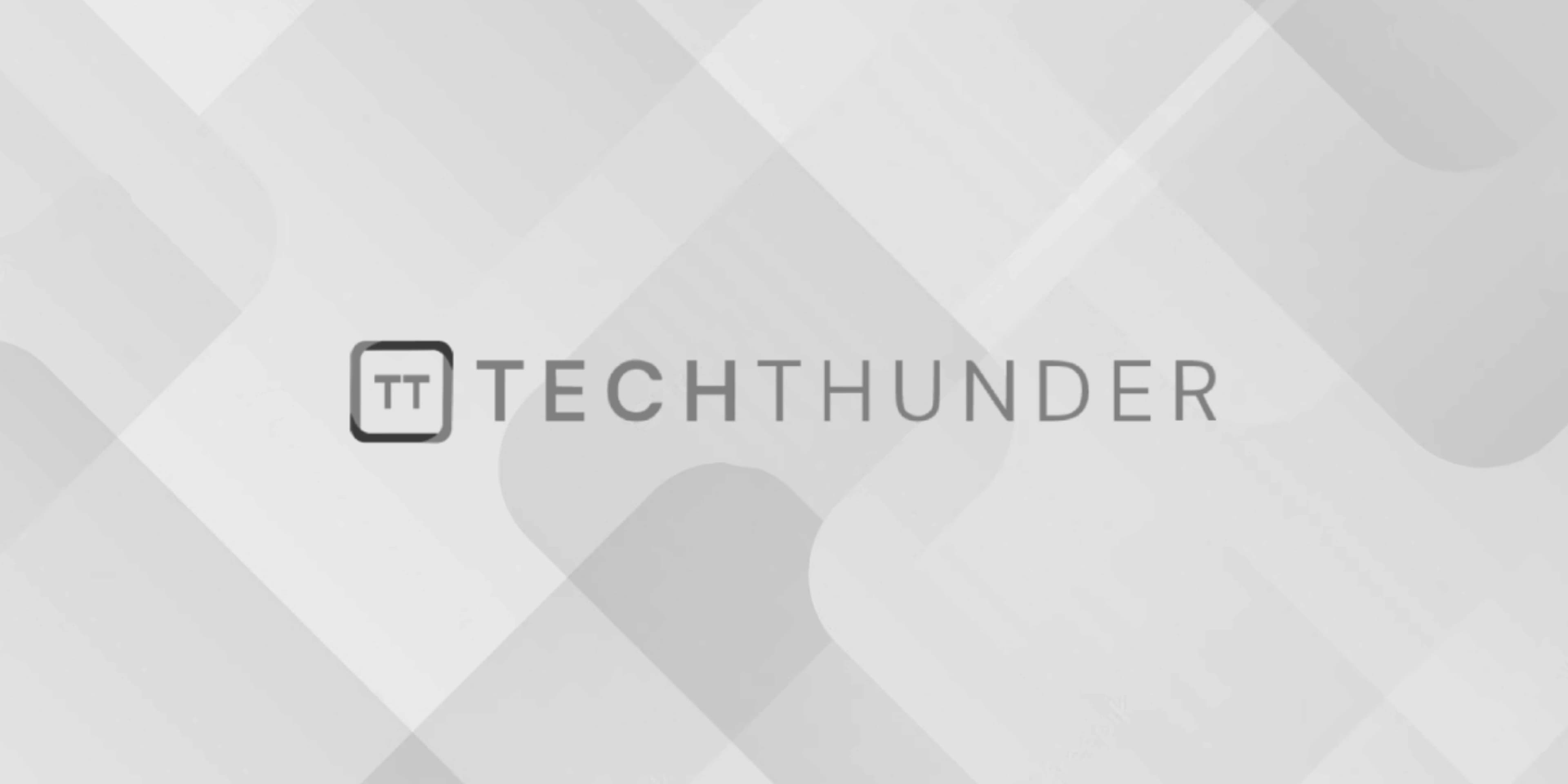
390 views
JavaScript String
The JavaScript String
is a sequence of characters that is used to represent text. You can create a String
using single quotes, double quotes, or backticks. Here are some examples:
JavaScript
let myString1 = 'Hello, world!';
let myString2 = "This is a string.";
let myString3 = `Another string.`;
The String
object provides many methods for working with strings. Here are some of the most commonly used ones:
length
: Returns the number of characters in a string.charAt(index)
: Returns the character at the specified index in a string.concat(str1, str2, ...)
: Combines two or more strings and returns the result.indexOf(searchValue, fromIndex)
: Returns the index of the first occurrence of a specified value in a string, starting from a specified index. Returns -1 if the value is not found.lastIndexOf(searchValue, fromIndex)
: Returns the index of the last occurrence of a specified value in a string, starting from a specified index. Returns -1 if the value is not found.slice(startIndex, endIndex)
: Extracts a section of a string and returns it as a new string.substring(startIndex, endIndex)
: Similar toslice()
, but does not accept negative indices.toLowerCase()
: Converts a string to lowercase.toUpperCase()
: Converts a string to uppercase.trim()
: Removes whitespace from both ends of a string.split(separator)
: Splits a string into an array of substrings based on a specified separator.
Here are some examples of using these methods:
JavaScript
let myString = 'Hello, world!';
console.log(myString.length); // Output: 13
console.log(myString.charAt(1)); // Output: "e"
let newString = myString.concat(' This is an additional text.');
console.log(newString); // Output: "Hello, world! This is an additional text."
console.log(myString.indexOf('o')); // Output: 4
console.log(myString.lastIndexOf('o')); // Output: 8
console.log(myString.slice(0, 5)); // Output: "Hello"
console.log(myString.substring(7, 12)); // Output: "world"
console.log(myString.toLowerCase()); // Output: "hello, world!"
console.log(myString.toUpperCase()); // Output: "HELLO, WORLD!"
let myStringWithSpaces = ' Hello, world! ';
console.log(myStringWithSpaces.trim()); // Output: "Hello, world!"
let myStringWithCommas = 'apple,orange,banana';
console.log(myStringWithCommas.split(',')); // Output: ["apple", "orange", "banana"]