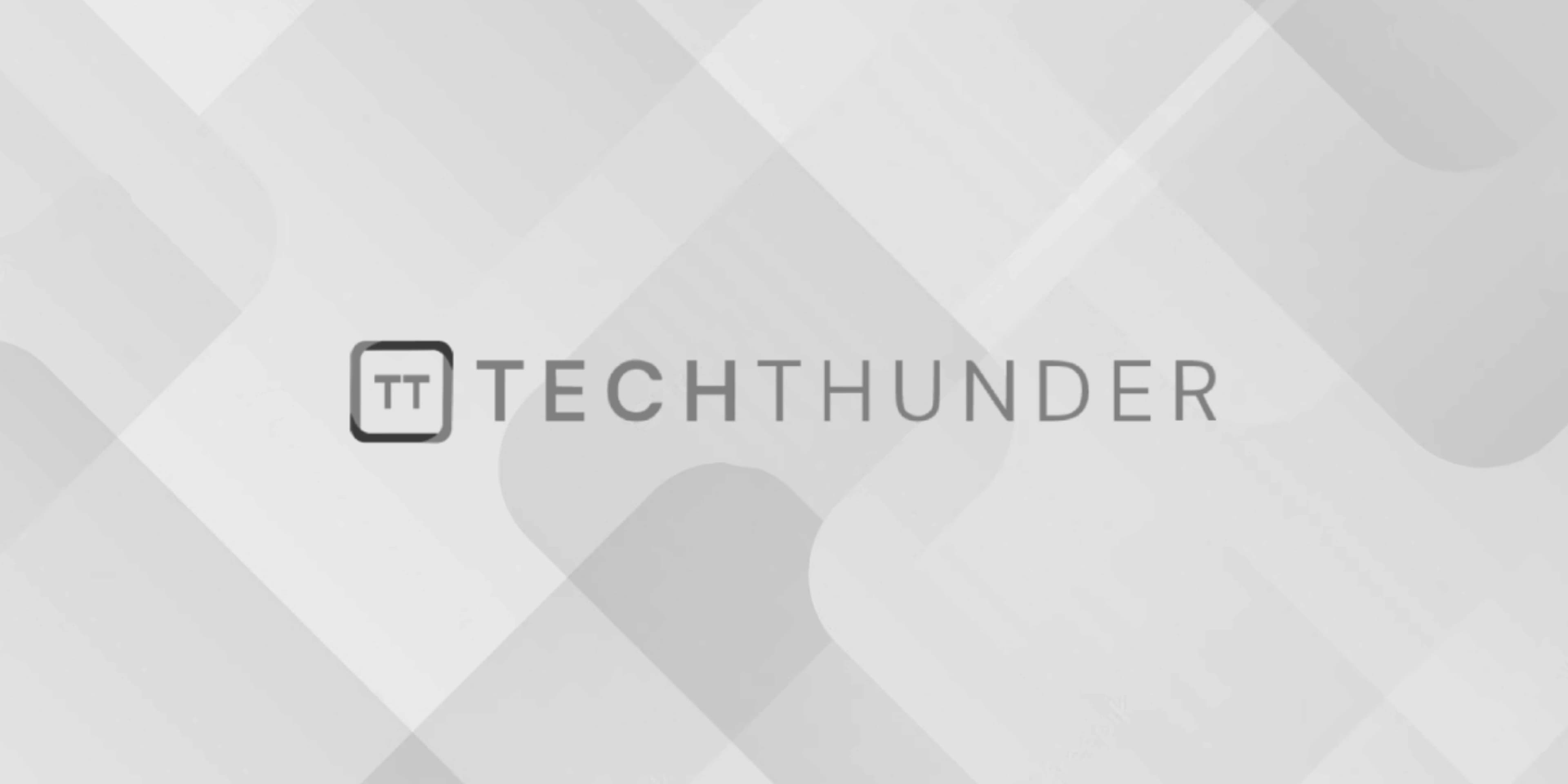
JavaScript Select Option
To manipulate a <select>
element and retrieve the selected option using JavaScript, you can utilize the selectedIndex
and options
properties of the <select>
element. Here’s an example:
HTML:
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
JavaScript:
// Get the select element by its ID or any other method
var selectElement = document.getElementById("mySelect");
// Get the selected option's value
var selectedValue = selectElement.options[selectElement.selectedIndex].value;
// Get the selected option's text
var selectedText = selectElement.options[selectElement.selectedIndex].text;
// Display the selected option's value and text
console.log("Selected Value: " + selectedValue);
console.log("Selected Text: " + selectedText);
In this example, the HTML code contains a <select>
element with an ID of "mySelect"
. It has multiple <option>
elements representing the selectable options.
The JavaScript code retrieves the <select>
element using document.getElementById()
or any other suitable method. Replace "mySelect"
with the actual ID or method that identifies your <select>
element.
To get the selected option’s value, you access the options
property of the <select>
element, followed by the selectedIndex
property. The selectedIndex
property gives the index of the currently selected option. Then, you retrieve the value using options[selectElement.selectedIndex].value
.
Similarly, to get the selected option’s text, you access the options
property and retrieve the text using options[selectElement.selectedIndex].text
.
Finally, the selected option’s value and text are displayed in the console using console.log()
.
By executing this code, you will obtain the value and text of the currently selected option in the <select>
element.