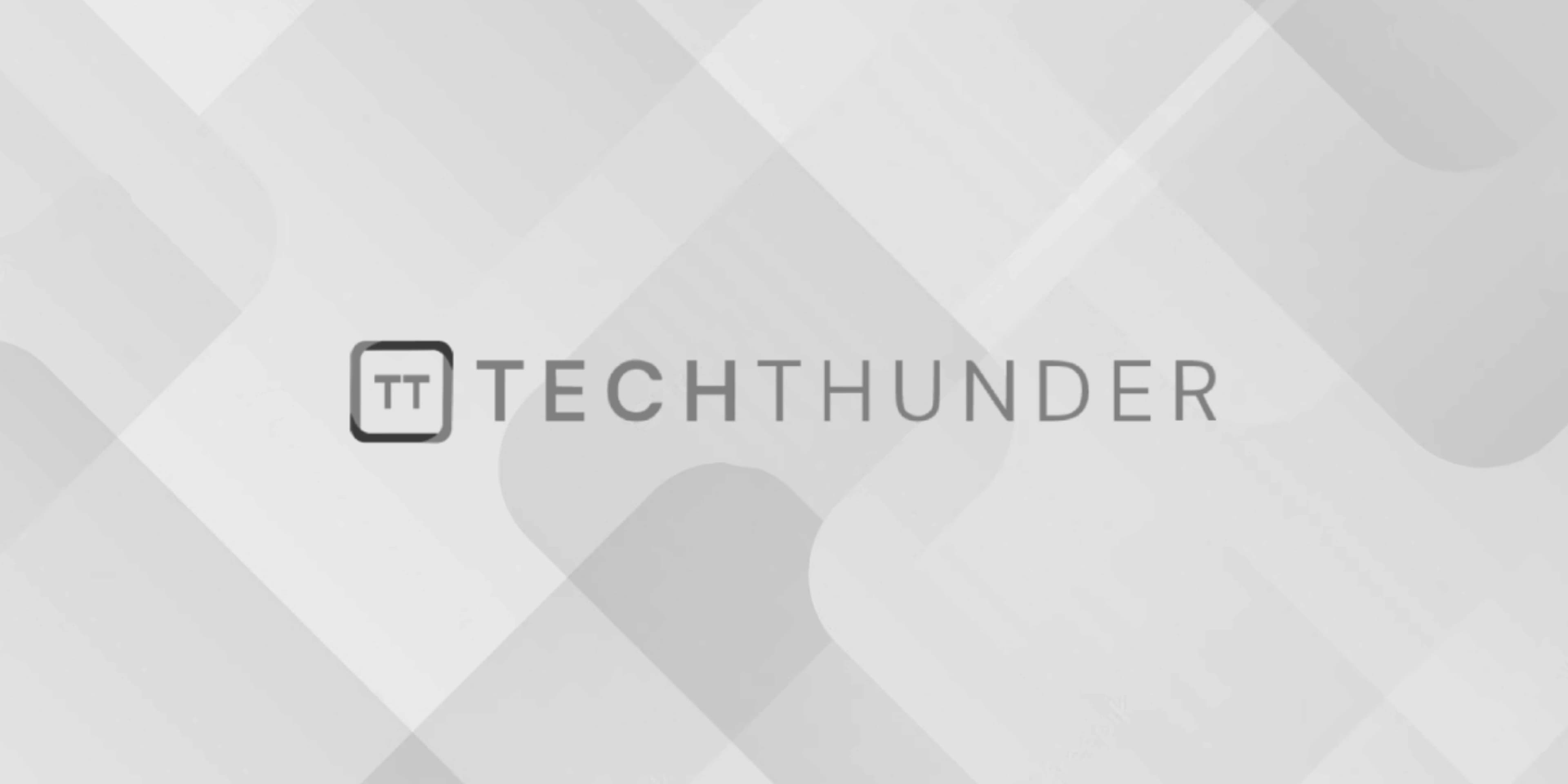
JavaScript TypedArray
The JavaScript Typed Arrays are a group of array-like objects that provide a way to store and manipulate binary data in a structured manner. They are part of the JavaScript standard since ECMAScript 2015 (ES6) and are used for handling raw binary data more efficiently.
There are several types of Typed Arrays available in JavaScript:
Int8Array
,Uint8Array
,Uint8ClampedArray
: Represents an array of 8-bit signed integers, 8-bit unsigned integers, and 8-bit unsigned integers clamped to 0-255 range, respectively.Int16Array
,Uint16Array
: Represents an array of 16-bit signed integers and 16-bit unsigned integers, respectively.Int32Array
,Uint32Array
,Float32Array
,Float64Array
: Represents an array of 32-bit signed integers, 32-bit unsigned integers, 32-bit floating-point numbers, and 64-bit floating-point numbers, respectively.
These Typed Arrays provide various methods and properties for accessing and manipulating the binary data. Here’s an example of creating and working with a Typed Array:
// Create a new Uint8Array with 4 elements
const array = new Uint8Array([10, 20, 30, 40]);
// Accessing values
console.log(array[0]); // Output: 10
// Modifying values
array[1] = 50;
console.log(array); // Output: Uint8Array [10, 50, 30, 40]
// Looping through the array
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
// Using array methods
const sum = array.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // Output: 130
Typed Arrays provide a more efficient and performant way to work with binary data compared to regular JavaScript arrays. They are commonly used in scenarios such as networking, file I/O, graphics processing, and other low-level operations where precise control over binary data is required.