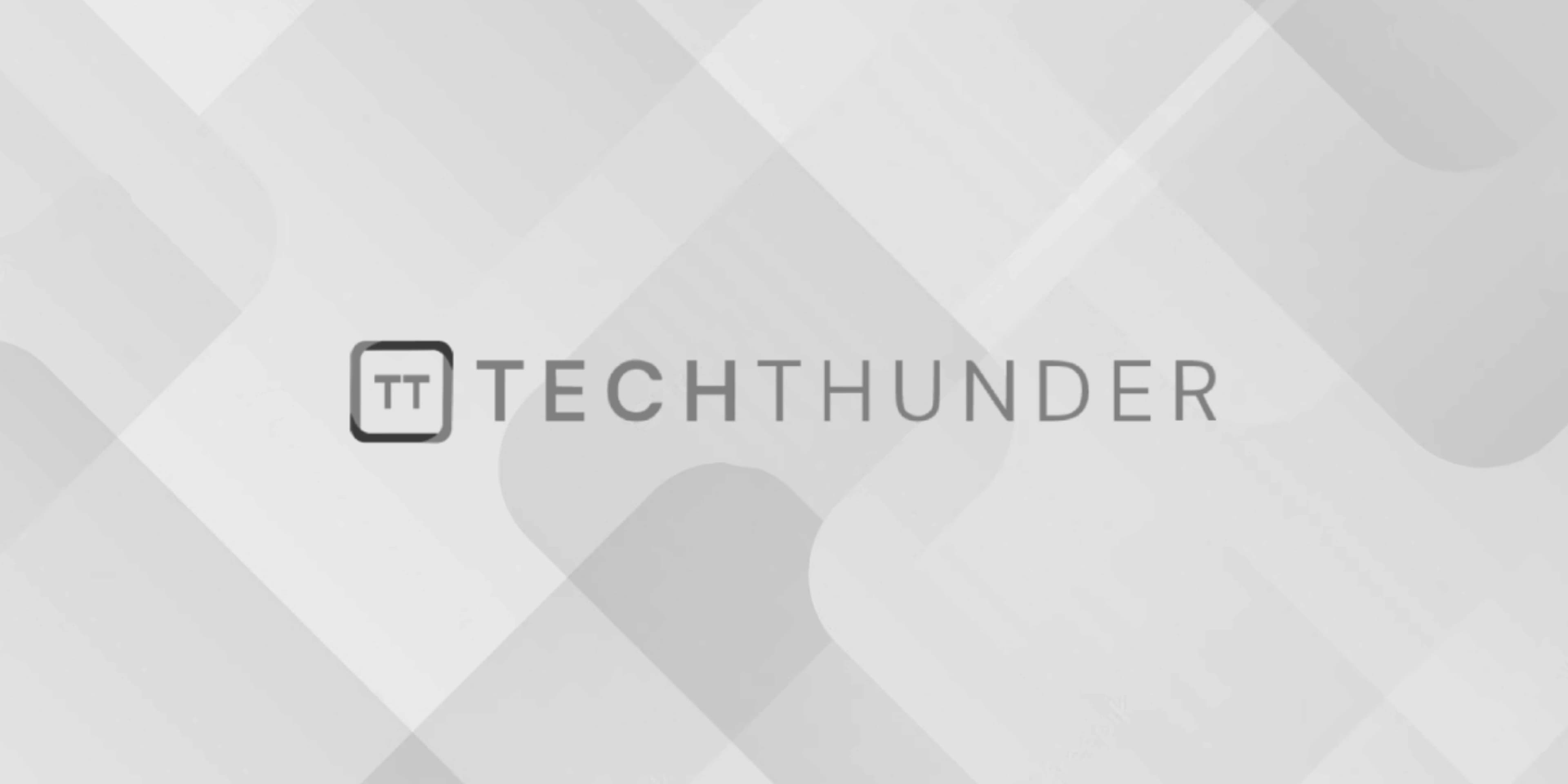
How to create dropdown list using JavaScript
To create a dropdown list using JavaScript, you can use the DOM (Document Object Model) manipulation methods to dynamically create the necessary HTML elements and append them to the desired container. Here’s an example of how you can create a dropdown list programmatically:
// Create an array of options
var options = ["Option 1", "Option 2", "Option 3", "Option 4"];
// Get the container element
var container = document.getElementById("dropdown-container");
// Create a select element
var select = document.createElement("select");
// Iterate over the options array and create option elements
for (var i = 0; i < options.length; i++) {
var option = document.createElement("option");
option.text = options[i];
option.value = options[i];
select.appendChild(option);
}
// Append the select element to the container
container.appendChild(select);
In this example, we first define an array options
that contains the list of options for the dropdown. Then, we get the container element using document.getElementById()
and create a select
element using document.createElement()
.
Next, we iterate over the options array using a for loop, create an option
element for each option, set its text
and value
attributes, and append it to the select
element using appendChild()
.
Finally, we append the select
element to the container using appendChild()
.
Make sure you have an HTML element with the specified id
(“dropdown-container” in this example) to serve as the container for the dropdown list.
This JavaScript code can be placed in a script tag within the HTML file or in an external JavaScript file and included in the HTML file using the script tag.