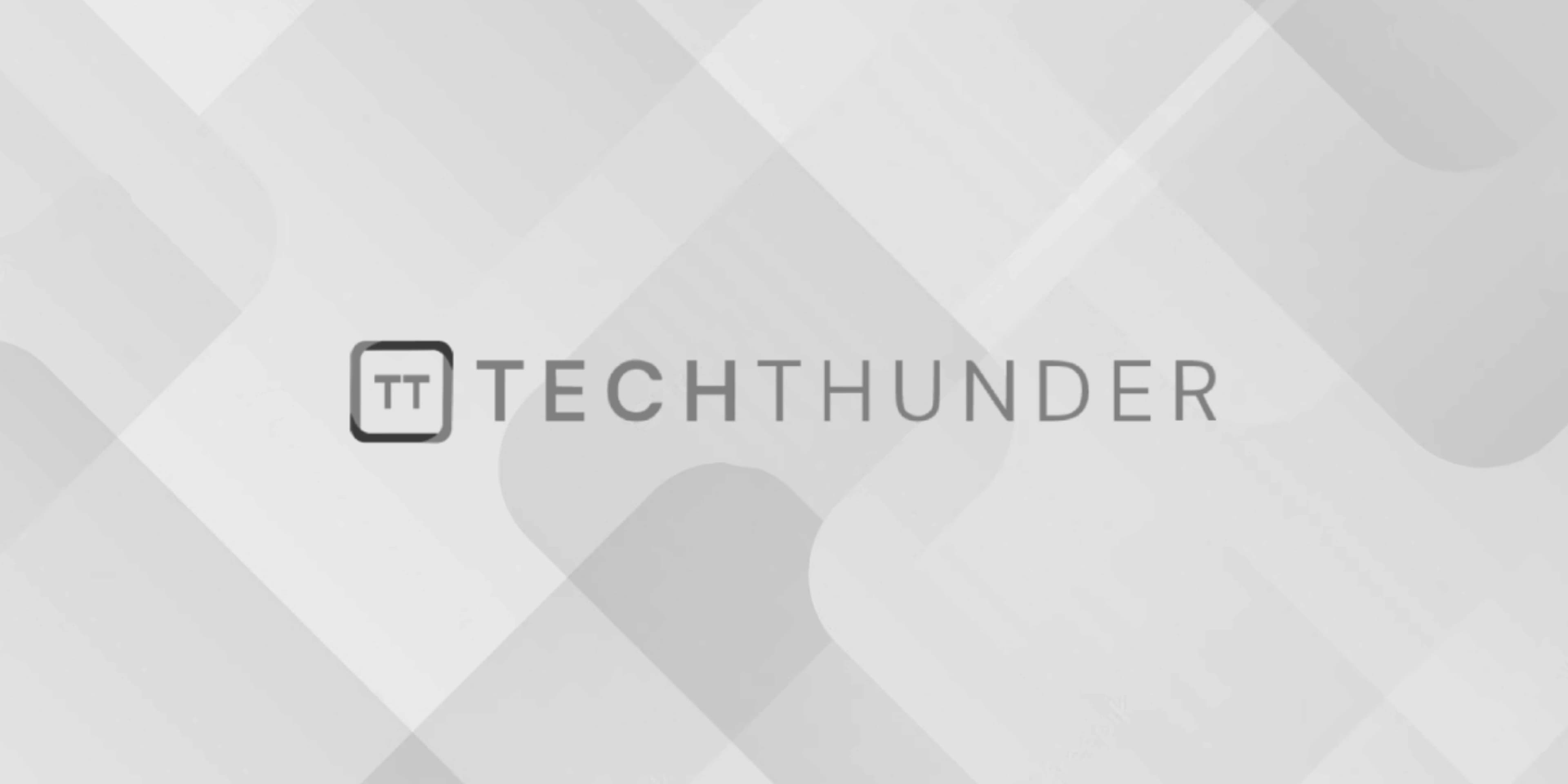
JavaScript label statement
The label
statement in JavaScript is used to provide a labeled statement that can be referenced elsewhere in the code using the break
or continue
statements. It is primarily used in conjunction with loops, such as for
or while
, to control the flow of the program.
Here’s an example that demonstrates the usage of the label
statement:
outerLoop: for (let i = 0; i < 3; i++) {
console.log('Outer loop:', i);
innerLoop: for (let j = 0; j < 3; j++) {
if (j === 1) {
break outerLoop; // Breaking out of the outer loop
}
console.log('Inner loop:', j);
}
}
In this example, we have an outer loop labeled as outerLoop
and an inner loop labeled as innerLoop
. The break
statement with the label outerLoop
is used to exit the entire loop structure when j
is equal to 1. As a result, the outer loop is terminated, and the program execution continues after the loop.
Similarly, you can use the continue
statement with a label to skip the current iteration and move to the next iteration of a specific loop.
It’s important to note that the usage of labeled statements is generally considered as a less common feature of JavaScript and can make the code harder to read and maintain. Therefore, it is recommended to use them sparingly and only when necessary.