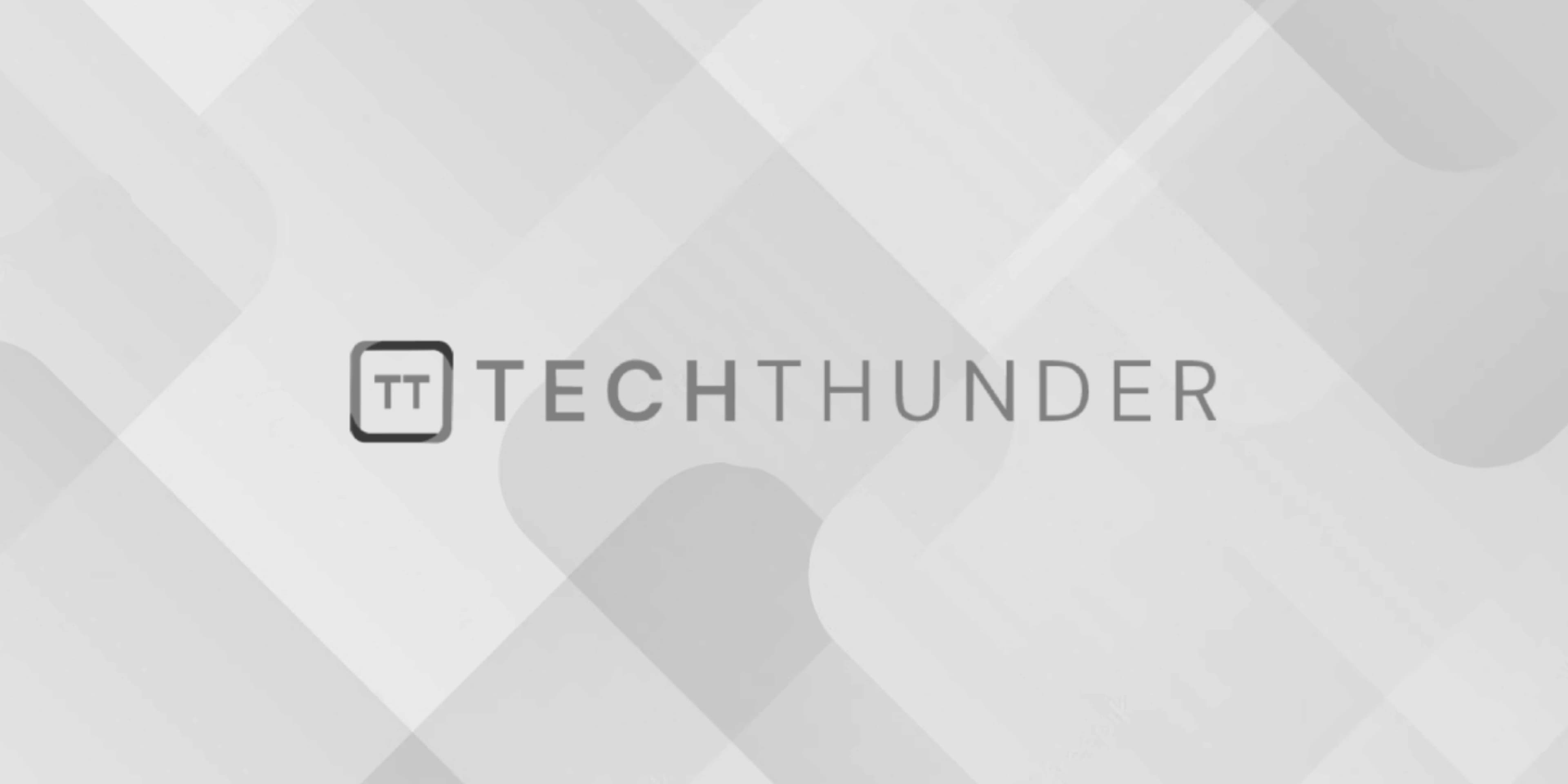
JavaScript removeChild
To remove a specific element from the DOM (Document Object Model) using JavaScript, you can make use of the removeChild()
method. Here’s an example:
<div id="parent">
<p>This is the parent element.</p>
<p id="child">This is the child element.</p>
</div>
<button onclick="removeChildElement()">Remove Child Element</button>
<script>
function removeChildElement() {
var parentElement = document.getElementById("parent");
var childElement = document.getElementById("child");
// Remove the child element from the parent
parentElement.removeChild(childElement);
}
</script>
In the above example, we have a parent div
element with an id
of “parent” and a child p
element with an id
of “child”. When the button is clicked, it triggers the removeChildElement()
function.
Inside the function, we first obtain references to both the parent and child elements using getElementById()
. Then, we call the removeChild()
method on the parent element, passing the child element as the argument. This removes the child element from the parent element, effectively removing it from the DOM.
By executing this code, the child element (with the id
of “child”) will be removed from the parent element, resulting in the disappearance of the corresponding paragraph from the rendered HTML.
Note that removeChild()
requires the parent element to remove the child element. It’s essential to ensure you have a reference to both the parent and child elements before attempting to remove the child element.