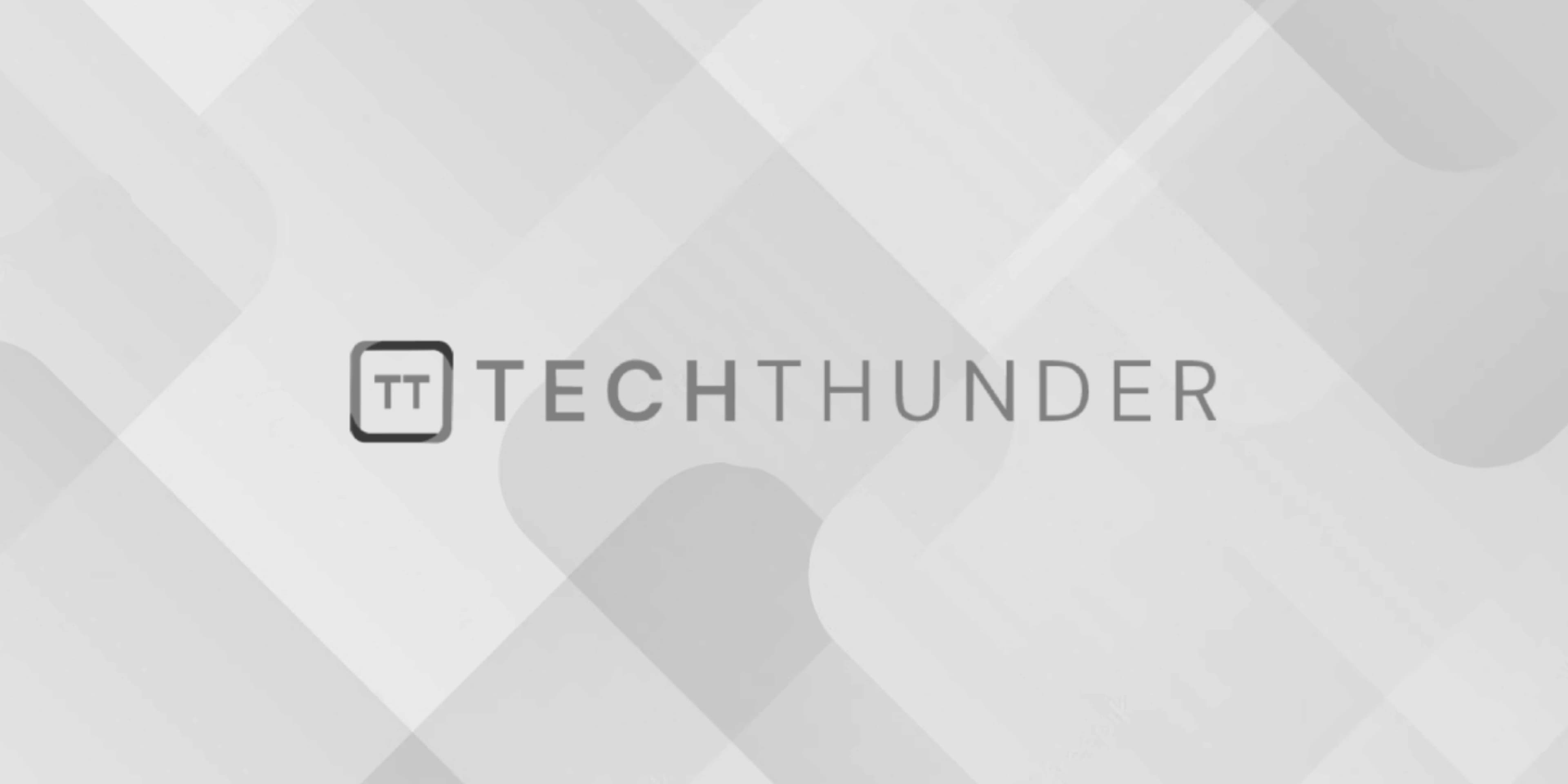
JavaScript onresize event
The onresize
event in JavaScript is triggered when the size of the browser window or an element is changed. It allows you to execute a JavaScript function or perform certain actions whenever the resizing event occurs.
To use the onresize
event, you can attach an event handler to the window
object or to a specific element using JavaScript. Here’s an example of how to use the onresize
event with the window
object:
window.onresize = function() {
// Code to be executed when the window is resized
console.log('Window has been resized');
};
In this example, whenever the window is resized, the anonymous function assigned to the onresize
event will be executed, and it will log a message to the console.
You can also use the addEventListener
method to attach the resize
event to the window
object. Here’s an example:
window.addEventListener('resize', function() {
// Code to be executed when the window is resized
console.log('Window has been resized');
});
Using the addEventListener
method allows you to attach multiple event handlers to the resize
event or easily remove the event handler using the removeEventListener
method.
Similarly, you can attach the onresize
event to specific HTML elements by targeting them using their id
, class
, or other selectors. For example:
var element = document.getElementById('myElement');
element.onresize = function() {
// Code to be executed when the element is resized
console.log('Element has been resized');
};
Remember to replace 'myElement'
with the actual ID of the element you want to target.
By utilizing the onresize
event, you can dynamically adjust the layout, update content, or perform any other necessary actions based on the changes in the window or element size.