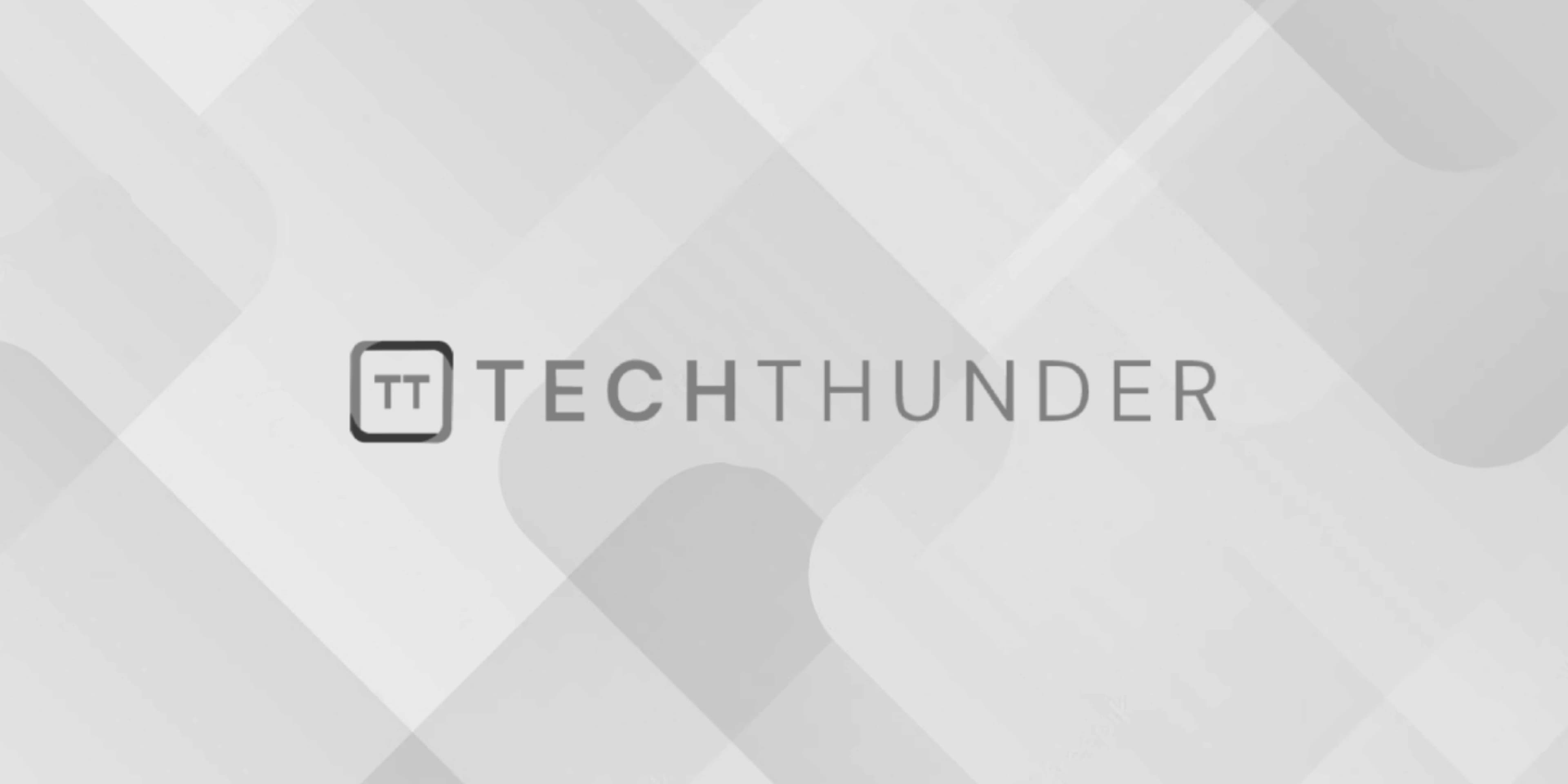
How to use Console in JavaScript
The JavaScript console
object provides methods for logging messages, debugging, and interacting with the browser console. Here are some commonly used methods of the console
object:
- console.log(): Logs messages to the console.
console.log('Hello, world!');
- console.error(): Logs error messages to the console.
console.error('An error occurred.');
- console.warn(): Logs warning messages to the console.
console.warn('This is a warning.');
- console.info(): Logs informational messages to the console.
console.info('This is an information.');
- console.debug(): Logs debug messages to the console. Note that the availability and behavior of this method may vary across different browsers.
console.debug('Debug information.');
- console.clear(): Clears the console.
console.clear();
- console.table(): Displays tabular data in the console.
var data = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 }
];
console.table(data);
- console.dir(): Displays an interactive listing of the properties of an object.
var obj = { name: 'John', age: 30 };
console.dir(obj);
These are just a few examples of the methods available on the console
object. The console is a powerful tool for debugging and logging information during JavaScript development. It provides a convenient way to output messages, inspect objects, and track the execution flow of your code.
You can open the browser’s developer console to view the logged messages and interact with the console output. Different browsers may have additional features and methods available on the console
object, so it’s recommended to refer to the browser’s documentation for specific details.