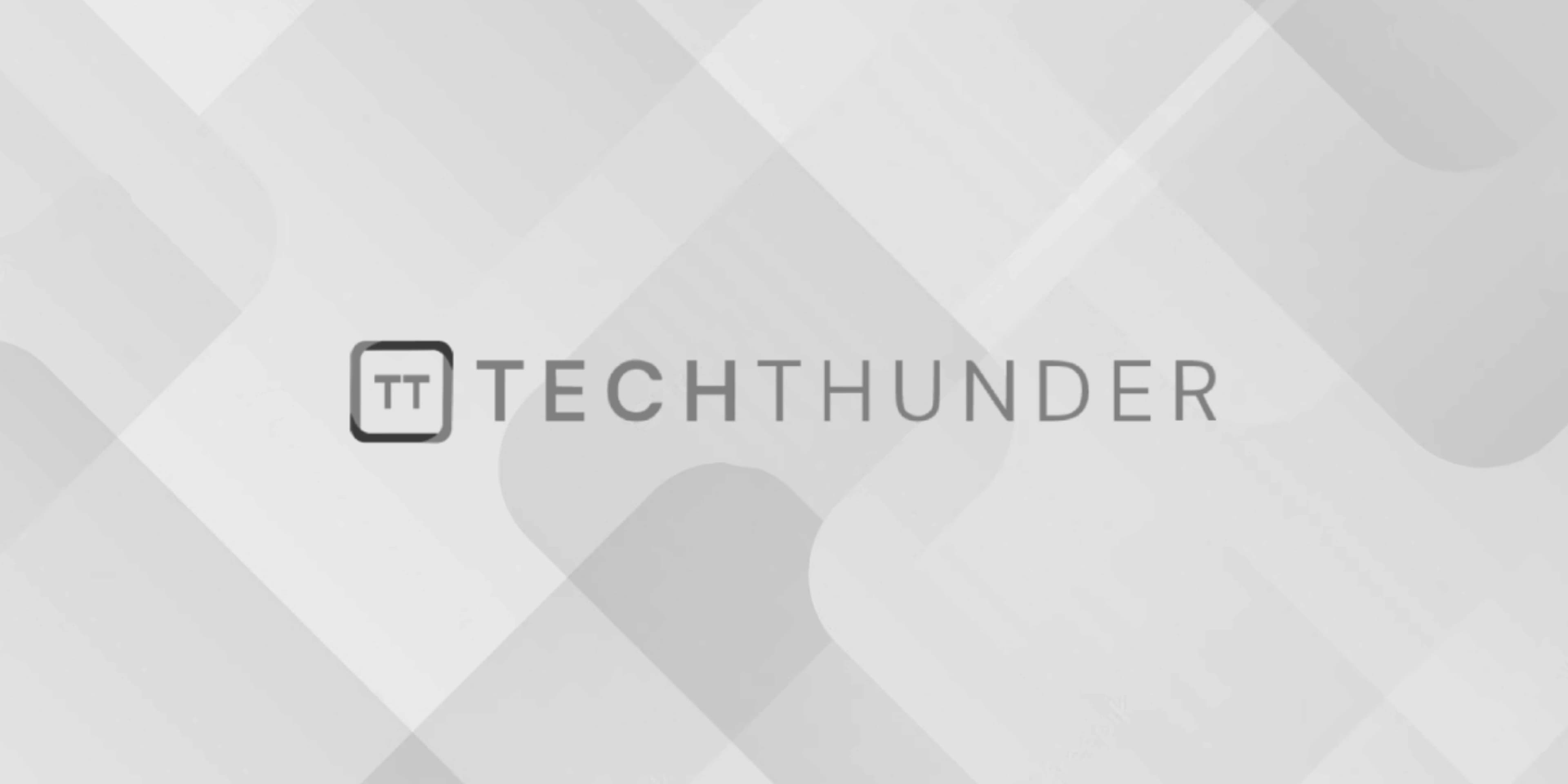
116 views
JavaScript Comparison
The JavaScript compare values using comparison operators to determine their relationship or equality. Here are the commonly used comparison operators in JavaScript:
- Equal to (
==
): Checks if two values are equal, performing type coercion if necessary. For example:
JavaScript
console.log(5 == 5); // true
console.log('5' == 5); // true (coerced to the same value)
console.log(5 == '5'); // true (coerced to the same value)
- Not equal to (
!=
): Checks if two values are not equal, performing type coercion if necessary. For example:
JavaScript
console.log(5 != 3); // true
console.log('5' != 5); // false (coerced to the same value)
console.log(5 != '5'); // false (coerced to the same value)
- Strict equal to (
===
): Checks if two values are equal without performing type coercion. It compares both the value and the type. For example:
JavaScript
console.log(5 === 5); // true
console.log('5' === 5); // false (different types)
console.log(5 === '5'); // false (different types)
- Strict not equal to (
!==
): Checks if two values are not equal without performing type coercion. It compares both the value and the type. For example:
JavaScript
console.log(5 !== 3); // true
console.log('5' !== 5); // true (different types)
console.log(5 !== '5'); // true (different types)
- Greater than (
>
): Checks if the left operand is greater than the right operand. For example:
JavaScript
console.log(5 > 3); // true
console.log(5 > 10); // false
- Less than (
<
): Checks if the left operand is less than the right operand. For example:
JavaScript
console.log(5 < 10); // true
console.log(5 < 3); // false
- Greater than or equal to (
>=
): Checks if the left operand is greater than or equal to the right operand. For example:
JavaScript
console.log(5 >= 3); // true
console.log(5 >= 5); // true
console.log(5 >= 10); // false
- Less than or equal to (
<=
): Checks if the left operand is less than or equal to the right operand. For example:
JavaScript
console.log(5 <= 10); // true
console.log(5 <= 5); // true
console.log(5 <= 3); // false
These comparison operators return a boolean value (true
or false
) based on the comparison result. Keep in mind the differences between ==
and ===
, as well as !=
and !==
, which involve type coercion.
Additionally, you can use logical operators (&&
, ||
, !
) to combine or negate comparison expressions for more complex conditions.