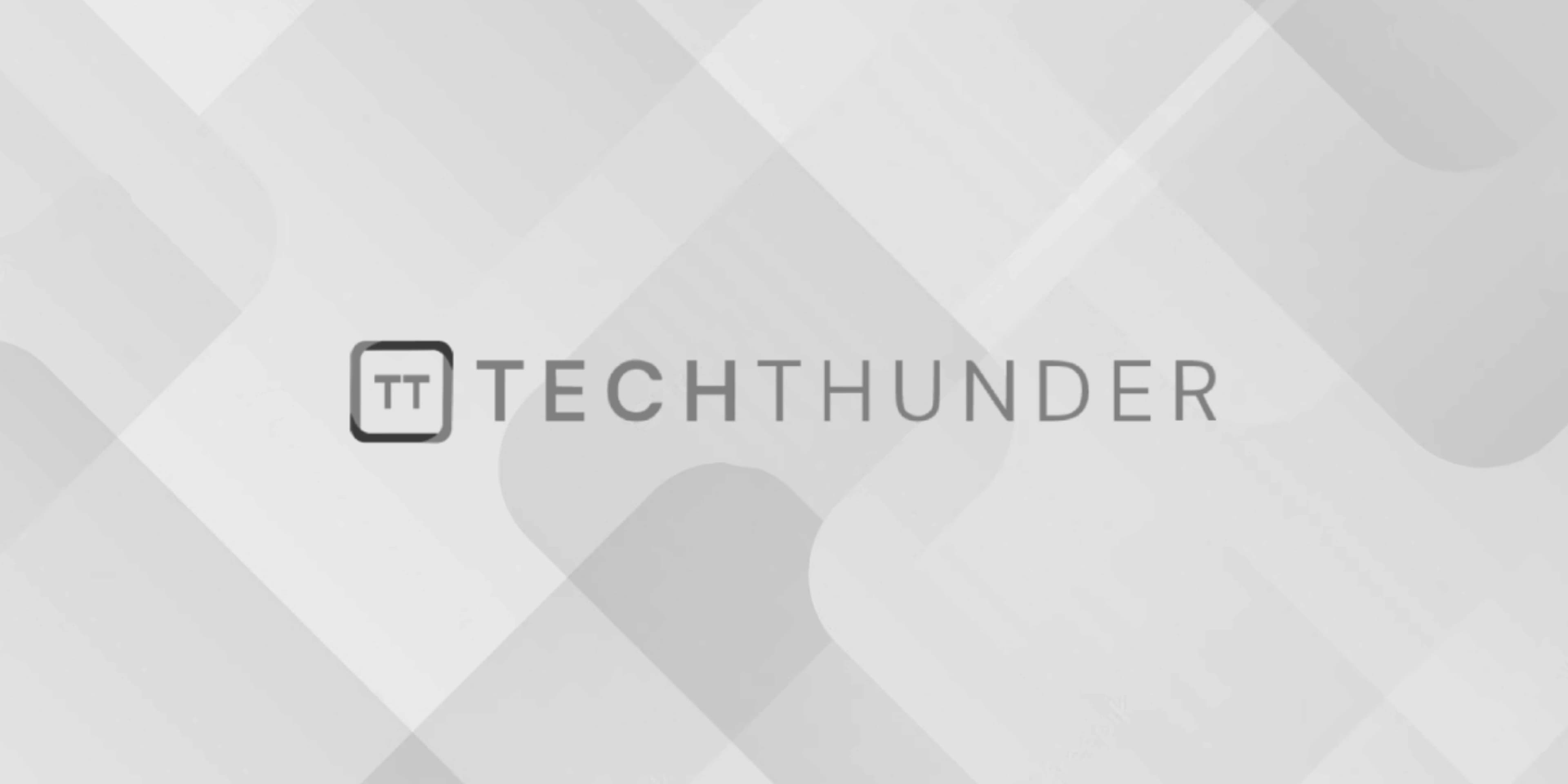
JavaScript First Class Function
The JavaScript functions are first-class citizens, which means they can be treated like any other value. This concept is known as “first-class functions” or “first-class citizens.” Here are some characteristics of first-class functions in JavaScript:
- Assigning Functions to Variables:
You can assign a function to a variable just like any other value. Here’s an example:
const greet = function(name) {
console.log('Hello, ' + name + '!');
};
greet('John'); // Output: Hello, John!
In this example, the greet
variable is assigned a function that takes a name
parameter and logs a greeting message.
- Passing Functions as Arguments:
Functions can be passed as arguments to other functions. This allows you to pass behavior or logic as a parameter. Here’s an example:
function processFunction(fn) {
// Perform some processing
fn('Alice');
}
function greet(name) {
console.log('Hello, ' + name + '!');
}
processFunction(greet); // Output: Hello, Alice!
In this example, the processFunction
function takes a function fn
as an argument and invokes it with a provided argument ('Alice'
in this case).
- Returning Functions from Functions:
Functions can also be returned from other functions. This enables you to create higher-order functions, which are functions that either take other functions as arguments or return functions as results. Here’s an example:
function createGreeter() {
return function(name) {
console.log('Hello, ' + name + '!');
};
}
const greet = createGreeter();
greet('Bob'); // Output: Hello, Bob!
In this example, the createGreeter
function returns an inner function that can be invoked later. The returned function retains access to variables in its lexical scope, even after the outer function has completed.
- Storing Functions in Data Structures:
Functions can be stored in arrays, objects, or other data structures just like any other value. Here’s an example:
const functionArray = [
function(name) {
console.log('Hello, ' + name + '!');
},
function(name) {
console.log('Goodbye, ' + name + '!');
}
];
functionArray[0]('Jane'); // Output: Hello, Jane!
functionArray[1]('John'); // Output: Goodbye, John!
In this example, an array functionArray
stores two functions that can be accessed and invoked using array indexes.
These are some of the ways in which JavaScript treats functions as first-class citizens. First-class functions give JavaScript its powerful functional programming capabilities and allow for greater flexibility and composability in your code.