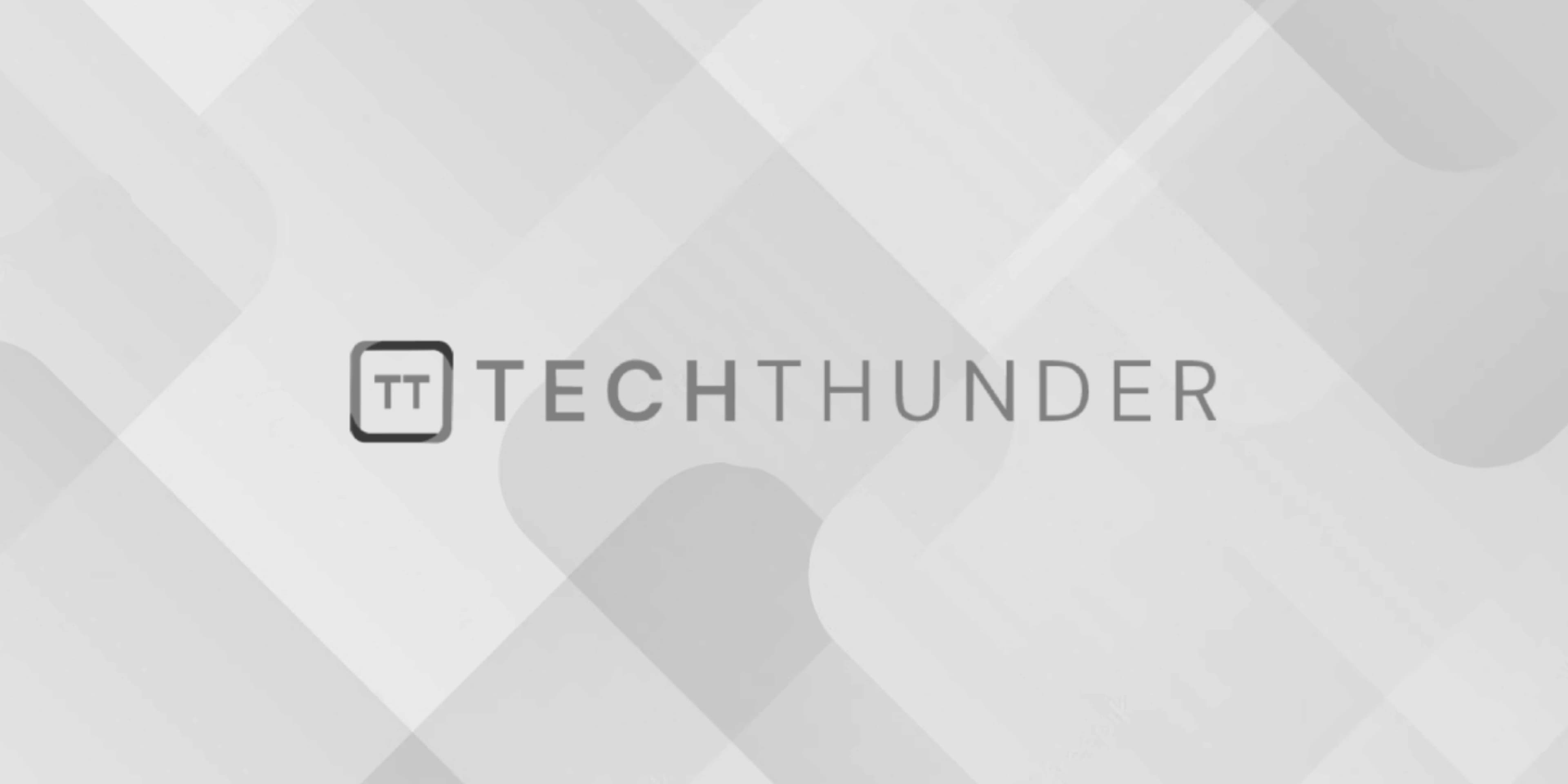
393 views
JavaScript Array
The JavaScript array is a data structure that allows you to store multiple values in a single variable. It is defined using square brackets [] and can contain any combination of data types, including numbers, strings, objects, and even other arrays.
JavaScript
let myArray = [1, 'two', true, {name: 'John'}, [4, 5, 6]];
You can access individual elements of an array by their index, which starts at 0 for the first element. For example, to access the second element of the array above, you would use:
JavaScript
console.log(myArray[1]); // Output: 'two'
You can also add, remove, or modify elements of an array using various built-in methods such as push()
, pop()
, shift()
, unshift()
, splice()
, and slice()
. Here are some examples:
JavaScript
myArray.push(7); // adds 7 to the end of the array
console.log(myArray); // Output: [1, 'two', true, {name: 'John'}, [4, 5, 6], 7]
myArray.pop(); // removes the last element (7) from the array
console.log(myArray); // Output: [1, 'two', true, {name: 'John'}, [4, 5, 6]]
myArray.shift(); // removes the first element (1) from the array
console.log(myArray); // Output: ['two', true, {name: 'John'}, [4, 5, 6]]
myArray.unshift(0); // adds 0 to the beginning of the array
console.log(myArray); // Output: [0, 'two', true, {name: 'John'}, [4, 5, 6]]
myArray.splice(2, 0, 'three'); // adds 'three' at index 2 and shifts the following elements
console.log(myArray); // Output: [0, 'two', 'three', true, {name: 'John'}, [4, 5, 6]]
let slicedArray = myArray.slice(2, 4); // creates a new array containing elements at index 2 and 3
console.log(slicedArray); // Output: ['three', true]