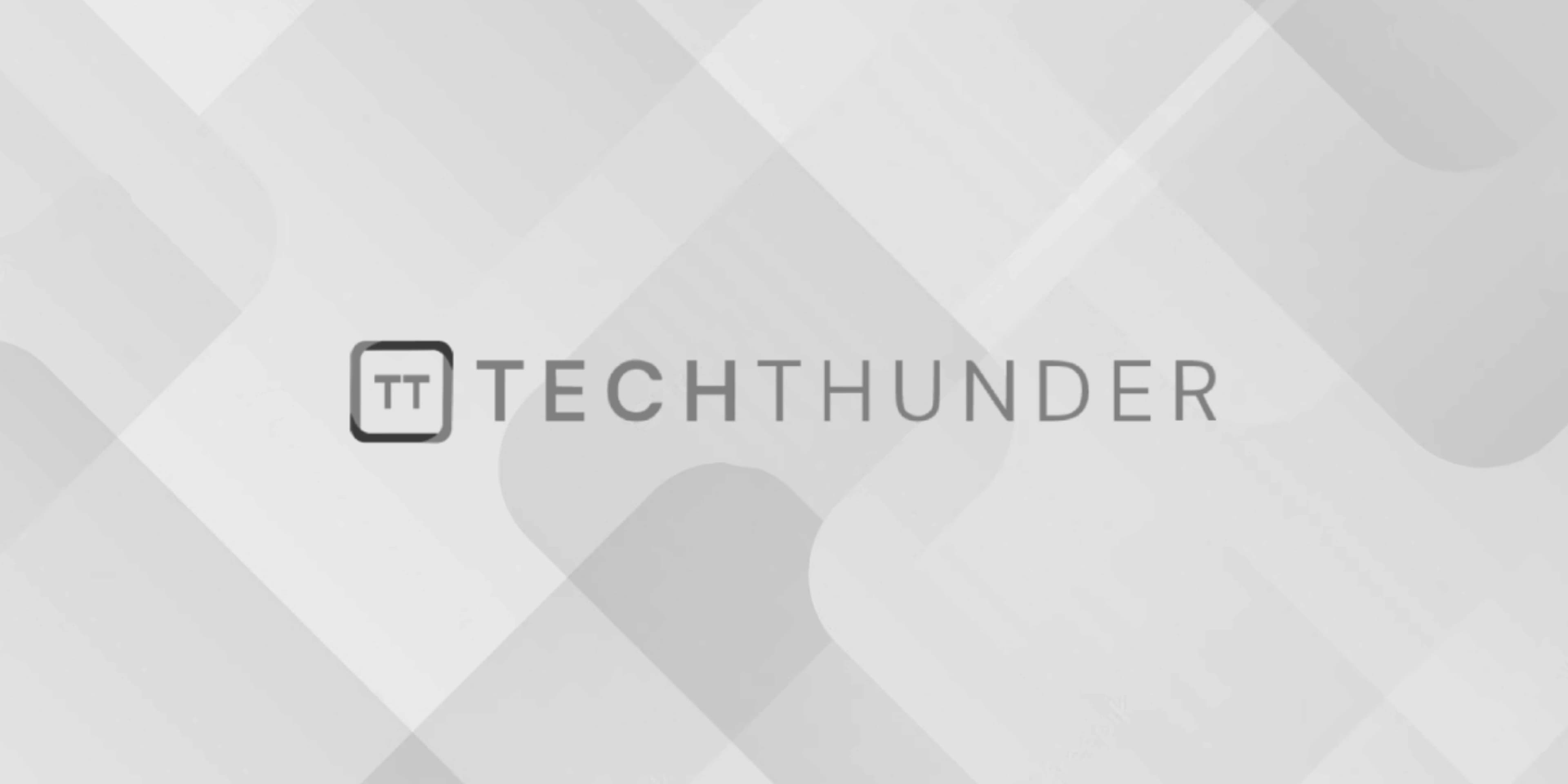
JavaScript scope
JavaScript has function scope, meaning that variables and functions defined within a function are only accessible within that function and its nested functions. However, starting from ECMAScript 2015 (ES6), JavaScript also introduced block scope with the let
and const
keywords.
Let’s take a closer look at JavaScript’s scope rules:
- Global Scope: Variables and functions defined outside of any function or block have global scope. They are accessible throughout the entire JavaScript program.
var globalVariable = 10;
function globalFunction() {
console.log(globalVariable); // Accessible here
}
console.log(globalVariable); // Accessible here
globalFunction(); // Function call
- Function Scope: Variables and functions declared within a function have function scope, which means they are only accessible within that function (including any nested functions).
function outerFunction() {
var outerVariable = 20;
function innerFunction() {
console.log(outerVariable); // Accessible here
}
console.log(outerVariable); // Accessible here
innerFunction(); // Function call
}
outerFunction(); // Function call
- Block Scope (with
let
andconst
): Starting from ES6, thelet
andconst
keywords introduced block scope. Variables declared withlet
orconst
are scoped to the nearest enclosing block (i.e., within curly braces{}
). This includes loops, conditionals, and any block statements.
function blockScopeExample() {
if (true) {
var x = 10; // Function-scoped variable
let y = 20; // Block-scoped variable
console.log(x); // 10
console.log(y); // 20
}
console.log(x); // 10
console.log(y); // Error: y is not defined
}
blockScopeExample();
In the example above, the variable x
declared with var
is function-scoped and accessible both inside and outside the if
block. However, the variable y
declared with let
is block-scoped and only accessible within the if
block.
It’s important to note that variables declared with var
have function scope, even if they are declared within a block. This behavior can sometimes lead to unexpected results or confusion. Therefore, it is generally recommended to use let
or const
for block-scoped variables.
Understanding JavaScript scope is crucial for writing clean and maintainable code and avoiding naming conflicts between variables.