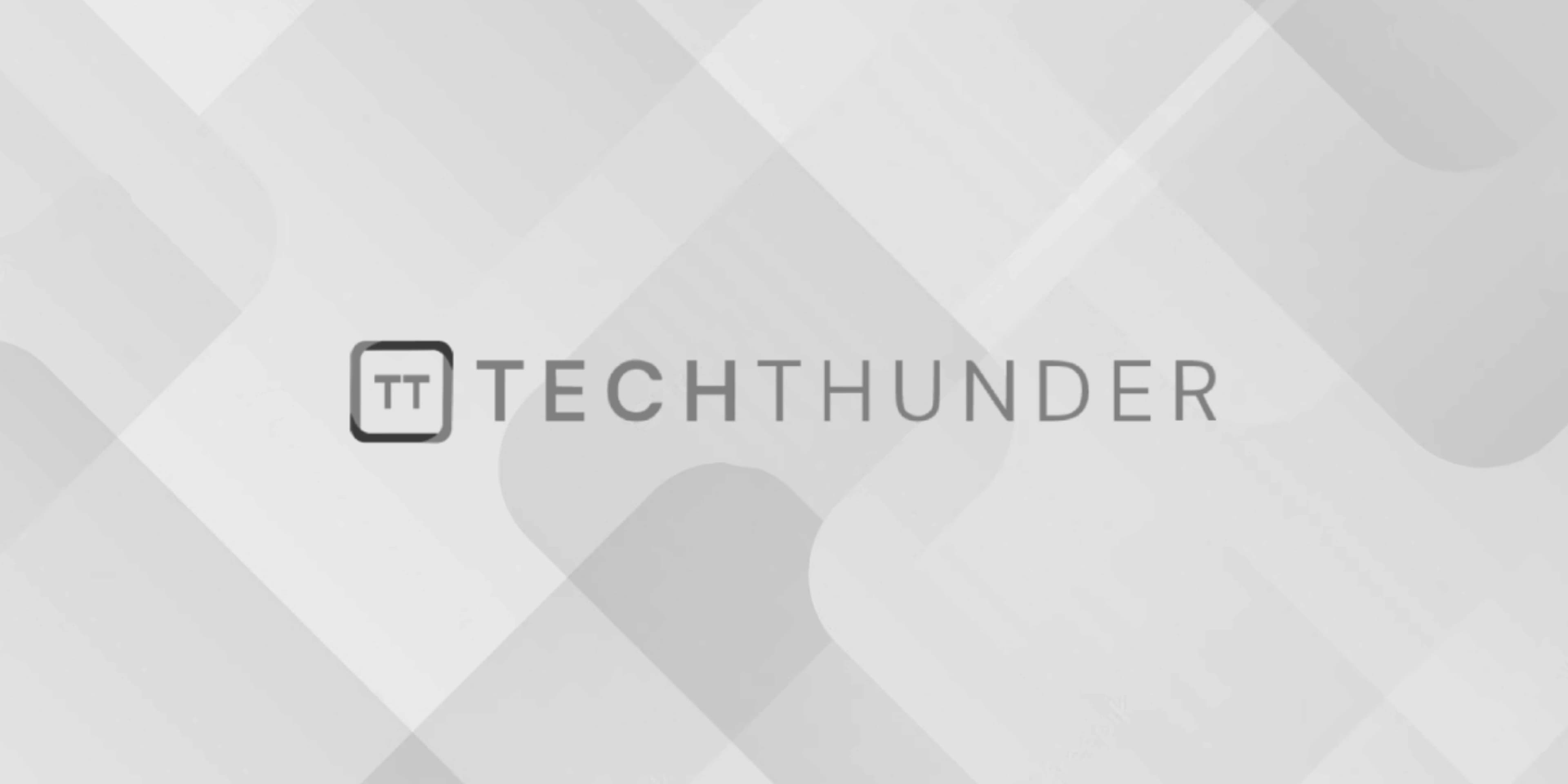
178 views
JavaScript Design Patterns
JavaScript design patterns are reusable solutions to common programming problems. They provide guidelines and best practices for structuring and organizing code in a way that promotes maintainability, scalability, and reusability. Here are some popular JavaScript design patterns:
- Module Pattern: The module pattern allows you to encapsulate private data and expose a public API. It uses closures to create private variables and functions, providing a way to achieve encapsulation and prevent naming collisions.
- Singleton Pattern: The singleton pattern restricts the instantiation of a class to a single instance. It ensures that only one instance of an object is created and provides a global access point to that instance.
- Observer Pattern: The observer pattern establishes a one-to-many dependency between objects. It allows multiple observers (subscribers) to be notified and updated automatically when the observed object (publisher) undergoes changes.
- Factory Pattern: The factory pattern provides an interface for creating objects but lets subclasses decide which class to instantiate. It abstracts the process of object creation, allowing flexibility and decoupling between the creator and the created objects.
- Prototype Pattern: The prototype pattern creates objects by cloning an existing object (prototype) rather than using a constructor function. It allows you to create new instances without explicitly defining a class or using a constructor.
- Decorator Pattern: The decorator pattern dynamically adds behaviors or responsibilities to objects without modifying their original implementation. It provides a flexible alternative to subclassing and allows you to extend object functionality at runtime.
- MVC Pattern: The Model-View-Controller (MVC) pattern separates an application into three interconnected components: the model (data and logic), the view (presentation layer), and the controller (handles user input and updates the model and view). It promotes separation of concerns and modularity.
- Promises Pattern: The promises pattern is a design pattern for handling asynchronous operations. It provides a way to represent and manage asynchronous computations, making code more readable and easier to reason about.
These are just a few examples of design patterns in JavaScript. Each pattern addresses specific concerns and can be applied in different scenarios based on the problem at hand. Understanding and applying design patterns can help you write more maintainable, modular, and reusable JavaScript code.