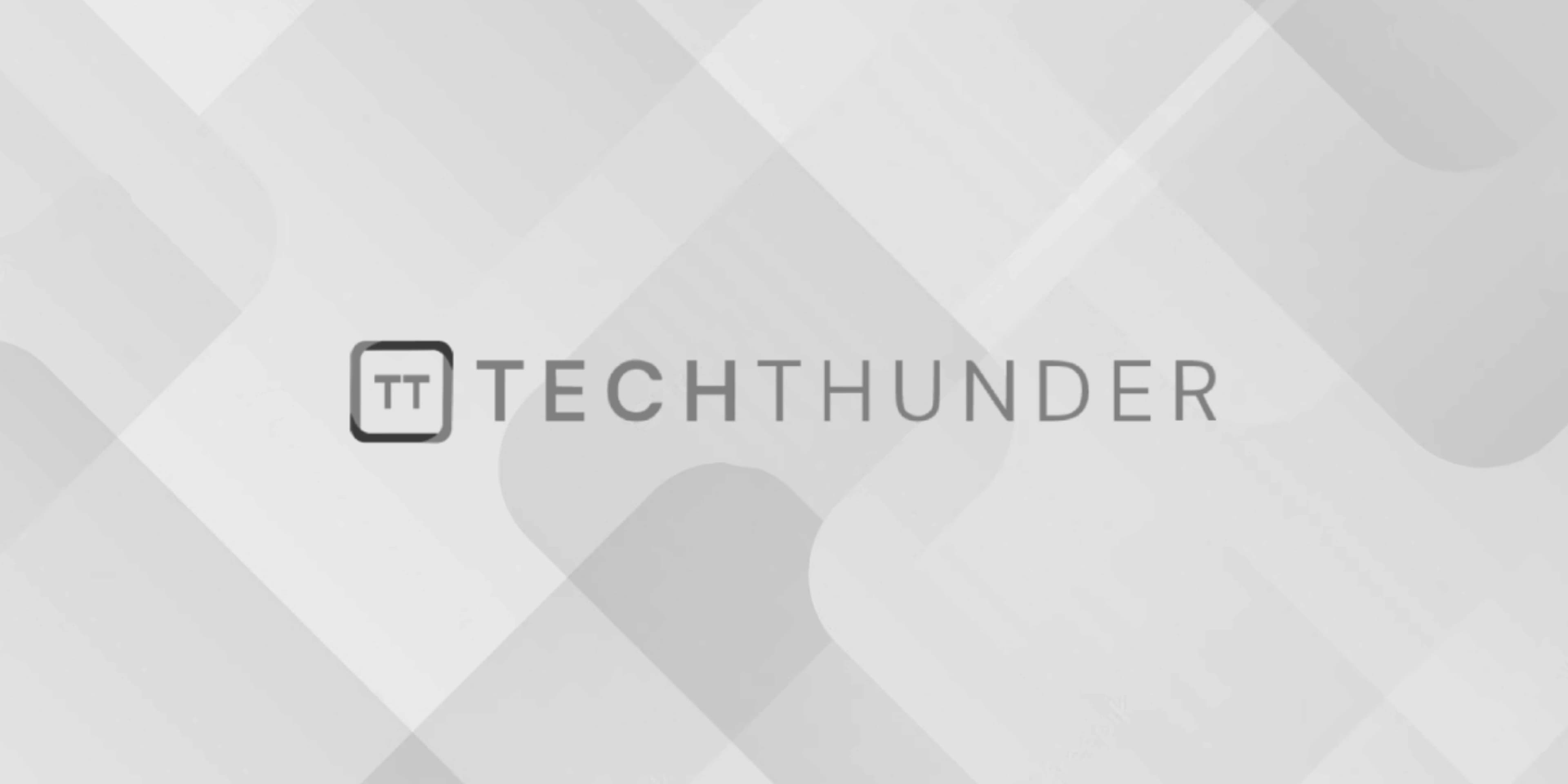
JavaScript Date
The JavaScript Date
object is used to work with dates and times. The Date
object can be used to create a new date object or manipulate an existing one. Here are some examples:
// create a new date object
let currentDate = new Date();
// create a new date object with a specific date and time
let specificDate = new Date(2023, 4, 12, 12, 30, 0);
// manipulate an existing date object
currentDate.setFullYear(2023);
currentDate.setMonth(4);
currentDate.setDate(12);
currentDate.setHours(12);
currentDate.setMinutes(30);
currentDate.setSeconds(0);
// convert a date object to a string
console.log(currentDate.toString());
// Output: "Fri May 12 2023 12:30:00 GMT+0100 (British Summer Time)"
The Date
object provides several methods for getting and setting date and time components. Here are some of the most commonly used ones:
getDate()
: Returns the day of the month (1-31) for the specified date object.getMonth()
: Returns the month (0-11) for the specified date object. Note that January is 0 and December is 11.getFullYear()
: Returns the year (four digits) for the specified date object.getHours()
: Returns the hour (0-23) for the specified date object.getMinutes()
: Returns the minutes (0-59) for the specified date object.getSeconds()
: Returns the seconds (0-59) for the specified date object.getTime()
: Returns the number of milliseconds since January 1, 1970, 00:00:00 UTC for the specified date object.
You can also perform arithmetic operations on Date
objects to calculate time intervals between them, or to add or subtract time from a given date. Here’s an example:
// create two date objects
let startDate = new Date(2023, 0, 1);
let endDate = new Date(2023, 11, 31);
// calculate the number of days between the two dates
let timeDiff = endDate.getTime() - startDate.getTime();
let daysDiff = timeDiff / (1000 * 3600 * 24);
console.log(daysDiff); // Output: 364
This code calculates the number of days between January 1, 2023, and December 31, 2023. The getTime()
method is used to get the number of milliseconds since January 1, 1970, for each date object, and the difference between them is calculated in milliseconds. The result is then divided by the number of milliseconds in a day to get the number of days between the two dates.