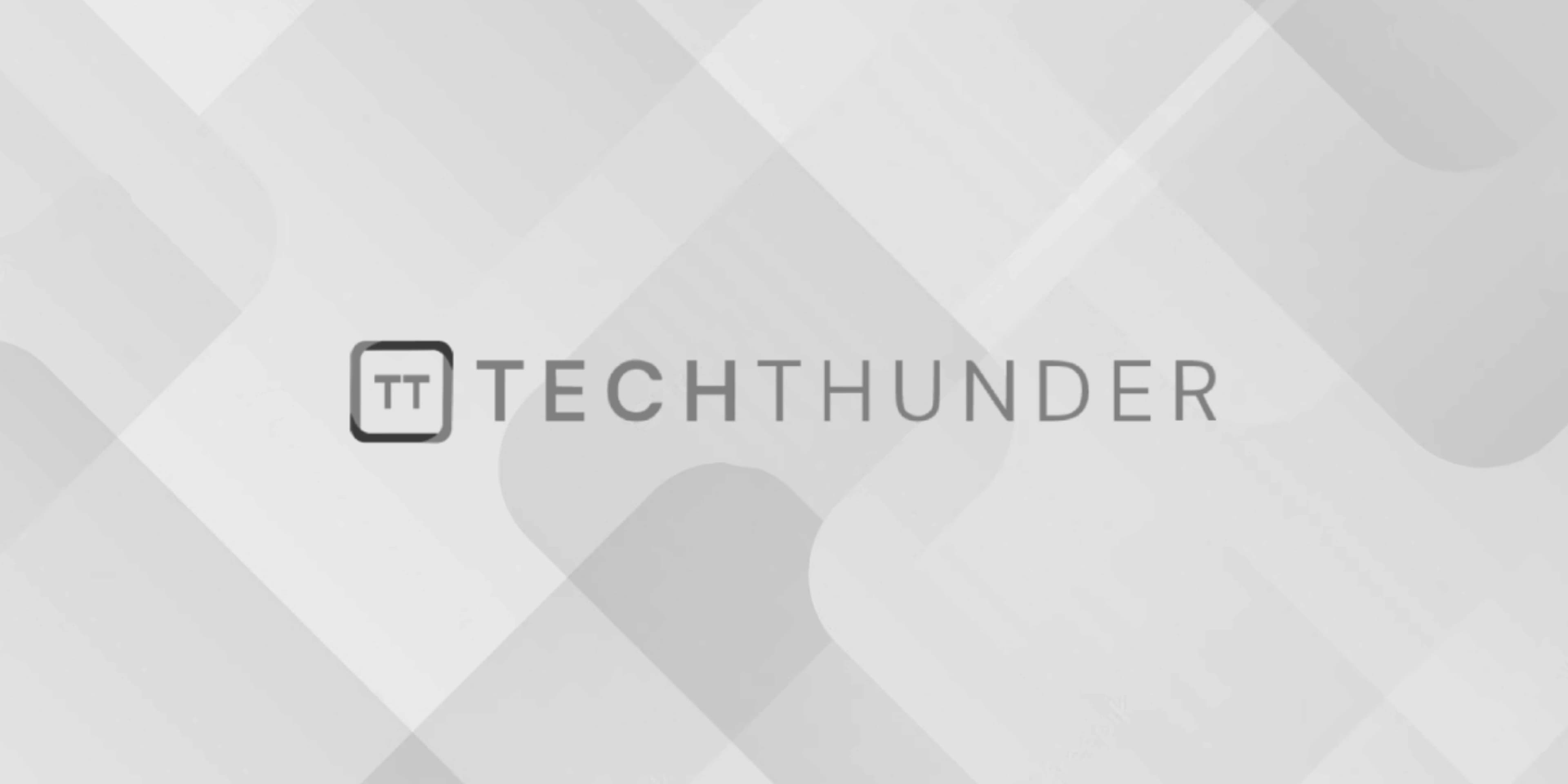
How to open JSON file
To open a JSON file using JavaScript, you can use the XMLHttpRequest object or the fetch API to make an HTTP request to retrieve the JSON file’s contents. Here’s an example using the fetch API:
fetch('path/to/file.json')
.then(response => response.json())
.then(data => {
// Use the data from the JSON file
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
In this example, replace 'path/to/file.json'
with the actual path or URL of your JSON file.
The fetch()
function is used to initiate an HTTP request to fetch the JSON file. It returns a Promise that resolves to the response of the request.
The first .then()
block converts the response to JSON format using the json()
method, which also returns a Promise that resolves to the JSON data.
In the second .then()
block, you can work with the JSON data retrieved from the file. In the example, it logs the data to the console. You can perform further processing or utilize the data as needed within this block.
If any errors occur during the fetch or JSON parsing process, the .catch()
block will catch and handle the error.
By executing this code, the JSON file will be fetched, and the contents will be available in the data
variable within the second .then()
block. You can then perform various operations or extract specific information from the JSON data.