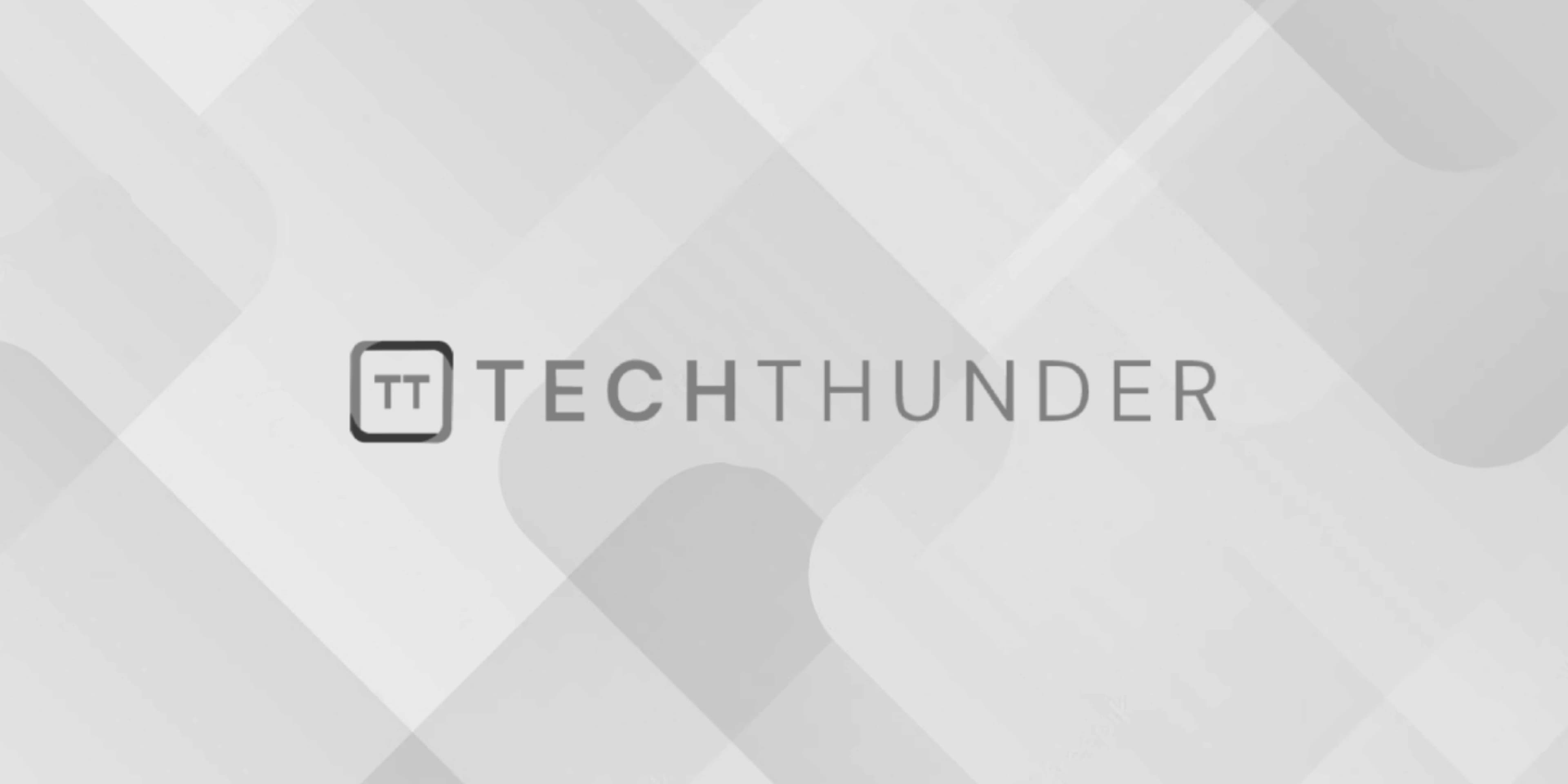
JavaScript Anonymous Functions
The JavaScript anonymous function is a function that is defined without a specified name. Instead of providing a name for the function, it is defined directly as an expression. Anonymous functions are commonly used in situations where a function is needed for a specific task, but it doesn’t need to be referenced or reused elsewhere in the code.
Here’s an example of an anonymous function:
// Anonymous function assigned to a variable
const greet = function() {
console.log("Hello, world!");
};
// Invoking the anonymous function
greet();
In this example, we define an anonymous function using the function
keyword without specifying a name. We assign the function to a variable called greet
. The function body simply logs the message “Hello, world!” to the console.
We can then invoke the anonymous function by calling the variable greet
as a function (greet()
). This will execute the function body and log the message to the console.
Anonymous functions can also accept parameters, just like named functions. Here’s an example:
// Anonymous function with parameters
const add = function(a, b) {
return a + b;
};
// Invoking the anonymous function
const result = add(5, 3);
console.log(result); // Output: 8
In this example, the anonymous function add
takes two parameters a
and b
and returns their sum. We can invoke the function by passing arguments to it (add(5, 3)
), and the result is stored in the result
variable. Finally, we log the result to the console.
Anonymous functions are commonly used in JavaScript for various purposes, such as event handlers, callbacks, and immediate function invocations. They provide a way to define and use functions inline without the need for a named function declaration.