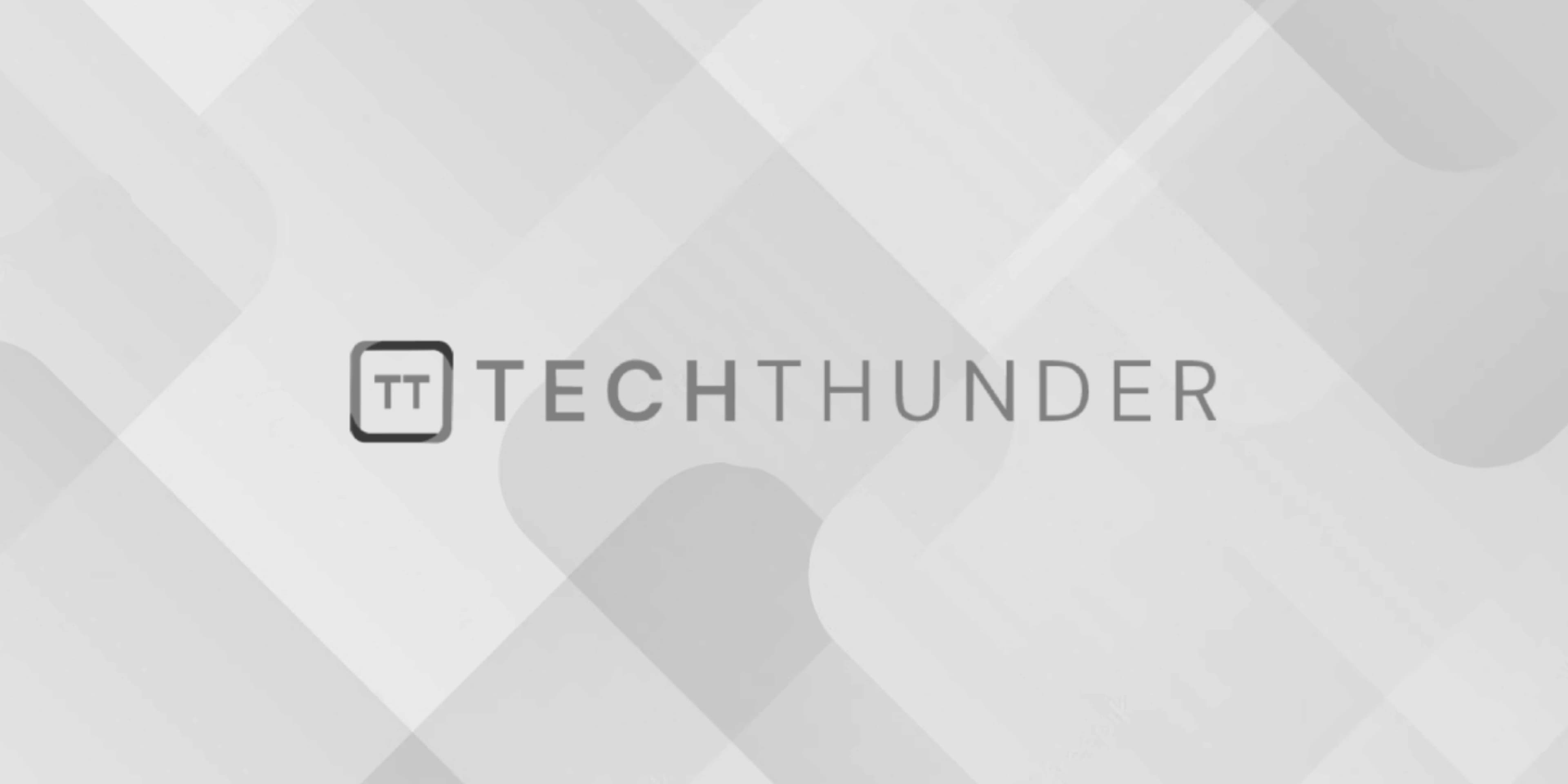
218 views
JavaScript Hoisting
JavaScript hoisting is a behavior in which variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that you can use variables and functions before they are declared in your JavaScript code.
Here are a few key points to understand about hoisting:
- Variable Hoisting: In JavaScript, variable declarations using the
var
keyword are hoisted to the top of their scope, but the assignment or initialization of the variable remains in its original position. This means that you can access and use a variable before it’s declared, but its value will beundefined
until it’s assigned a value.
JavaScript
console.log(myVariable); // Output: undefined
var myVariable = "Hello";
- Function Hoisting: Function declarations are completely hoisted, including both the function name and the function body. This means you can call a function before it’s defined in the code.
JavaScript
sayHello(); // Output: "Hello!"
function sayHello() {
console.log("Hello!");
}
- Function Expressions: Function expressions, on the other hand, are not hoisted. Only the variable declaration is hoisted, not the assignment of the function expression.
JavaScript
sayHello(); // Output: TypeError: sayHello is not a function
var sayHello = function() {
console.log("Hello!");
};
It’s important to note that while hoisting allows you to use variables and functions before they are declared, it’s considered best practice to declare and initialize variables at the beginning of their scope and define functions before using them. This promotes code readability and reduces potential issues caused by hoisting.