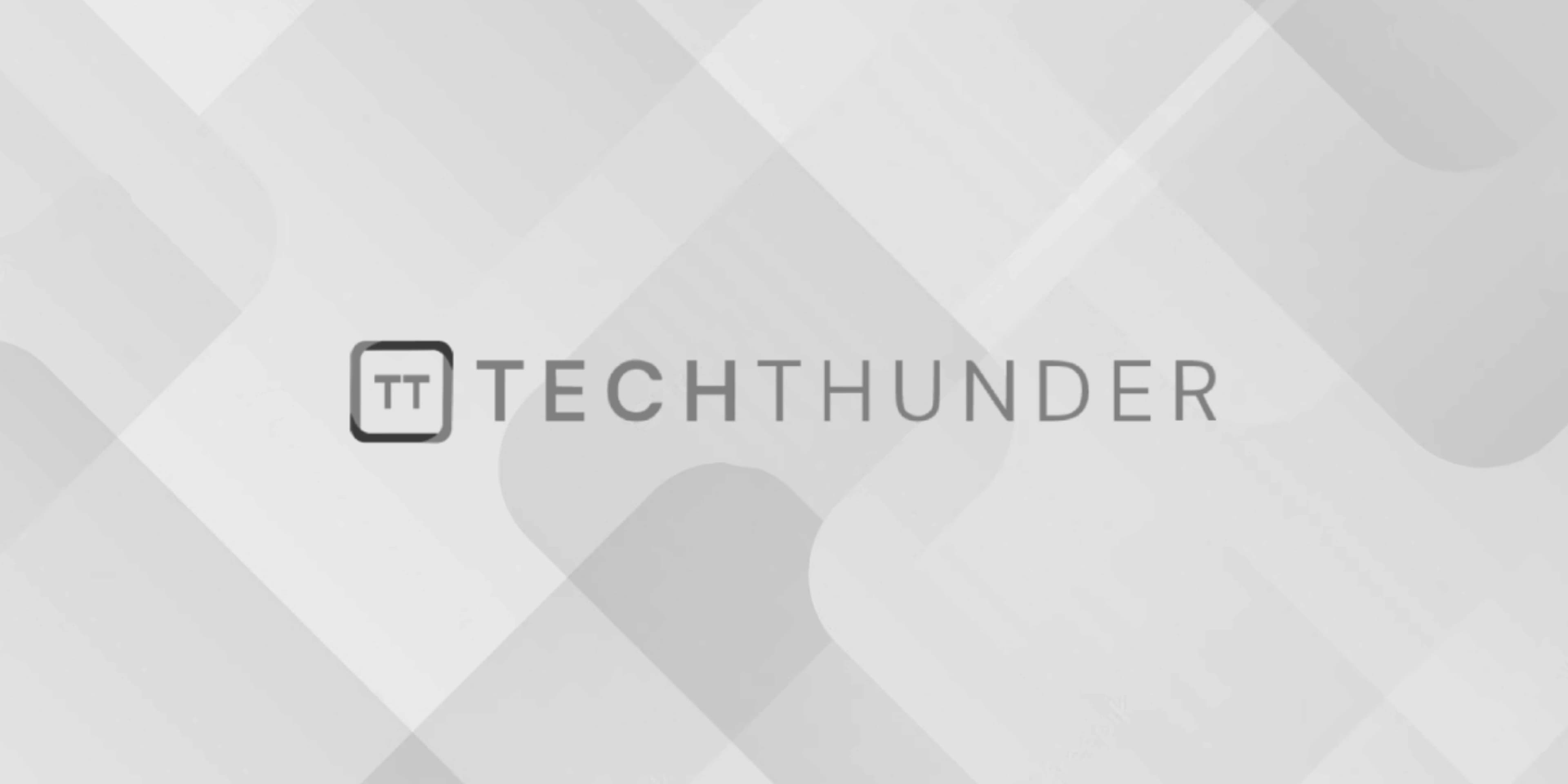
How to select all checkboxes using JavaScript
To select all checkboxes on a web page using JavaScript, you can iterate over all checkbox elements and set their checked
property to true
. Here’s an example:
// Get all checkbox elements
var checkboxes = document.querySelectorAll('input[type="checkbox"]');
// Loop through each checkbox and set checked to true
for (var i = 0; i < checkboxes.length; i++) {
checkboxes[i].checked = true;
}
In this example, document.querySelectorAll('input[type="checkbox"]')
selects all checkbox elements on the page using the CSS selector 'input[type="checkbox"]'
. The querySelectorAll
method returns a NodeList of matching elements.
Then, a for
loop is used to iterate over each checkbox element in the NodeList. The checked
property of each checkbox is set to true
to select it.
After executing this code, all checkboxes on the page will be checked.
Please note that this code assumes there are no nested checkbox elements within other elements. If you have nested checkboxes, you may need to adjust the code accordingly to target only the desired checkboxes.