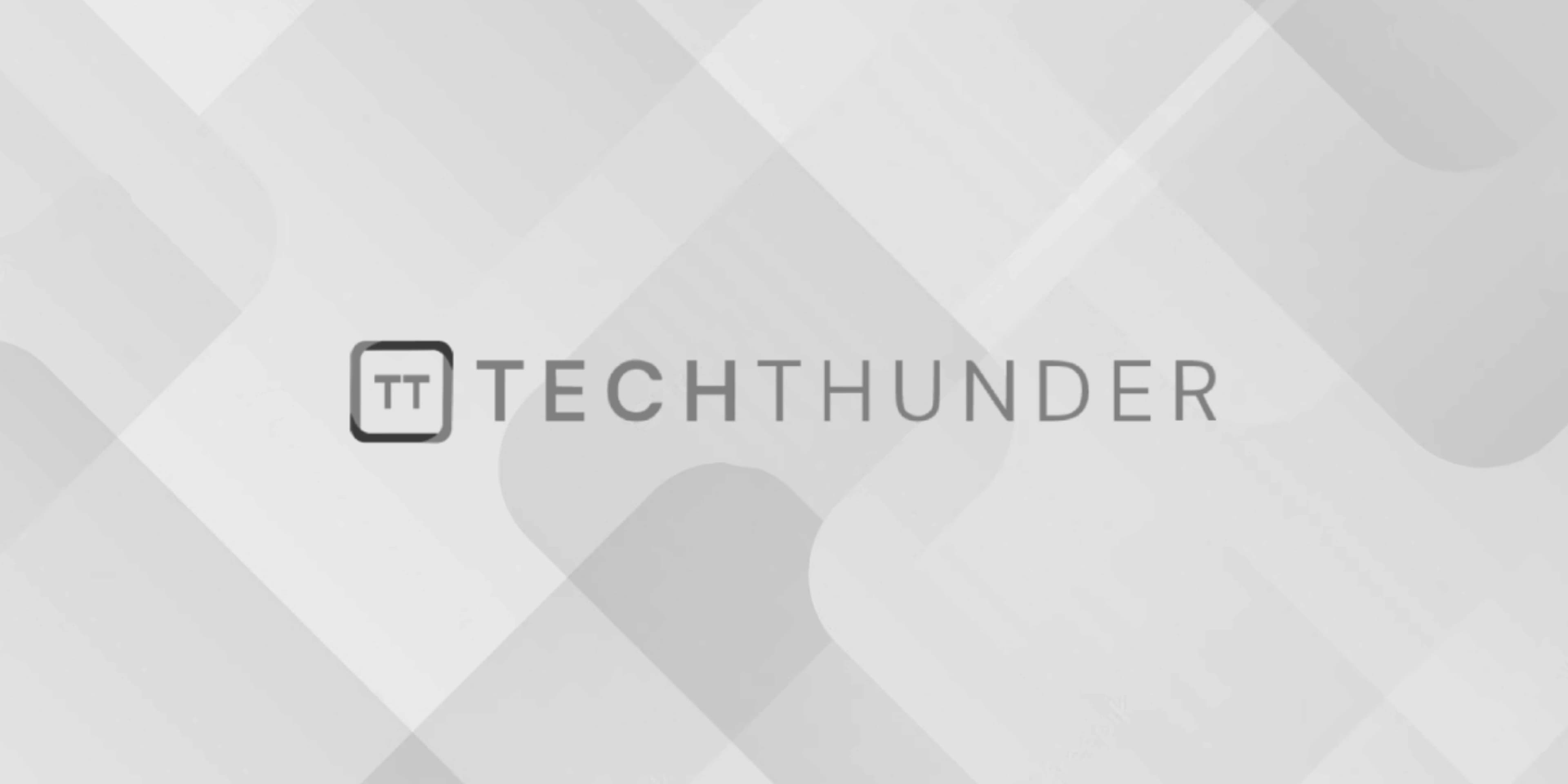
How to Check the Specific Element in the JavaScript Class
To check if a specific element exists within a JavaScript class or an HTML element with a specific class name, you can use various methods and properties provided by JavaScript. Here are a few approaches:
- Using
querySelector()
:
// Check if an element with class "myClass" exists within a specific container
const container = document.getElementById('container');
const elementExists = container.querySelector('.myClass') !== null;
console.log(elementExists); // Output: true or false
In this example, querySelector('.myClass')
selects the first element with the class “myClass” that is a descendant of the container element. If such an element exists, it returns the element; otherwise, it returns null
.
- Using
getElementsByClassName()
:
// Check if an element with class "myClass" exists in the entire document
const elements = document.getElementsByClassName('myClass');
const elementExists = elements.length > 0;
console.log(elementExists); // Output: true or false
Here, getElementsByClassName('myClass')
returns a collection of elements with the class “myClass”. We can check if the length of the collection is greater than 0 to determine if the element exists.
- Using
classList.contains()
:
// Check if a specific element has the class "myClass"
const element = document.getElementById('myElement');
const hasClass = element.classList.contains('myClass');
console.log(hasClass); // Output: true or false
In this example, classList.contains('myClass')
checks if the specified element has the class “myClass” by returning true
if it does and false
otherwise.
These methods can be adapted to fit your specific use case and provide flexibility in checking for the existence of elements with specific classes within JavaScript classes or HTML elements.