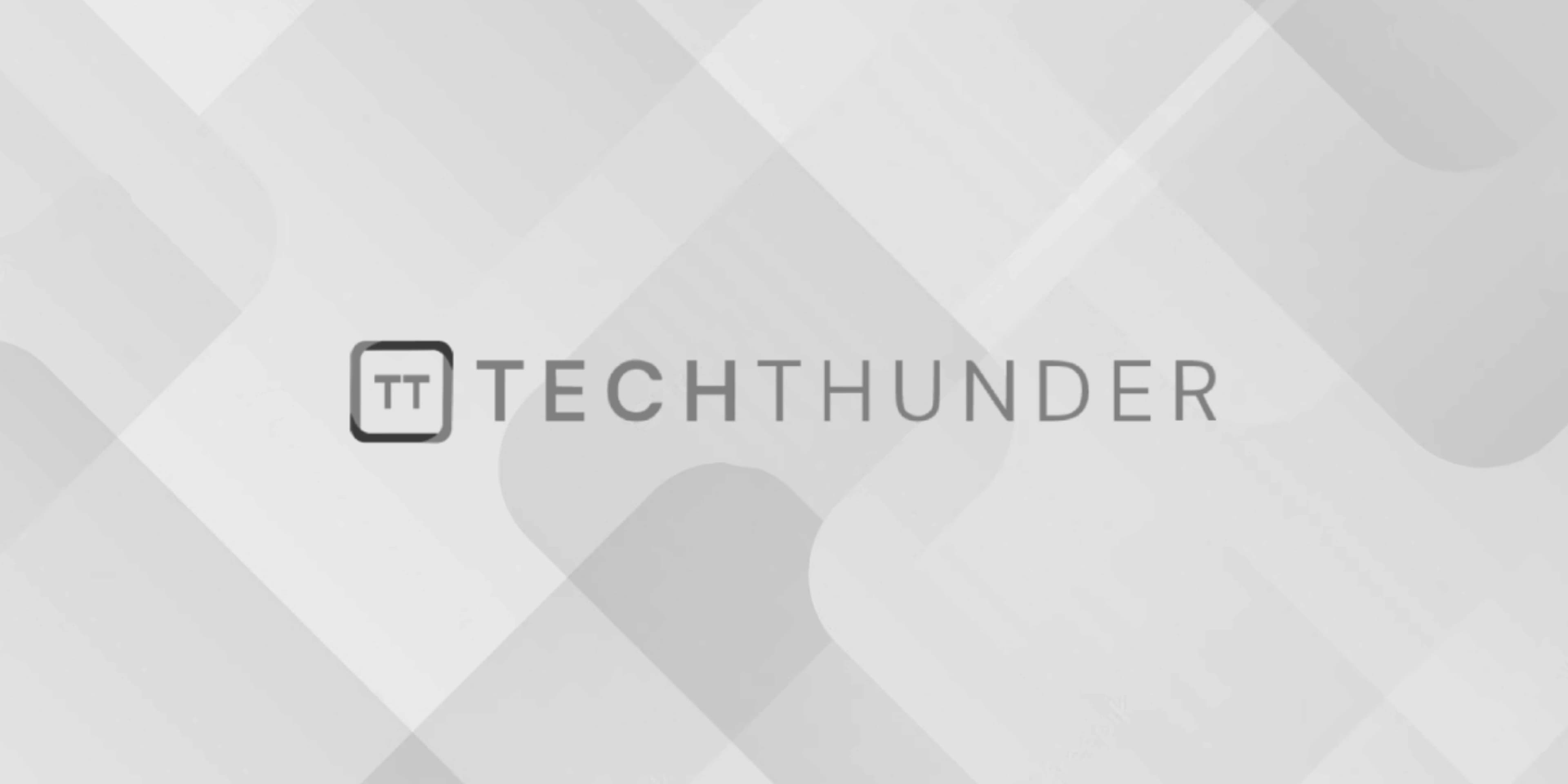
JavaScript indexedDB
IndexedDB is a JavaScript API for storing and retrieving large amounts of structured data, including files and blobs, within the browser. It provides a way to create client-side databases that can be queried using indexes.
Here are the basic steps to work with IndexedDB:
- Open a connection to the IndexedDB database using the
indexedDB.open()
method. Provide the name of the database and the version number.
var request = indexedDB.open('myDatabase', 1);
request.onerror = function(event) {
// Handle error
};
request.onsuccess = function(event) {
var db = event.target.result;
// Continue with database operations
};
- In the
onsuccess
event handler, you can access the database instance throughevent.target.result
. Store it in a variable (db
in the example above) for further operations. - Set up the database schema by defining object stores and indexes within the database. Object stores are like tables in a relational database, and indexes allow efficient retrieval of data based on certain properties.
request.onupgradeneeded = function(event) {
var db = event.target.result;
// Create an object store
var objectStore = db.createObjectStore('myObjectStore', { keyPath: 'id' });
// Create an index
objectStore.createIndex('nameIndex', 'name', { unique: false });
};
In the onupgradeneeded
event handler, you can define the structure of the database, including creating object stores and indexes. This event is triggered when the database is first created or when its version number is incremented.
- Perform database operations such as adding, retrieving, updating, or deleting data.
var transaction = db.transaction('myObjectStore', 'readwrite');
var objectStore = transaction.objectStore('myObjectStore');
// Add data
objectStore.add({ id: 1, name: 'John Doe', age: 25 });
// Retrieve data
var request = objectStore.get(1);
request.onsuccess = function(event) {
var data = event.target.result;
console.log(data);
};
// Update data
var updateRequest = objectStore.put({ id: 1, name: 'Jane Smith', age: 30 });
// Delete data
var deleteRequest = objectStore.delete(1);
In the above code, we first create a transaction using the transaction()
method on the database instance. Then, we access an object store within the transaction using the objectStore()
method.
We can perform various operations on the object store, such as adding data using add()
, retrieving data using get()
, updating data using put()
, and deleting data using delete()
. The results of these operations are handled through the onsuccess
and onerror
event handlers.
IndexedDB also supports more advanced features like querying with indexes, working with cursors, and handling transactions. For more detailed information on working with IndexedDB, you can refer to the official documentation or other online resources.