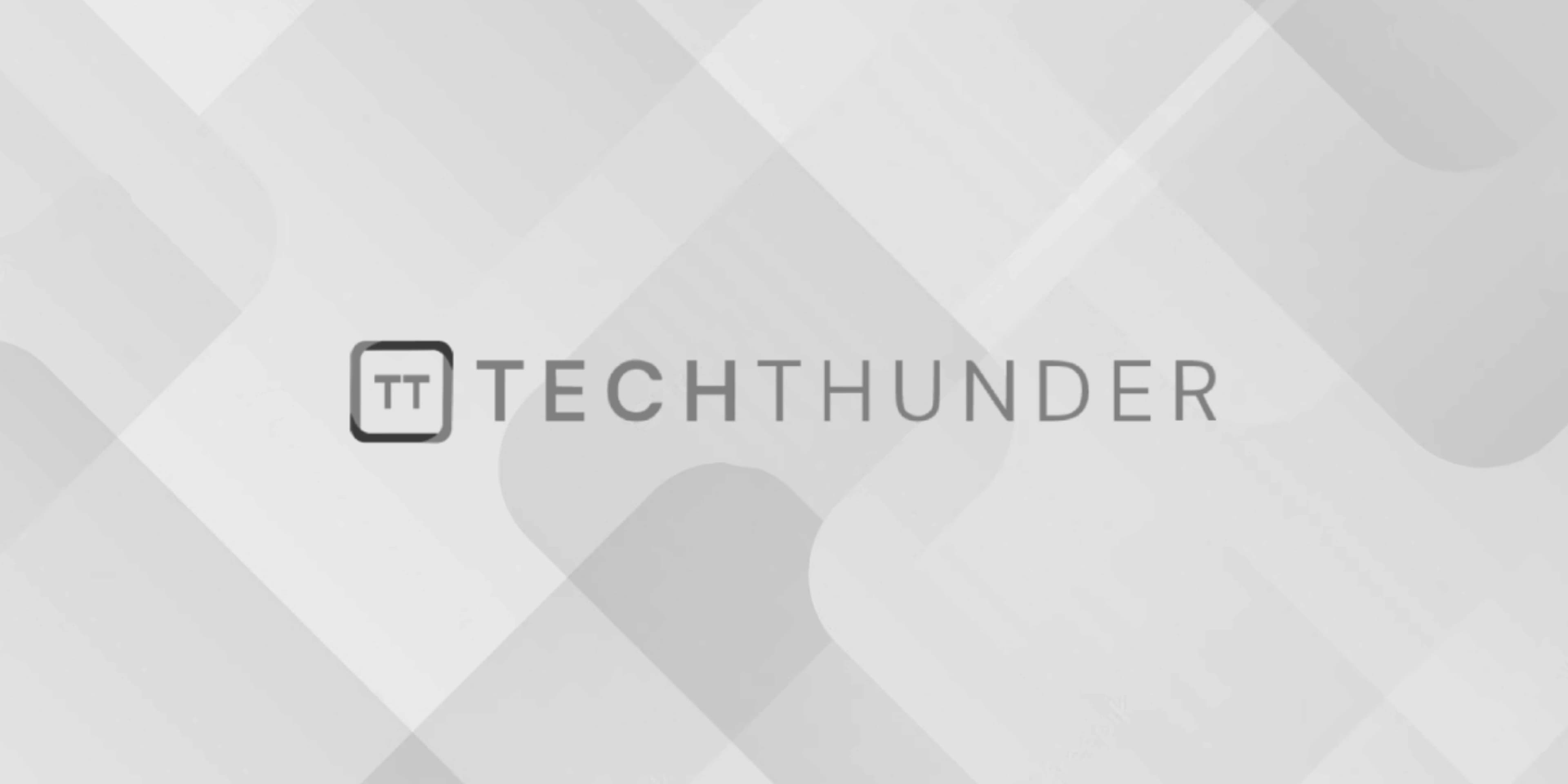
232 views
Calculate current week number in JavaScript
To calculate the current week number in JavaScript, you can use the Date
object and some simple calculations. Here’s an example:
JavaScript
function getCurrentWeekNumber() {
var now = new Date();
var yearStart = new Date(now.getFullYear(), 0, 1);
var weekNumber = Math.ceil(((now - yearStart) / 86400000 + yearStart.getDay() + 1) / 7);
return weekNumber;
}
// Example usage
var currentWeek = getCurrentWeekNumber();
console.log(currentWeek); // Output: Current week number
In this example, the getCurrentWeekNumber
function calculates the current week number based on the current date.
Here’s how the calculation works:
now
represents the current date and time.yearStart
is set to January 1st of the current year.- The difference in milliseconds between
now
andyearStart
is divided by the number of milliseconds in a day (86400000) to get the number of days. - The day of the week of
yearStart
(0 for Sunday, 1 for Monday, etc.) is added to the calculated number of days. - The sum is divided by 7 and rounded up using
Math.ceil()
to get the week number.
The resulting weekNumber
represents the current week number.
Note that the week numbering can vary depending on the country or region, so it’s important to consider the specific rules and conventions used in your target context.