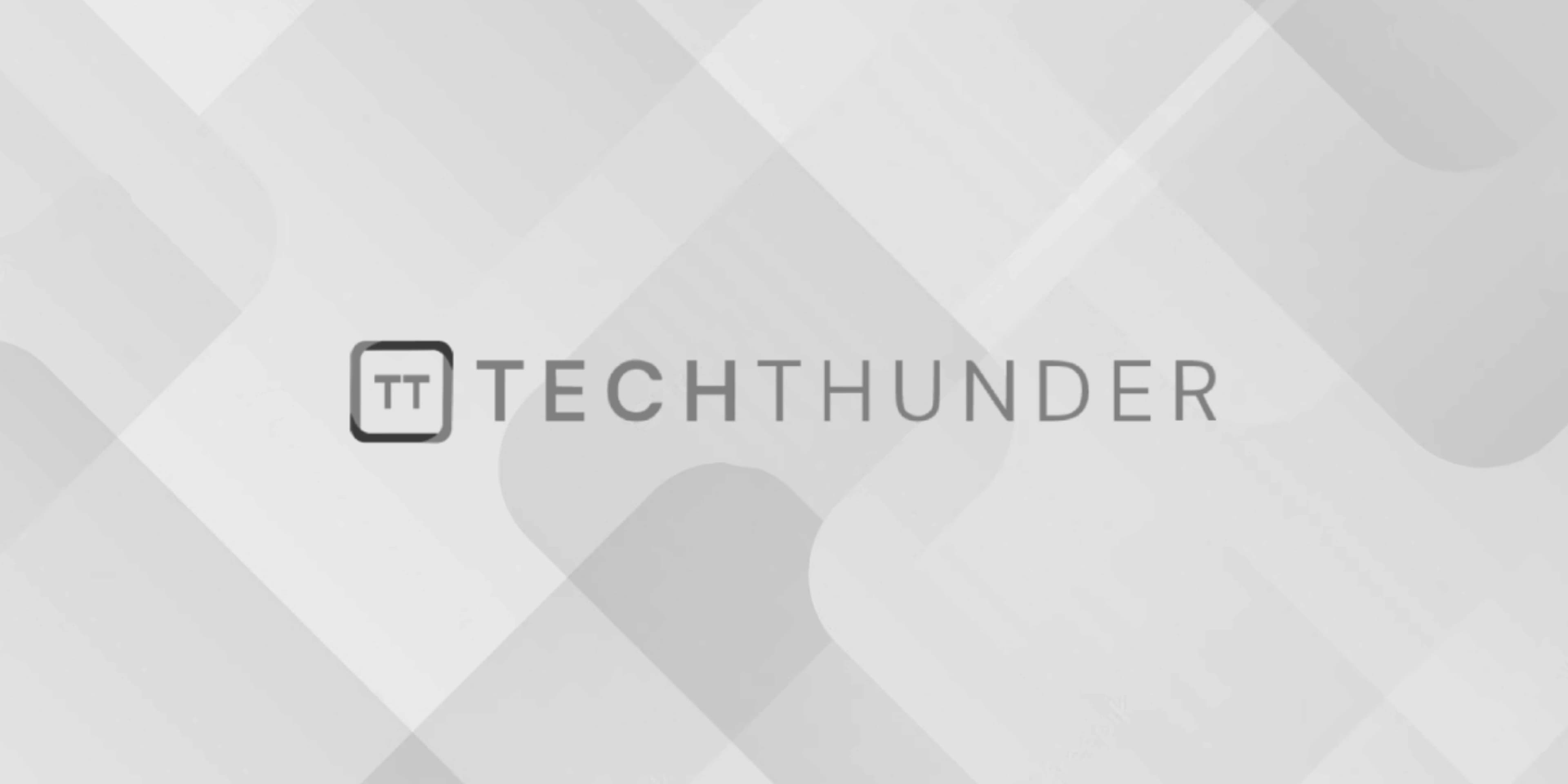
Event Bubbling and Capturing in JavaScript
Event bubbling and capturing are two mechanisms in JavaScript that describe how events propagate through the DOM (Document Object Model) tree.
Event capturing occurs in the “capturing phase” and starts from the outermost element (e.g., the <html>
element) and travels down to the target element. It allows you to capture an event on an ancestor element before it reaches the target element.
Event bubbling, on the other hand, occurs in the “bubbling phase” and starts from the target element and travels up through its ancestors until it reaches the outermost element. It allows you to handle an event on the target element and then propagate the event to its parent elements.
By default, most events in JavaScript follow the bubbling phase. When an event occurs on an element, it triggers the event handlers attached to that element, and then the event “bubbles up” through the DOM tree, triggering event handlers on each ancestor element unless the event propagation is explicitly stopped.
You can control whether an event uses capturing or bubbling by using the addEventListener
method and passing a third parameter, useCapture
. By default, useCapture
is set to false
, enabling the event to use the bubbling phase. If you set useCapture
to true
, the event will use the capturing phase.
Here’s an example that demonstrates event capturing and bubbling:
<div id="outer">
<div id="inner">
Click me
</div>
</div>
function handleEvent(event) {
console.log("Event type: " + event.type + ", Target element: " + event.target.id);
}
var outerElement = document.getElementById("outer");
var innerElement = document.getElementById("inner");
outerElement.addEventListener("click", handleEvent, true); // Capturing phase
innerElement.addEventListener("click", handleEvent); // Bubbling phase
In this example, we have an outer <div>
element with the ID “outer” and an inner <div>
element with the ID “inner”.
We attach event listeners to both elements using the addEventListener
method. The event listener for the outer element is set to use the capturing phase by passing true
as the third parameter. The event listener for the inner element uses the default bubbling phase.
When you click on the inner element, the event will first trigger the capturing phase event handler attached to the outer element, and then it will trigger the bubbling phase event handler attached to the inner element. The event will propagate from the outer element to the inner element and then back up to the outer element.
In the event handlers, we log the event type and the ID of the target element to the console for demonstration purposes.
By understanding event capturing and bubbling, you can handle events at different phases of propagation and control how they propagate through the DOM tree.