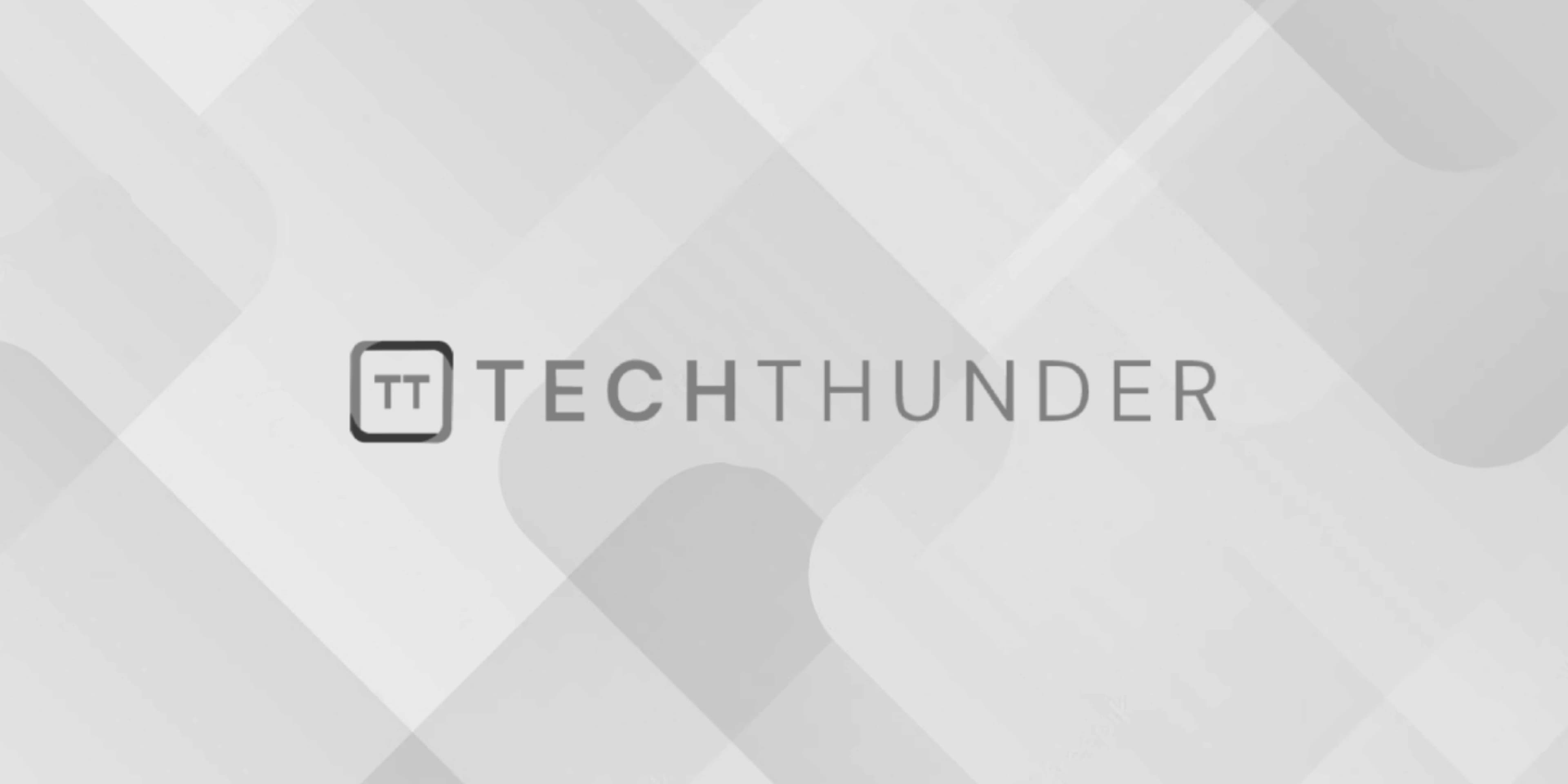
Random String Generator using JavaScript
To generate a random string using JavaScript, you can combine various techniques such as generating random characters or numbers and concatenating them together. Here’s an example of a random string generator:
function generateRandomString(length) {
var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
var result = '';
for (var i = 0; i < length; i++) {
var randomIndex = Math.floor(Math.random() * characters.length);
result += characters.charAt(randomIndex);
}
return result;
}
// Example usage: generate a random string of length 8
var randomString = generateRandomString(8);
console.log(randomString);
In this example, the generateRandomString
function takes a length
parameter, which specifies the desired length of the random string to be generated.
Inside the function, we define a string of characters that can be used to construct the random string. In this case, we use a combination of uppercase letters, lowercase letters, and digits.
A result
variable is initialized as an empty string, which will store the generated random string.
A for loop is used to iterate length
times. In each iteration, a random index is generated using Math.random()
multiplied by the length of the characters
string. The Math.floor()
function is used to round the random index down to an integer value.
The character at the random index is retrieved using charAt()
and appended to the result
string.
After the loop finishes, the result
string contains the generated random string, which is then returned by the function.
To generate a random string, you can call the generateRandomString
function with the desired length as an argument. In the example usage, we generate a random string of length 8 and log it to the console.
Executing this code will generate and display a random string of the specified length each time it is run.