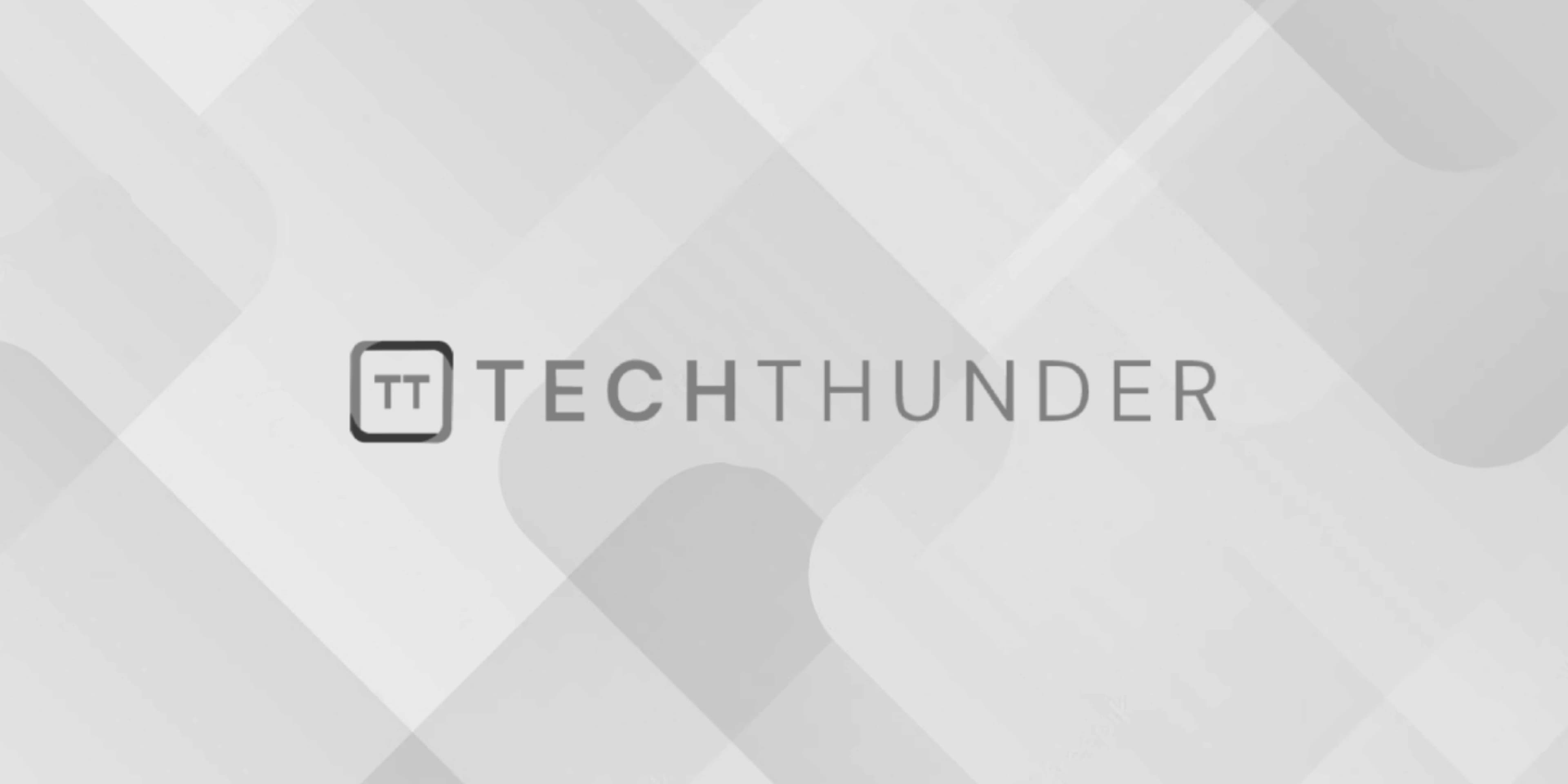
Insert data into javascript indexedDB
To insert data into JavaScript IndexedDB, you need to perform the following steps:
- Open a connection to the IndexedDB database using the
indexedDB.open()
method. Provide the name of the database and the version number.
var request = indexedDB.open('myDatabase', 1);
request.onerror = function(event) {
// Handle error
};
request.onsuccess = function(event) {
var db = event.target.result;
// Continue with database operations
};
- In the
onsuccess
event handler, you can access the database instance throughevent.target.result
. Store it in a variable (db
in the example above) for further operations. - Create a transaction to access an object store within the database. Use the
db.transaction()
method, providing the name of the object store and the desired access mode (read/write).
var transaction = db.transaction('myObjectStore', 'readwrite');
- Access the object store within the transaction using the
transaction.objectStore()
method.
var objectStore = transaction.objectStore('myObjectStore');
- Use the
objectStore.add()
orobjectStore.put()
method to insert or update data in the object store, respectively. Provide the data you want to insert/update as the first parameter.
var data = { id: 1, name: 'John Doe', age: 25 };
var request = objectStore.add(data);
request.onsuccess = function(event) {
console.log('Data inserted successfully');
};
request.onerror = function(event) {
console.log('Error inserting data');
};
In the above code, we create an object data
with the properties we want to insert into the object store. We then call objectStore.add(data)
to insert the data into the object store. You can use objectStore.put(data)
instead if you want to update an existing record with the same key.
The onsuccess
and onerror
event handlers of the request object are used to handle the success or failure of the operation.
By following these steps, you can insert data into a JavaScript IndexedDB object store. Remember to handle errors appropriately by assigning an onerror
event handler to the request and transaction objects to catch any potential errors that may occur during the operation.