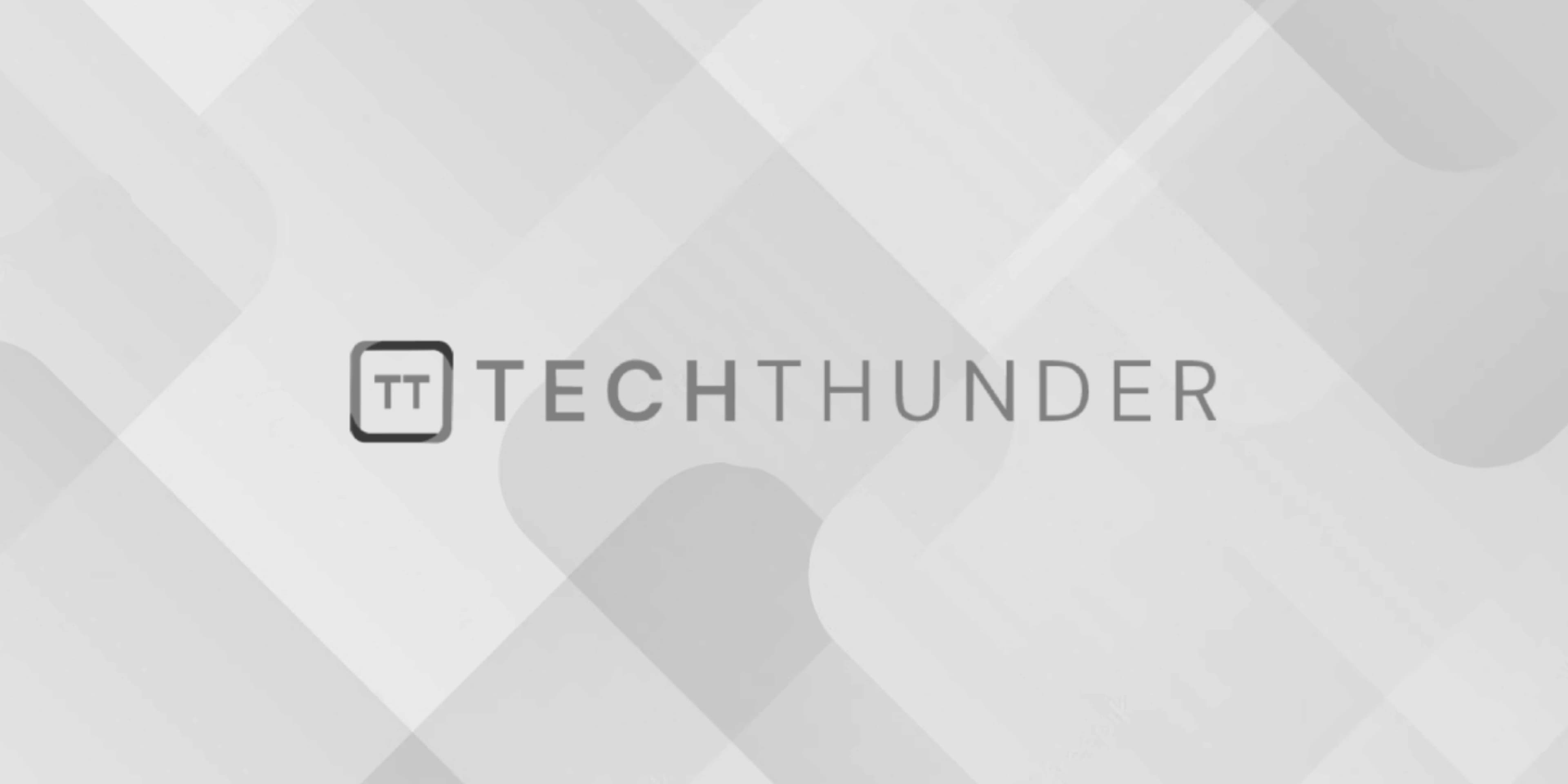
208 views
JavaScript function to check array is empty or not
To check whether an array is empty or not in JavaScript, you can use the length
property of the array. If the length is 0, it means the array is empty. Here’s a simple function that checks the emptiness of an array:
JavaScript
function isEmptyArray(arr) {
return arr.length === 0;
}
You can use this function by passing an array as an argument. It will return true
if the array is empty and false
otherwise.
Example usage:
JavaScript
var array1 = []; // Empty array
console.log(isEmptyArray(array1)); // Output: true
var array2 = [1, 2, 3]; // Non-empty array
console.log(isEmptyArray(array2)); // Output: false
In the above example, the isEmptyArray
function takes an array (arr
) as a parameter. It checks the length of the array using arr.length
and compares it to 0. If the length is 0, it returns true
, indicating an empty array. Otherwise, it returns false
.
You can customize or extend this function based on your specific requirements.