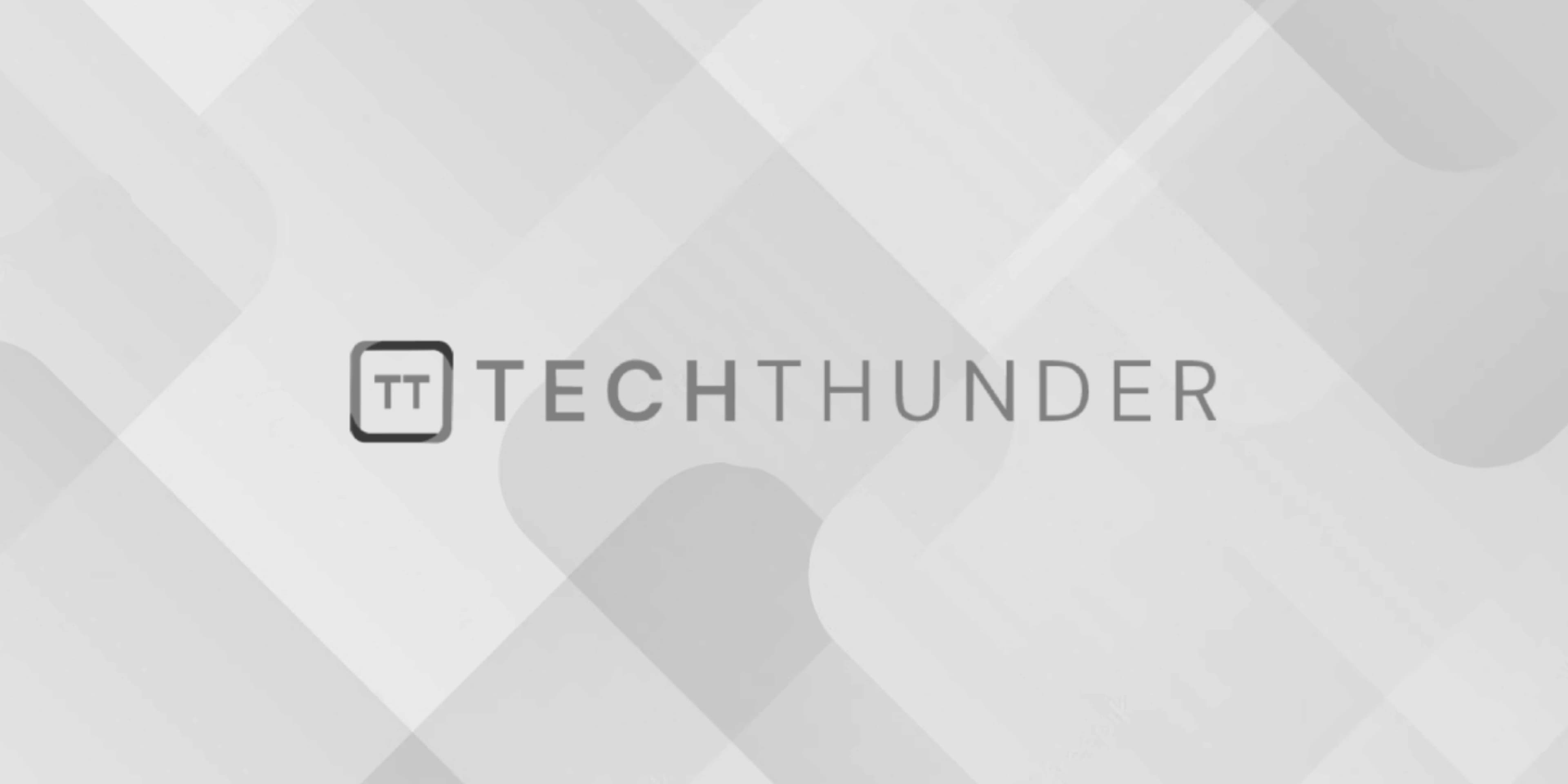
127 views
JavaScript NaN Function
The JavaScript NaN
(Not-a-Number) value is a special value that represents an invalid or unrepresentable numeric result. It is typically returned when a mathematical operation or function fails to produce a valid numeric result.
JavaScript provides several functions to check if a value is NaN
or perform operations related to NaN
. Here are a few commonly used functions:
isNaN(value)
: TheisNaN()
function checks if a value isNaN
. It returnstrue
if the value isNaN
, andfalse
otherwise. However,isNaN()
has a quirk: it coerces the value to a number before performing the check. This means that non-numeric values are first converted to a number and then checked if they areNaN
. This can lead to unexpected results, especially with non-numeric strings. To mitigate this, you can useNumber.isNaN()
(introduced in ES6) which performs a strictNaN
check without type coercion. Example:
JavaScript
isNaN(10); // Output: false
isNaN("hello"); // Output: true
isNaN("123"); // Output: false
Number.isNaN("hello"); // Output: false
Number.isNaN(NaN); // Output: true
isNaN()
vsNumber.isNaN()
: As mentioned earlier,isNaN()
performs type coercion before the check, whileNumber.isNaN()
does not. It is generally recommended to useNumber.isNaN()
when specifically checking forNaN
and useisNaN()
when you want to check if a value cannot be converted to a number.Object.is(value1, value2)
: TheObject.is()
function can also be used to check if a value isNaN
. It performs a strict equality comparison, which means it checks if the values are identical, includingNaN
. Example:
JavaScript
Object.is(NaN, NaN); // Output: true
Object.is(10, NaN); // Output: false
These functions can be helpful when working with numeric calculations and handling situations where NaN
values may occur. It’s important to use them appropriately based on your specific use case to ensure accurate results.