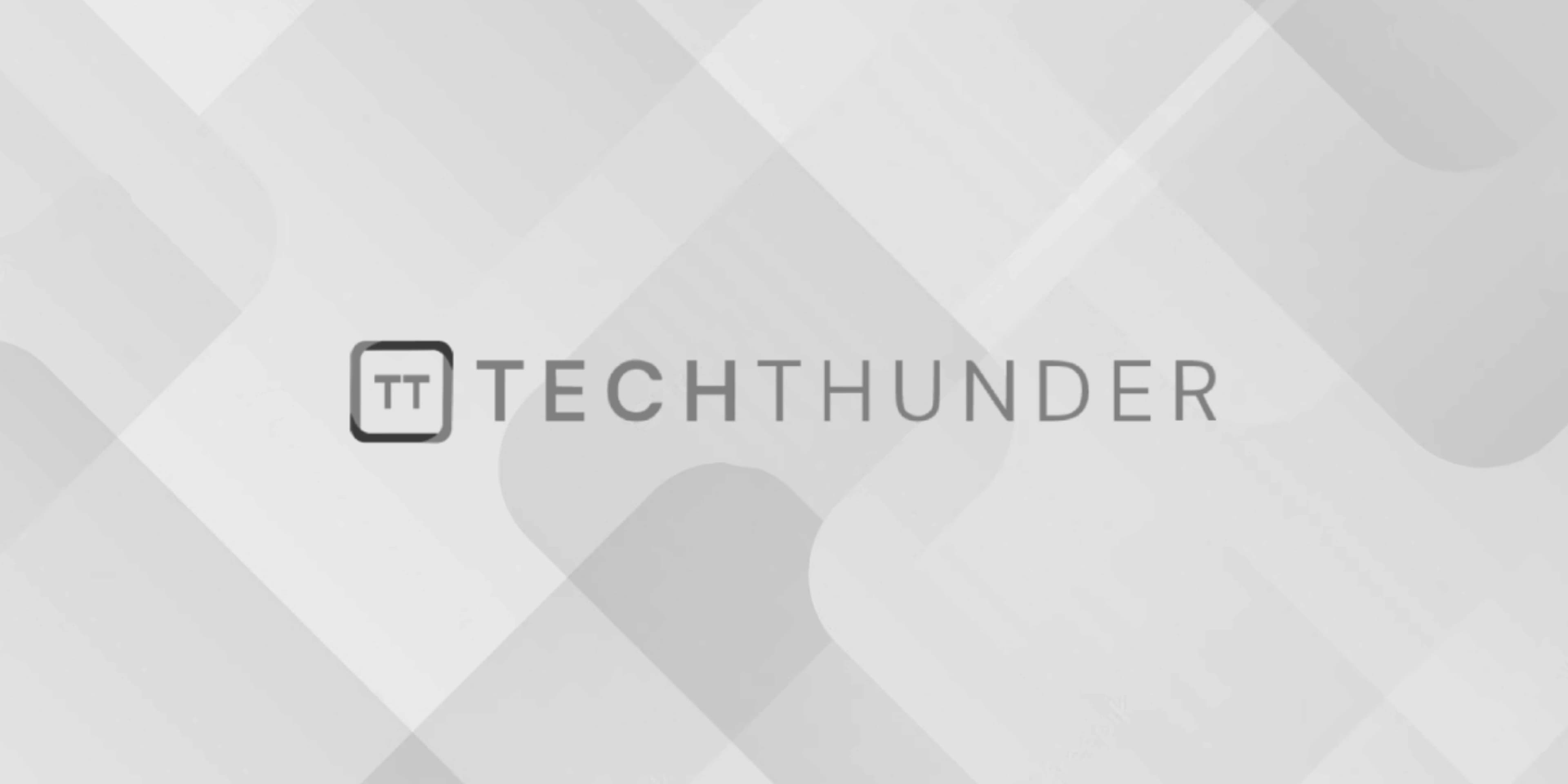
JavaScript Object
In JavaScript, an object is a collection of key-value pairs where each key is a unique identifier (also known as a property) that maps to a value. Objects are used to represent entities or concepts in code and allow you to store related data and functions together.
There are two main ways to create objects in JavaScript:
1. Object Literal Syntax:
const person = {
name: "John",
age: 25,
city: "New York",
sayHello: function() {
console.log("Hello!");
}
};
In this example, we create an object person
using object literal syntax. It has properties such as name
, age
, and city
, which are assigned values. It also has a method sayHello
that logs “Hello!” to the console.
2. Object Constructor Syntax:
function Person(name, age, city) {
this.name = name;
this.age = age;
this.city = city;
this.sayHello = function() {
console.log("Hello!");
};
}
const person = new Person("John", 25, "New York");
Here, we define a constructor function Person
that is used to create objects with the specified properties and methods. The this
keyword refers to the newly created object. We use the new
keyword to create an instance of the Person
object.
To access the properties and methods of an object, you can use dot notation or bracket notation:
console.log(person.name); // Output: "John"
console.log(person["age"]); // Output: 25
person.sayHello(); // Output: "Hello!"
You can also modify or add properties and methods to an object dynamically:
person.age = 30;
person["city"] = "San Francisco";
person.greet = function() {
console.log(`Greetings from ${this.city}!`);
};
person.greet(); // Output: "Greetings from San Francisco!"
Objects in JavaScript are versatile and can be used to model complex data structures and encapsulate functionality. They are a fundamental part of the JavaScript language and are extensively used in web development.