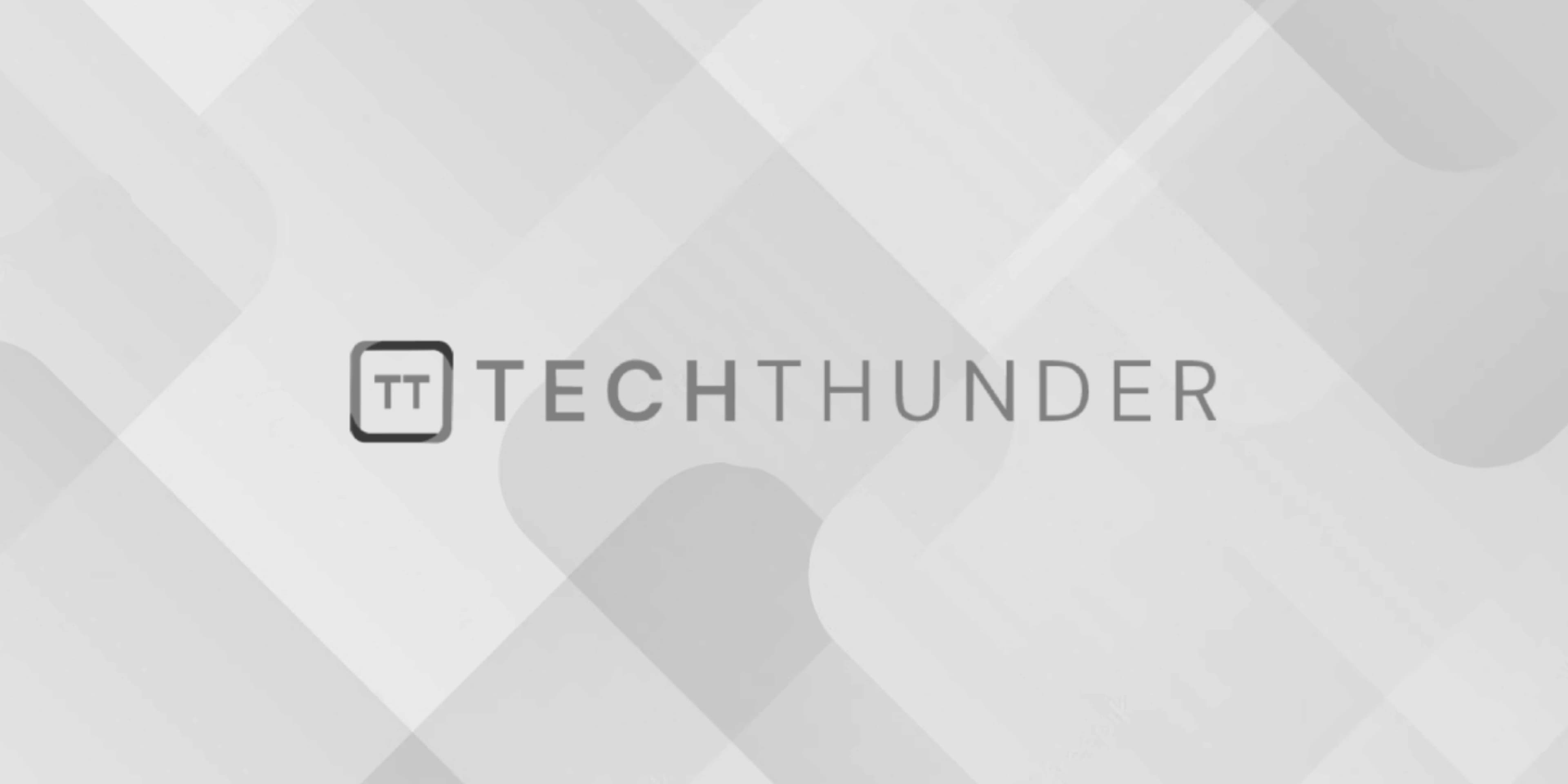
JavaScript closures
The JavaScript closure is a combination of a function and the lexical environment within which that function was declared. It allows a function to access variables and outer function scopes even after the outer function has finished executing.
Here’s an example to demonstrate closures in JavaScript:
function outer() {
var outerVar = 'I am from outer function';
function inner() {
var innerVar = 'I am from inner function';
console.log(outerVar + ' and ' + innerVar);
}
return inner;
}
var closureFn = outer();
closureFn(); // Output: "I am from outer function and I am from inner function"
In the above example, the outer
function defines an inner function inner
that has access to the outerVar
variable even after the outer
function has returned. This is possible because the inner function forms a closure over the variables in its outer function’s scope. The closureFn
variable stores a reference to the inner function, and when invoked, it still has access to the outerVar
variable.
Closures are useful in many scenarios, such as:
- Data privacy: Variables within a closure are not accessible from outside, providing a way to create private variables.
- Encapsulation: Closures can encapsulate data and behavior together, allowing you to create modules or reusable components.
- Function factories: Closures can be used to create functions with pre-configured settings or parameters.
- Asynchronous operations: Closures are commonly used to handle asynchronous operations, ensuring that the callback functions retain access to the relevant data.
Understanding closures is important for writing clean and efficient JavaScript code, especially in scenarios involving nested functions, callbacks, and maintaining data privacy.