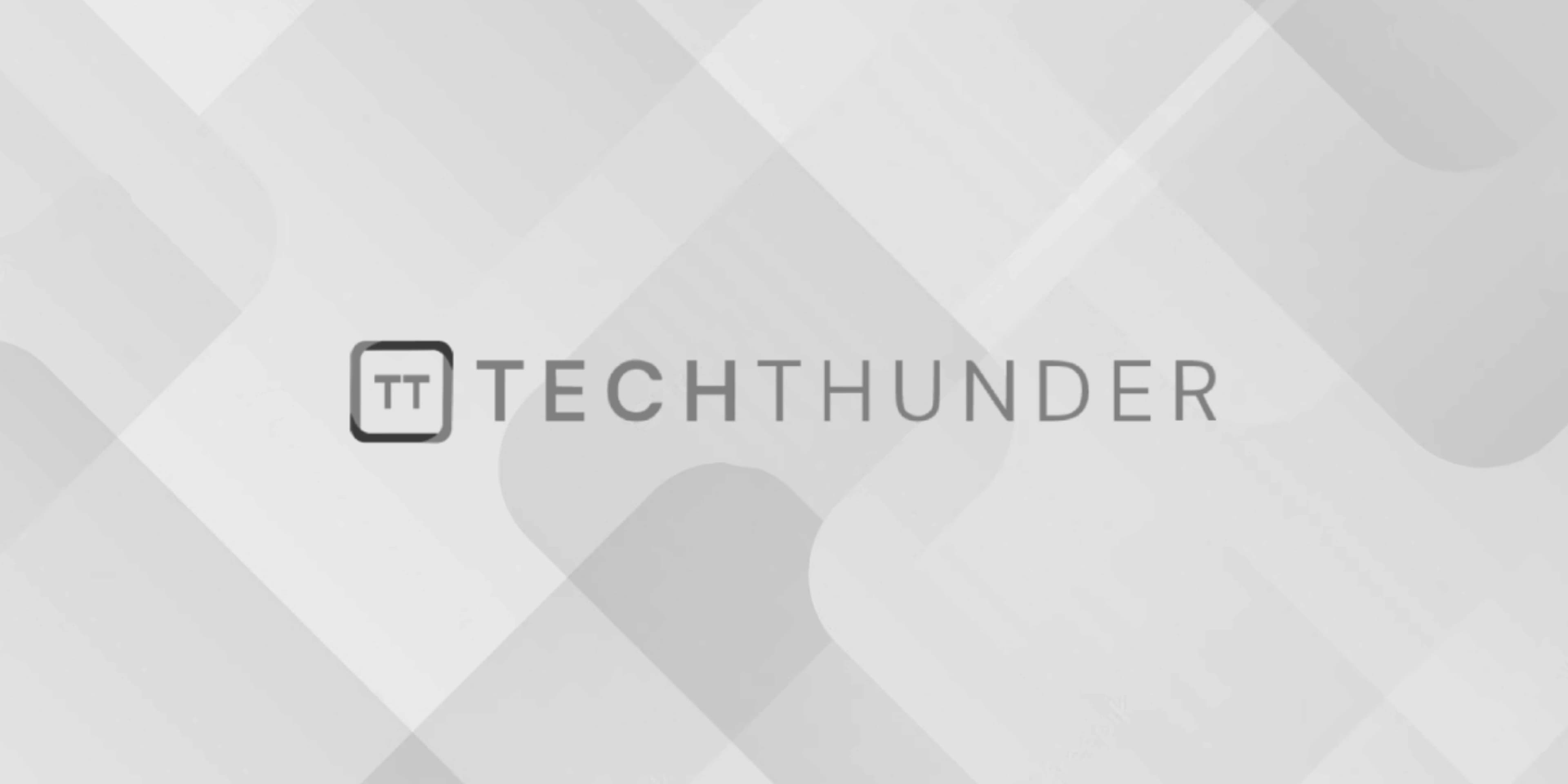
Traverse array object using JavaScript
To traverse an array of objects in JavaScript, you can use various methods such as a for loop, forEach, or map. Here are a few examples:
- Using a for loop:
var array = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 35 }
];
for (var i = 0; i < array.length; i++) {
console.log(array[i].name);
console.log(array[i].age);
}
In this example, the for loop iterates over each object in the array
. The array.length
property is used to determine the number of objects in the array. Inside the loop, you can access the properties of each object using dot notation (array[i].name
, array[i].age
).
- Using forEach:
var array = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 35 }
];
array.forEach(function(item) {
console.log(item.name);
console.log(item.age);
});
In this example, the forEach
method is called on the array
. It takes a callback function as an argument, which is executed for each item in the array. Inside the callback function, you can access the properties of each object using the item
parameter.
- Using map:
var array = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 35 }
];
var names = array.map(function(item) {
return item.name;
});
console.log(names);
In this example, the map
method is used to create a new array containing only the name
property of each object. The callback function provided to map
is executed for each item in the array. Inside the callback function, you can access the properties of each object using the item
parameter. The map
method returns a new array with the desired values.
These are just a few examples of how you can traverse an array of objects in JavaScript. The specific method you choose may depend on the desired outcome, such as performing operations on each object or extracting specific properties.